In the world of coding, parameters play a significant role in the functionality and versatility of functions. Parameters are essentially variables that are used to pass data from the caller into a function. By utilizing parameters, programmers can create functions that can perform tasks without knowing the specific input values ahead of time.
To understand the concept of parameters, let’s delve into the basics of functions in coding. A function is a self-contained block of code that performs a specific task. It takes input, processes it, and returns an output. Functions are essential for breaking down complex programs into smaller, manageable pieces.
When defining a function, parameters are used to specify what kind of input the function expects. These parameters act as placeholders for the actual values that will be passed into the function when it is called. They allow the function to be flexible and adaptable to different situations.
Parameters are enclosed within parentheses after the function’s name. Multiple parameters can be included, separated by commas. Each parameter is assigned a name, which is used within the function to refer to the corresponding input value.
Once a function is defined with parameters, it can be called by providing actual values for those parameters. These values are known as arguments. When the function is called, the arguments are passed into the function and assigned to the corresponding parameters. The function then executes its code using these values.
Parameters can have different data types, such as integers, strings, or booleans. They can also have default values assigned to them. Default values are used when the caller does not provide a specific argument for that parameter. They ensure that the function can still be executed even if some arguments are missing.
One of the key benefits of using parameters is code reusability. By defining functions with parameters, programmers can create modular and reusable code. Functions can be called multiple times with different arguments, allowing them to perform the same task on different sets of data.
Parameters also enhance the flexibility and scalability of functions. They enable functions to handle a wide range of inputs, making them adaptable to various scenarios. By simply changing the arguments passed into the function, the behavior and output can be altered without modifying the function itself.
Parameters are vital components of functions in coding. They allow functions to receive input values from the caller, making the functions versatile and adaptable. By using parameters effectively, programmers can create reusable and flexible code that can handle a wide range of inputs. So next time you write code, make sure to harness the power of parameters to enhance the functionality and efficiency of your functions.
What Is Parameter With Example?
A parameter, in the context of statistics and research, refers to a characteristic or measure that describes an entire population being studied. It is a value that summarizes a particular aspect of the population of interest. Parameters are often used to make inferences or draw conclusions about the population based on a sample of data.
To provide a clearer understanding, let’s consider an example. Imagine a group of researchers is interested in studying the average height of all adults in a specific country. In this case, the parameter would be the average height of the entire adult population in that country. This parameter would represent the true average height if it were possible to measure every adult in the country. However, this is often not feasible due to time, cost, and logistical constraints.
To estimate the parameter, the researchers would take a sample of adults from the population and measure their heights. The sample mean, which is the average height of the individuals in the sample, would serve as an estimate of the population parameter.
Parameters are used to describe characteristics of an entire population and are often estimated using sample data. They provide valuable insights into the population being studied and help researchers make generalizations and draw conclusions about the larger group.
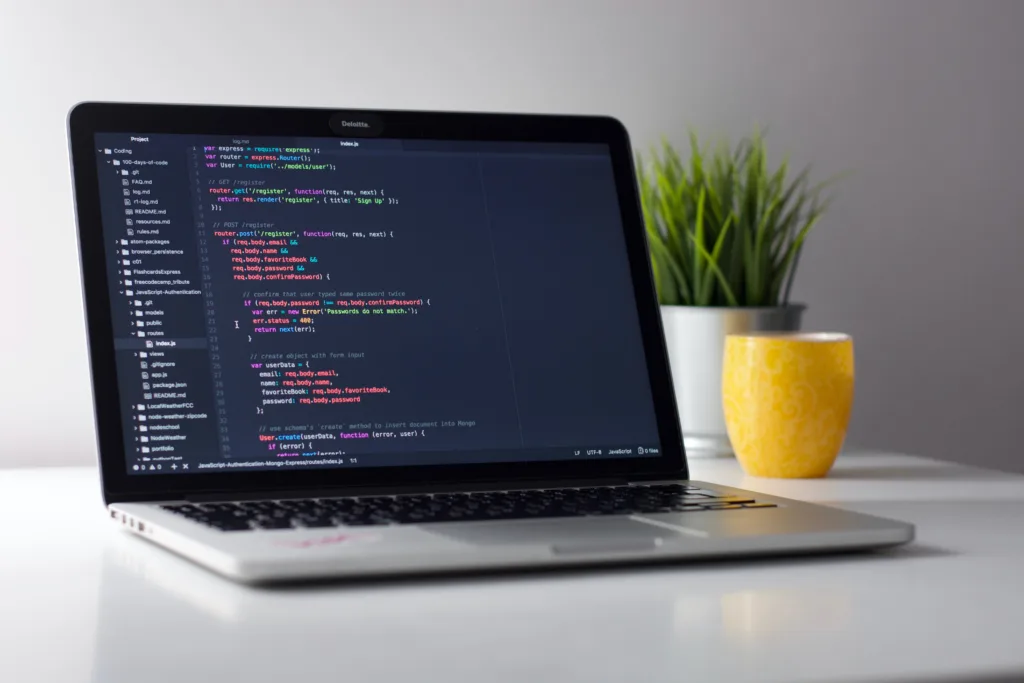
What Is A Parameter In Simple Terms?
A parameter, in simple terms, refers to an arbitrary constant or quantity that helps describe or characterize a particular system or population. It is commonly used in fields such as mathematics, statistics, and science. Parameters can take on various forms depending on the context in which they are used. For instance, in mathematics, a parameter can be an unknown constant that represents a family of curves or equations. In statistics, parameters can represent descriptive measures like means or variances that provide information about a population.
To provide a clearer understanding, here are a few examples of parameters in different contexts:
1. In mathematics, consider the equation of a straight line: y = mx + c. Here, ‘m’ and ‘c’ are parameters that determine the slope and y-intercept of the line, respectively. By varying the values of ‘m’ and ‘c,’ you can obtain different lines within the family of equations.
2. In statistics, let’s say you want to describe the average height of a population. You can collect data from a sample of individuals and calculate their heights. The mean height of the sample would then be an estimate of the population parameter, which represents the average height of everyone in the population.
3. In computer programming, parameters are often used in functions or methods to pass values between different parts of a program. These parameters define the input and output requirements for a particular function, allowing it to perform specific tasks.
A parameter is an arbitrary constant or quantity that helps define or describe a system or population. It can take different forms depending on the field of study, but its purpose remains the same – to provide valuable information about a specific context.
Why Are Parameters Used In Code?
Parameters are used in code to enhance the flexibility and reusability of functions. They allow a function to perform tasks without knowing the specific input values ahead of time. By accepting parameters, a function can be written in a generic way, making it applicable to a wide range of scenarios.
There are several key reasons why parameters are used in code:
1. Flexibility: Parameters enable functions to handle different inputs and produce different outputs based on those inputs. By passing different values as parameters, the same function can be used to perform various operations, adapting to different situations.
2. Reusability: Functions with parameters can be reused in multiple parts of a program or even in different programs. Instead of writing multiple functions to perform similar tasks with slight variations, a single function with parameters can be created and reused wherever needed, thereby reducing code duplication.
3. Modularity: Parameters allow programmers to break down their code into logical blocks, making it easier to understand, debug, and maintain. By passing parameters to functions, the code can be organized into smaller, self-contained units, each responsible for a specific task.
4. Customization: Parameters make it possible to customize the behavior of a function based on specific requirements. By altering the values passed as parameters, the function’s output can be tailored to meet different needs, providing a high level of flexibility and adaptability.
5. Interoperability: Parameters enable functions to interact with each other by sharing information. Functions can pass data to one another through parameters, allowing them to collaborate and achieve more complex tasks collectively.
Parameters are an essential component of functions in code as they enhance flexibility, reusability, modularity, customization, and interoperability. They enable functions to handle different inputs, produce different outputs, and be used in various contexts, resulting in more efficient and maintainable code.
What Does A Parameter Look Like In Code?
A parameter in code is a variable or storage place that is used to pass data from the caller of a function into the function itself. It is defined within the function’s parentheses, separated by commas if there are multiple parameters.
Parameters are like containers that hold the data that is passed into the function. They act as placeholders for the values that the function needs to perform its tasks. When a function is called, the actual values that are passed into the function are called arguments, and they are assigned to the corresponding parameters.
Here’s an example to illustrate how parameters are used in code:
“`python
Def calculate_sum(num1, num2):
Result = num1 + num2
Return result
Sum = calculate_sum(5, 10)
Print(sum)
“`
In this code snippet, the function `calculate_sum` takes two parameters: `num1` and `num2`. When the function is called with the values `5` and `10`, these values are assigned to the parameters `num1` and `num2`. The function then calculates the sum of the two numbers and returns the result.
Parameters can have different data types, such as integers, strings, or even other objects. They can also have default values, which means that if no value is provided when calling the function, the default value will be used.
Using parameters allows for more flexibility and reusability in code. By passing different values as arguments, the same function can perform different tasks based on the provided data. It also enables functions to interact with data from outside their scope, making the code more modular and organized.
A parameter in code is a variable or storage place that holds the data passed into a function. It is defined within the function’s parentheses and allows for the flexibility and reusability of functions.
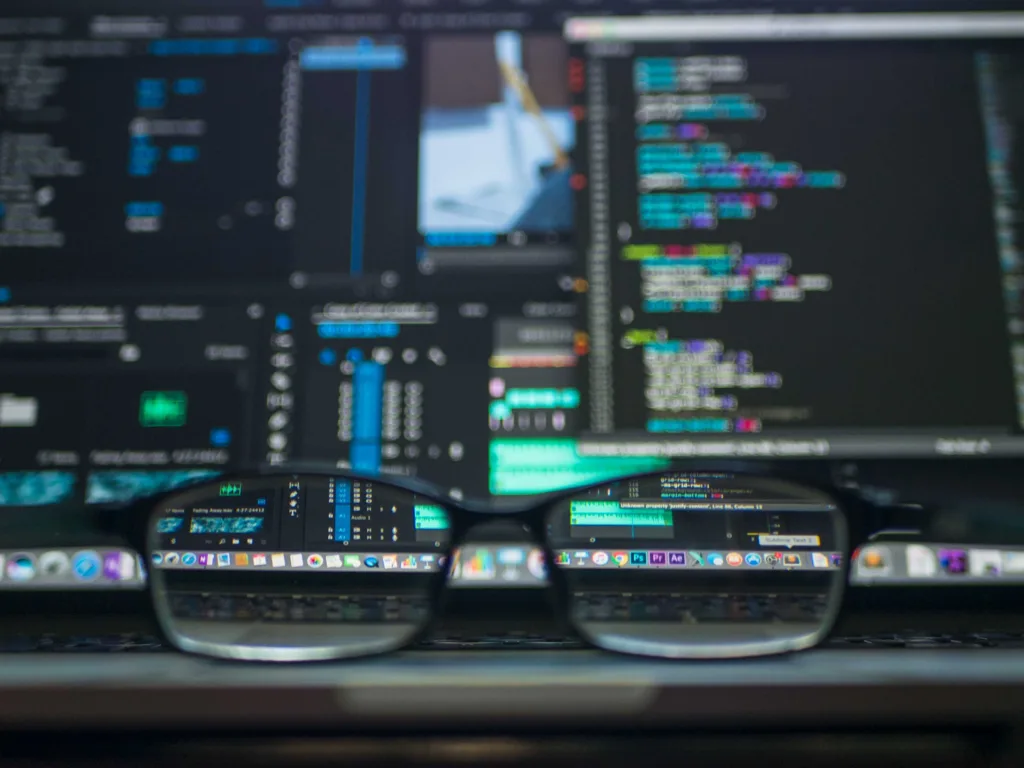
Conclusion
A parameter is a crucial component in programming that allows functions to perform tasks efficiently without requiring specific input values. It serves as an arbitrary constant or quantity that characterizes a member of a system or describes a statistical population. Parameters act as storage places or variables within a function, enabling the passing of data from the caller into the function. They play a vital role in dividing code into logical blocks, enhancing code reusability, and improving the overall organization and efficiency of programs. By understanding and effectively utilizing parameters, programmers can create more flexible and adaptable functions that can cater to a wide range of scenarios and inputs.