When it comes to object-oriented programming, constructors and destructors are two essential components of a class. Constructors are responsible for initializing the data members of an object, while destructors are responsible for freeing up the memory space occupied by the object when it is no longer needed. While overloading constructors is a common practice in C++, the same cannot be said for destructors.
Unlike constructors, a class can only have one destructor. This is because the destructor’s primary function is to destroy the object created by the constructor, and having multiple destructors would create conflicts and ambiguity. Therefore, it is not possible to define more than one destructor in a class.
It is important to note that a destructor takes no arguments and does not return any value. Its sole purpose is to release the resources held by the object and to perform any necessary cleanup operations. Once the destructor is called, the object is destroyed, and the memory it occupied is freed up.
While it is not possible to overload a destructor, there are certain circumstances in which you may need to perform specific actions before or after the object is destroyed. In such cases, you can use conditional statements wthin the destructor to perform those actions based on certain conditions.
For example, if an object holds a pointer to a resource that needs to be released before the object is destroyed, you can include a conditional statement in the destructor to release the resource if it exists. Similarly, if an object is part of a larger system and needs to perform certain cleanup operations before it is destroyed, you can include those operations in the destructor.
While it is not possible to overload a destructor in C++, you can still perform specific actions within the destructor to perform any necessary cleanup operations before or after the object is destroyed. By understanding the role of constructors and destructors in object-oriented programming, you can ensure that your code is efficient, maintainable, and error-free.
How Is Destructor Overloading Done?
Destructor overloading is not possble in C++. A class can have only one destructor, and its purpose is to free the memory allocated to the object. When an object goes out of scope or is explicitly deleted, the destructor is called automatically to perform the cleanup. The syntax for the destructor is similar to that of the constructor, but with a tilde (~) symbol before the class name.
For example:
“`
Class MyClass {
Public:
MyClass(); // Constructor
~MyClass(); // Destructor
};
MyClass::~MyClass() {
// Cleanup code here
}
“`
It is worth noting that if a derived class has a destructor, then the base class destructor is also called automatically after the derived class destructor finishes executing. This ensures that all resources are properly cleaned up in the correct order.
Destructor overloading is not possible in C++ as a class can have only one destructor.
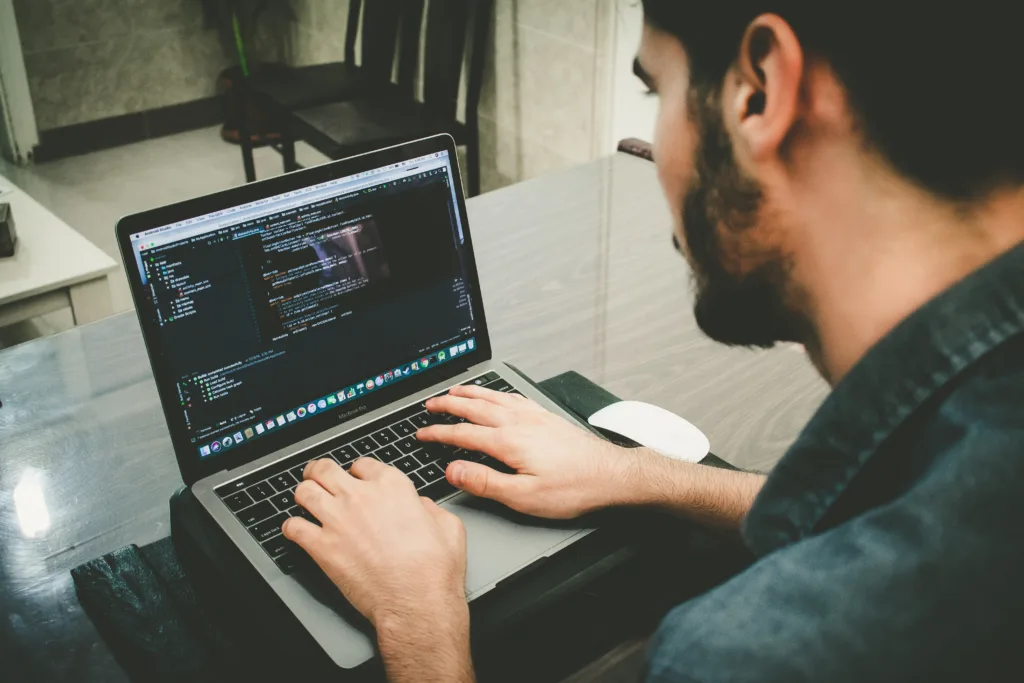
Why Can’t We Overload A Destructor?
The destructor is a special member function of a class that is responsible for releasing the resources allocated by the constructor. It is automatically called when the object goes out of scope or is explicitly destroyed using the delete operator.
However, it is not possible to define more than one destructor in a class. This is becaue the destructor serves a specific purpose of destroying the object created by the constructor, and having multiple destructors would only create ambiguity and confusion.
Furthermore, the destructor does not require any arguments and does not return any value, making it unnecessary to overload it. Overloading is only necessary for member functions that have different parameters or return types.
Overloading a destructor is not allowed because it serves a specific purpose, and having multiple destructors would only create ambiguity and confusion.
Can We Overload A Constructor And Destructor?
In C++ programming, we can overload constructors but we cannot overload destructors. Overloading a constructor means that we can have multiple constructors with dfferent parameters in the same class. This allows us to create objects of the class with different initializations. For example, we can have a constructor that initializes all the member variables to zero and another constructor that initializes them to some specific values.
However, we cannot overload a destructor. In fact, we should only have one destructor per class, which is responsible for freeing the memory allocated by the object. Having multiple destructors with different parameters would not make sense, as the destructor is called automatically when the object goes out of scope or is explicitly deleted.
To summarize, we can overload constructors in C++ but not destructors. A class should have only one destructor, which is responsible for freeing the memory allocated by the object.
Can We Overload Destructor In Swift?
In Swift, it is not possible to overload a destructor. A destructor, also known as a deinitializer in Swift, is a special method that is automatically called when an object is destroyed. It takes no arguments and canot be called explicitly. Since it is a special method with a specific signature, overloading it would not make sense. If you need to perform different actions depending on certain circumstances when destroying an object, you can use conditional statements within the deinitializer to achieve this. However, it is not possible to have multiple deinitializers with different signatures for the same class or struct.
Conclusion
It is not possible to overload a destructor in C++. The reason being that a class can have only one destructor, which is used to destroy the object created by the constructor. Moreover, a destructor takes no arguments and does not return any value. Therefore, there is no point in trying to overload it. If you need to perform different actions depending on crtain circumstances while destroying an object, you can use appropriate if statements in your destructor to check for those circumstances.