Java is an incredibly powerful and versatile language, but one of its few drawbacks is the fact that it doesn’t have built-in support for constants. What exactly are constants? Constants are values that remain unchanged throughout the course of a program, making them incredibly useul when working with complex data structures or algorithms. Unfortunately, Java does not provide any way to declare constant values, which can make it difficult to keep track of important values in your code.
However, there are still ways to work around this limitation and declare constants in your Java code. The most common method is to use the keyword “final” before declaring a variable. This tells the compiler that the value associated with the variable will never change and should be treated as a constant. Additionally, it’s a good practice to write constants in capital letters, so they’re easily recognizable from other variables.
Another type of constant you can use in Java is real constants (also known as floating-point constants). Real constants consist of a sequence of digits with fractional parts or decimal points, such as 2.3, 0.0034, -0.75 and 56.7 etc., and they’re often used when dealing with more complex mathematical operations that involve lots of numbers with decimal points.
By using these two types of constants, you can effectively create immutable values in your Java code without introducing any additional complexity into your programs. This makes it easier to keep track of important values throughout the course of your program and ensures that these values are not accidentally changed by other parts of your codebase at any point during execution.
Overall, while Java does not have built-in support for constants like some other languages do, there are still ways to effectively create them using the language’s existing features and practices. By following best practices such as using the “final” keyword and writing real or floating-point constants appropriately, you can ensure that important values remain unchanged throughout your program’s execution for greater reliability and accuracy!
Does Java Include Constants?
No, Java does not have built-in support for constants. However, developers can use the keyword “final” before a variable declaration to indicate that the value of the variable cannot be changed in the future. This can help improve readability and performance of a program, as well as help ensure that a given value is not unintentionally changed within code.
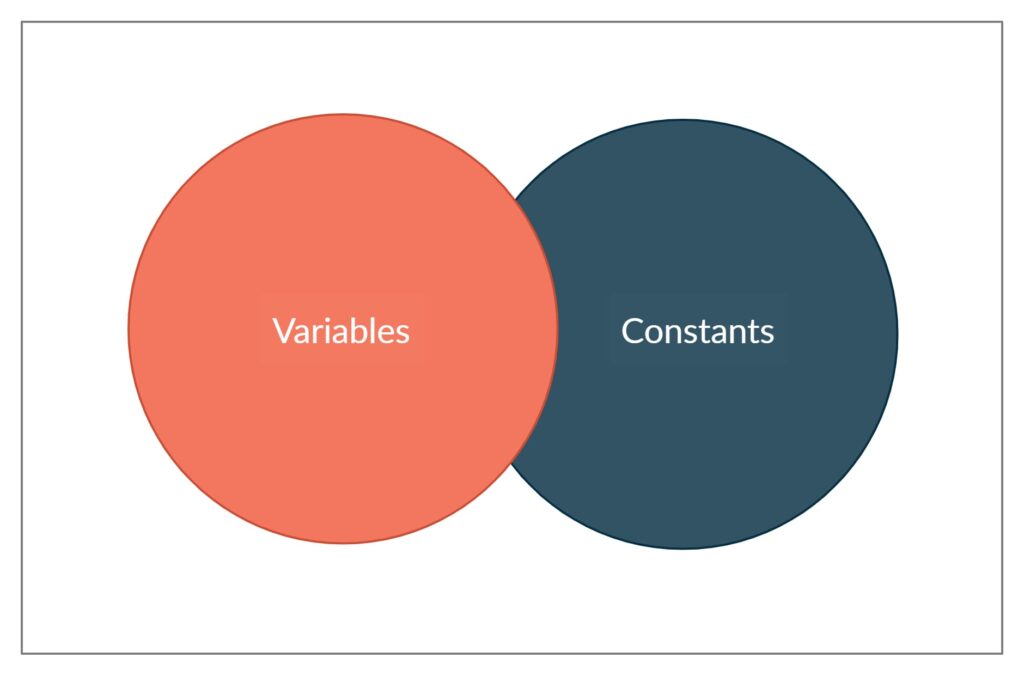
Why Java Does Not Use Const
Java was designed to be a language that is easy to learn and use. The designers wanted to avoid any confusion that might arise from implementing the keyword ‘const’. Instead, they chose to provide other language features which allow developers to declare constants or provide immutability. This allows for a more straightforward approach for developers as they do not need to worry about the specific syntax of ‘const’ when declaring a constant or making something immutable. Furthermore, by avoiding ‘const’, the language remains simpler and easier to use.
Declaring a Const in Java
To declare a constant in Java, you need to use the keyword “final” before the data type of the variable. For example, if you wanted to declare a constant integer called MAX_VALUE, you would write:
final int MAX_VALUE = 10;
The “final” keyword indicates that this variable cannot be changed once it has been assigned a value. It is also good practice to write constants in capital letters, as this helps differentiate them from ordinary variables. If you try to change the value of a constant in your program, javac (the Java Compiler) will generate an error message.
Examples of Constants in Java
In Java, constants are values that don’t change and remain the same troughout the execution of a program. Constants can be classified into two types: numerical constants and character constants.
Numerical constants can be further divided into integer constants, real constants, and boolean constants. Integer constants are whole numbers without a fractional part or decimal point, such as 5, -7, 0 etc. Real constants consist of a sequence of digits with fractional parts or decimal points, such as 2.3, 0.0034, -0.75 etc. Boolean constants can be either true or false.
Character constants are single characters enclosed in single quotes ‘a’, ‘b’, ‘c’ etc or string literals enclosed in double quotes “Hello World”.
Uses of the ‘const’ Keyword
Const is used to create an immutable value, meaning that the value cannot be changed during the course of a program’s execution. This can be useful in situations where a value needs to remain constant and unchanging, such as when defining a mathematical constant or when setting up a loop that has to repeat the same action with no change. Const also helps with code readability and maintainability, as it clearly indicates which values are meant to be immutable.
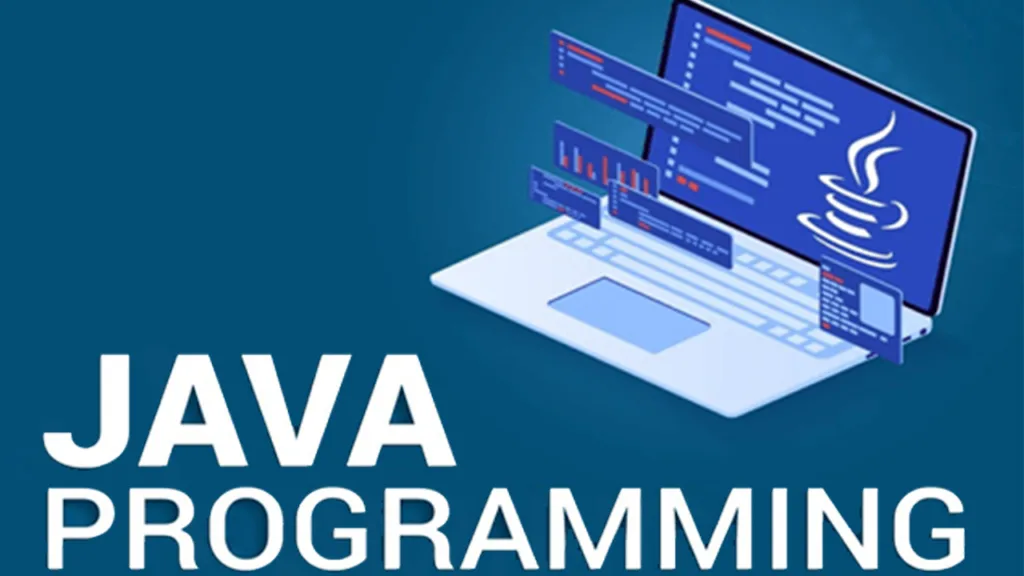
Types of Variables: Is Const a Variable Type?
No, const is not a variable type. It is an attribute that can be applied to a variable declaration. A const variable must be initialized when it is declared and its value cannot be changed during the lifetime of the program. This attribute causes the compiler to generate an error if you try to modify the value of a const variable.
Comparing the Benefits of Const and Let
Const is generally preferred over let when declaring variables, because it helps to ensure that the value of the variable will not change unexpectedly. This can help reduce bugs and make code easier to read and understand. However, if you know that a variable’s value will be changing often, then it is best to use let instead of const. Const is better than let in terms of keeping your code clean and organized, but when it comes to flexibility and changing values, let may be a better choice.
Alternative to Using ‘const’ in Java
In Java, when you want to declare a constant variable, you can use the keyword ‘final’ instead of ‘const’. This keyword is used to indicate that the value of this variable cannot be changed. For instance, if you wanted to assign a fixed number to a variable, you could do so by declaring it as ‘final int number = 10;’. The same applies for other data types such as Strings and Booleans.
Comparing Const and Macro
It really depends on the situation. Const statements are allocated in read-only memory, meaning that their value cannot be changed by the executing program. This can be very useful when you need to guarantee a cetain value stays the same throughout your code. However, macros are more efficient than const statements since they don’t require any memory allocation and are faster to execute. They also tend to make code more readable, as they can be used to define shorthand versions of longer commands. Ultimately, it’s up to you as a programmer to decide which one is better for the task at hand.
What is a Const Statement?
A const statement is a type of declaration used to create a block-scoped constant, which is similar to a variable declared using the let keyword. The value of a constant cannot be changed through reassignment (e.g. with the assignment operator) or redeclared (e.g. with a variable declaration). Constants must be initialized when declared, and their values cannot be changed afterwards. This makes them useful in situations where you need to ensure that the value of a variable stays the same throughout your code.
The Use of Const in Classes
We use the const keyword in classes for severl reasons. Firstly, it helps to protect our data and prevent accidental changes to our objects. It also helps to ensure that our code is more readable and maintainable, as it allows us to clearly indicate which functions are meant to be used to modify our objects and which are not. Additionally, const member functions can be called by any type of object, thus making them more flexible. Finally, using const can also help improve performance, as calling a const member function may enable the compiler to perform optimizations when generating the executable code.
Types of Constants
The three main types of constants are integer, string, and hexadecimal string. An integer constant is a type of data that consists of any whole number, such as -5 or 45. A string constant is any sequence of characters surrounded by quotation marks, such as “Hello World!” Lastly, a hexadecimal string constant is a type of data consisting of any combination of the 16 digits (0-9, A-F) that represents a hexadecimal number. For example, 0xFF would be a valid hexadecimal string constant.
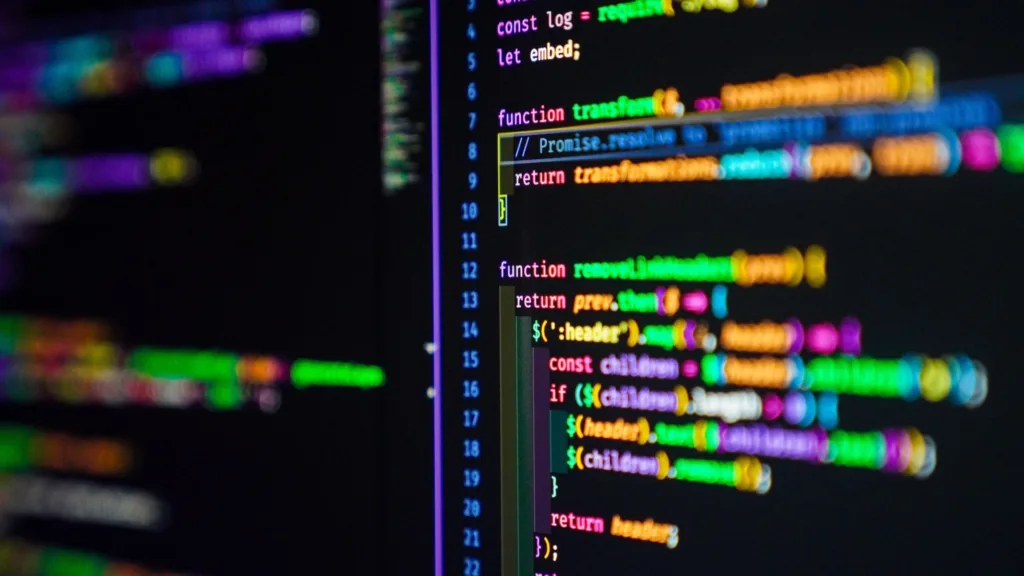
Conclusion
In conclusion, Java does not have built-in support for constants, but they can still be declared and used in the program. To declare a constant in Java, simply use the keyword “final” before the variable name. Constants should be written in all capital letters to differentiate them from ordinary variables. Real constants are also called floating-point constants and consist of a sequence of digits with fractional parts or decimal points. Although there is no ‘const’ keyword in Java, developers can still use constants to make their programs more easily readable and efficient.