When working with maps, it is important to understand that duplicate keys are not allowed. However, in some cases, it may be necessary to store duplicate keys in a map. In such cases, a multi-map can be used to store these elements.
A multi-map is similar to a regular map, with the addition that multiple elements can have the same keys. It is not required that the key-value and mapped value pair have to be unique in this case. This makes multi-maps ideal for storing elements with duplicate keys.
To store duplicate keys in a map, one can use the Guava library. Guava is an open-source Java library that provides a variety of usful utilities and data structures for Java programmers. The Guava library includes a MultiMap interface that can be used to store elements with duplicate keys.
To use the MultiMap interface in Guava, one can create an instance of the MultiMap interface using the following code:
“`
MultiMap multiMap = ArrayListMultimap.create();
“`
In this example, the MultiMap interface is parameterized with a key type of String and a value type of Integer. The ArrayListMultimap.create() method is used to create a new instance of the MultiMap interface.
Once the MultiMap interface has been created, elements can be added to it using the put() method. For example, to add an element to the multi-map, one can use the following code:
“`
MultiMap.put(“key”, 1);
MultiMap.put(“key”, 2);
MultiMap.put(“key”, 3);
“`
In this example, we are adding three elements with the key “key” and values 1, 2, and 3 respectively. Since we are using a multi-map, all three elements will be stored in the map even though they have the same key.
To retrieve elements from the multi-map, one can use the get() method. For example, to retrieve all the elements with the key “key”, one can use the following code:
“`
Collection values = multiMap.get(“key”);
“`
In this example, the get() method is used to retrieve all the values associated with the key “key”. The values are returned as a Collection of integers.
When working with maps, it is important to understand that duplicate keys are not allowed. However, in some cases, it may be necessary to store elements with duplicate keys. In such cases, a multi-map can be used to store these elements. The Guava library provides a MultiMap interface that can be used to store elements with duplicate keys. By using a multi-map, one can store elements with duplicate keys and retrieve them as needed.
Storing Duplicate Keys in a Map
The concept of Map in Java is to store key-value pairs where each key is unique. This means that if you try to add a key that already exists in the Map, it will overwrite the previous value associated with that key. However, there are certain scenarios where you may want to store duplicate keys in a Map. In such cases, you can use one of the following approaches:
1. Use a List as the value for each key – You can create a Map where the value for each key is a List of values. Whenever you want to add a new value for a key, you can simply add it to the List associated with that key.
2. Use a Set as the value for each key – Similar to the first approach, you can create a Map where the value for each key is a Set of values. Whenever you want to add a new value for a key, you can simply add it to the Set associated with that key. This approach ensures that each value is unique for a given key.
Here’s an example implementation of the first approach:
“`java
Map map = new HashMap();
String key = “example”;
If (map.containsKey(key)) {
List list = map.get(key);
List.add(1); // add new value to existing list
Map.put(key, list); // update map with updated list
} else {
List list = new ArrayList();
List.add(1); // add new value to new list
Map.put(key, list); // add new key-value pair to map
}
“`
And here’s an example implementation of the second approach:
“`java
Map map = new HashMap();
String key = “example”;
If (map.containsKey(key)) {
Set set = map.get(key);
Set.add(1); // add new value to existing set
Map.put(key, set); // update map with updated set
} else {
Set set = new HashSet();
Set.add(1); // add new value to new set
Map.put(key, set); // add new key-value pair to map
}
“`
It’s important to note that while these approaches allow you to store duplicate keys in a Map, you need to be careful when retrieving values from the map. You’ll need to loop through the List or Set to get all the values associated with a given key. Additionally, you may want to consider using a differnt data structure altogether if you need to store a large number of duplicate keys.
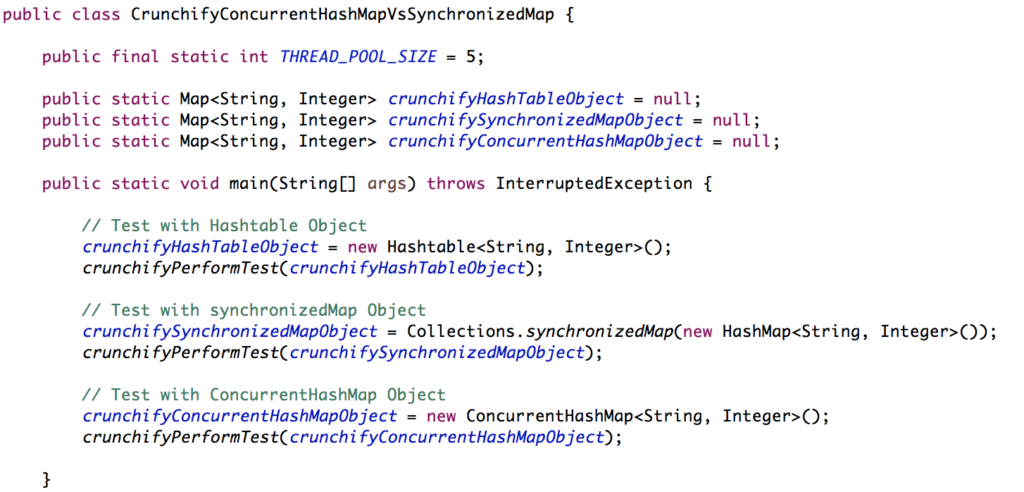
Can Duplicate Keys Be Used in a HashMap?
HashMap is a widely used data structure in Java that stores data in key-value pairs. One of the fundamental characteristics of HashMap is that it does not allow duplicate keys. This means that any attempt to insert a key that already exists in the HashMap will replace the existing value asociated with that key. In other words, the HashMap will only contain one entry per unique key.
However, it is important to note that HashMap does allow duplicate values. This means that multiple keys can have the same value associated with them. It is also worth noting that HashMap allows for a null key, but only one null key can be present in the HashMap.
To summarize, HashMap does not allow duplicate keys, but it does allow duplicate values and a single null key. This is an important aspect to keep in mind when working with HashMaps, as it can impact the behavior of your code.
Storing Duplicate Keys in a Map in CPP
C++ is a popular programming language that is widely used to develop efficient and reliable software applications. One of the most commonly used data structures in C++ is the map container, which allows you to store key-value pairs. However, a map in C++ does not alow you to have duplicate keys. This means that if you try to insert a key that already exists in the map, the new value will overwrite the existing value.
To store duplicate keys in a map in C++, you can use a multi-map. A multi-map is a container that is similar to a map, but it allows you to store multiple values for the same key. This means that each key in a multi-map can have multiple values associated with it.
To use a multi-map in C++, you need to include the header file in your code. Once you have included the header file, you can create a multi-map object using the following syntax:
“`cpp
Std::multimap my_multimap;
“`
In this syntax, `key_type` is the data type of the key that you want to use, and `value_type` is the data type of the value that you want to associate with the key.
To insert a new key-value pair into the multi-map, you can use the `insert()` function, like this:
“`cpp
My_multimap.insert(std::make_pair(key, value));
“`
This will insert a new key-value pair into the multi-map. If the key already exists in the multi-map, the new value will be added to the existing values for that key.
To iterate over the elements in the multi-map, you can use a range-based for loop, like this:
“`cpp
For (const auto& pair : my_multimap) {
// Do something with pair.first (the key)
// and pair.second (the value)
}
“`
Alternatively, you can use the `find()` function to search for elements with a specific key, like this:
“`cpp
Auto range = my_multimap.equal_range(key);
For (auto it = range.first; it != range.second; ++it) {
// Do something with it->second (the value)
}
“`
If you need to store duplicate keys in a map in C++, you can use a multi-map. A multi-map is a container that allows you to store multiple values for the same key. You can create a multi-map using the std::multimap class and insert new key-value pairs using the `insert()` function. To iterate over the elements in the multi-map, you can use a range-based for loop or the `find()` function.
Duplicate Keys in Map C++
In C++, a map is a container that stores key-value pairs where the keys are unique. This means that there cannot be duplicate keys in a map. If a duplicate key is inserted into the map, the previous value associated with that key will be overwritten by the new value.
However, if you need to store multiple values for the same key, you can use a data structure called a multimap. A multimap is similar to a map, but it allos for multiple values to be associated with the same key. In other words, a multimap can have duplicate keys.
Here’s an example of how to use a multimap in C++:
“`
#include
#include
Int main() {
Std::multimap myMap;
// Insert multiple values for the same key
MyMap.insert(std::make_pair(1, “apple”));
MyMap.insert(std::make_pair(1, “banana”));
MyMap.insert(std::make_pair(2, “orange”));
// Iterate over the multimap and print all values
For (auto it = myMap.begin(); it != myMap.end(); ++it) {
Std::cout
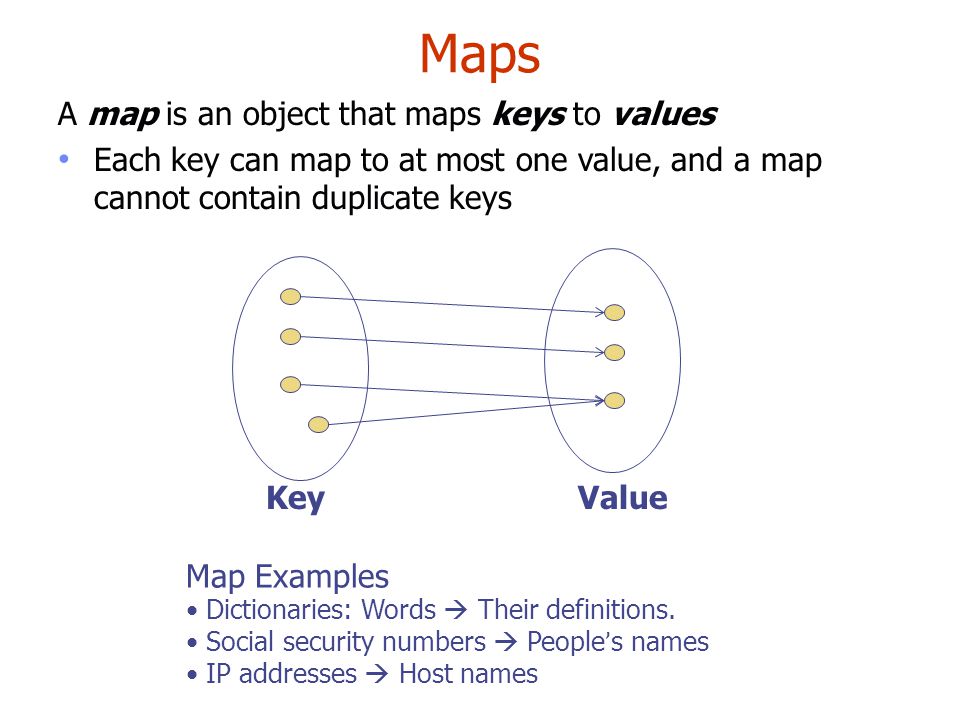
Conclusion
When it comes to storing duplicate keys in a Map, it is important to consider the implementation classes available. While HashMap allows for duplicate values, it does not allow for duplicate keys. On the other hand, TreeMap maintains an order of objects but also does not allow for duplicate keys.
However, the solution to storing duplicate keys lies in usng a MultiMap. This data structure allows for multiple elements to have the same keys, which is not possible in a regular Map. This makes it a perfect solution when dealing with situations where duplicate keys are present.
By using a MultiMap, we can efficiently store and access data with duplicate keys without having to resort to complicated workarounds. It is a simple and effective solution that makes managing data with duplicate keys much easier. it is important to choose the appropriate data structure for the task at hand to ensure efficient and organized data management.