When it comes to building composite types in Java, there are two options: classes and interfaces. Unlike other programming languages, such as C or C++, Java does not support the use of structs or unions. However, the concept of a struct can be achieved through the use of classes and interfaces.
A struct is a composite type that allows you to group related variables into one place. Each variable in the struct is known as a member. Unlike arrays, structs can hold many different data types, including int, float, char, and more. With structs, you can represent real-world entities with their respective properties.
In Java, you can use classes to create structs. Here is an example:
“`
Public class Car {
String model;
String make;
Int year;
Float price;
}
“`
In this example, a Car class has been created, with four members: model, make, year, and price. Each member has its own data type, and together they represent a car.
To use the Car struct, you can create an instance of the class and set its values:
“`
Car myCar = new Car();
MyCar.model = “Toyota”;
MyCar.make = “Camry”;
MyCar.year = 2021;
MyCar.price = 25000.50f;
“`
Now, you can access the members of the Car struct using the dot notation:
“`
System.out.println(“Model: ” + myCar.model);
System.out.println(“Make: ” + myCar.make);
System.out.println(“Year: ” + myCar.year);
System.out.println(“Price: ” + myCar.price);
“`
This will output:
“`
Model: Toyota
Make: Camry
Year: 2021
Price: 25000.5
“`
Another way to create structs in Java is by using interfaces. Interfaces are similar to classes, but they only cntain method signatures and constants. Here is an example:
“`
Public interface Person {
String getName();
Int getAge();
Void setName(String name);
Void setAge(int age);
}
“`
In this example, a Person interface has been created, with four method signatures: getName, getAge, setName, and setAge. This interface can be used to create a struct for a person:
“`
Public class Employee implements Person {
Private String name;
Private int age;
Public String getName() {
Return name;
}
Public int getAge() {
Return age;
}
Public void setName(String name) {
This.name = name;
}
Public void setAge(int age) {
This.age = age;
}
}
“`
In this example, the Employee class implements the Person interface. It has two members, name and age, and it implements the four methods from the Person interface. Now, you can create an instance of the Employee struct and set its values:
“`
Employee myEmployee = new Employee();
MyEmployee.setName(“John Doe”);
MyEmployee.setAge(30);
“`
And you can access the members of the Employee struct using the methods from the Person interface:
“`
System.out.println(“Name: ” + myEmployee.getName());
System.out.println(“Age: ” + myEmployee.getAge());
“`
This will output:
“`
Name: John Doe
Age: 30
“`
Structs can be created in Java using classes and interfaces. They allow you to group related variables into one place, and they can represent real-world entities with their respective properties. By using structs, you can create cleaner and more organized code, making it easier to maintain and update in the future.
Does Java Have Struct?
Java does not have a struct data type. Structs are a type of composite data type that are commonly used in C, C++, and other languages. In contrast, the primary composite data types in Java are classes and interfaces. These types allow you to define custom data structures that can contain multiple fields or properties, including other objects, arrays, and primitive types. Classes can also contain methods, which allow you to define behavior and functionality for the data type. In Java, you can define classes and interfaces using the class and interface keywords, respectively. While Java does not have a direct equivalent to structs, you can use classes and interfaces to build similar types of composite data structures.
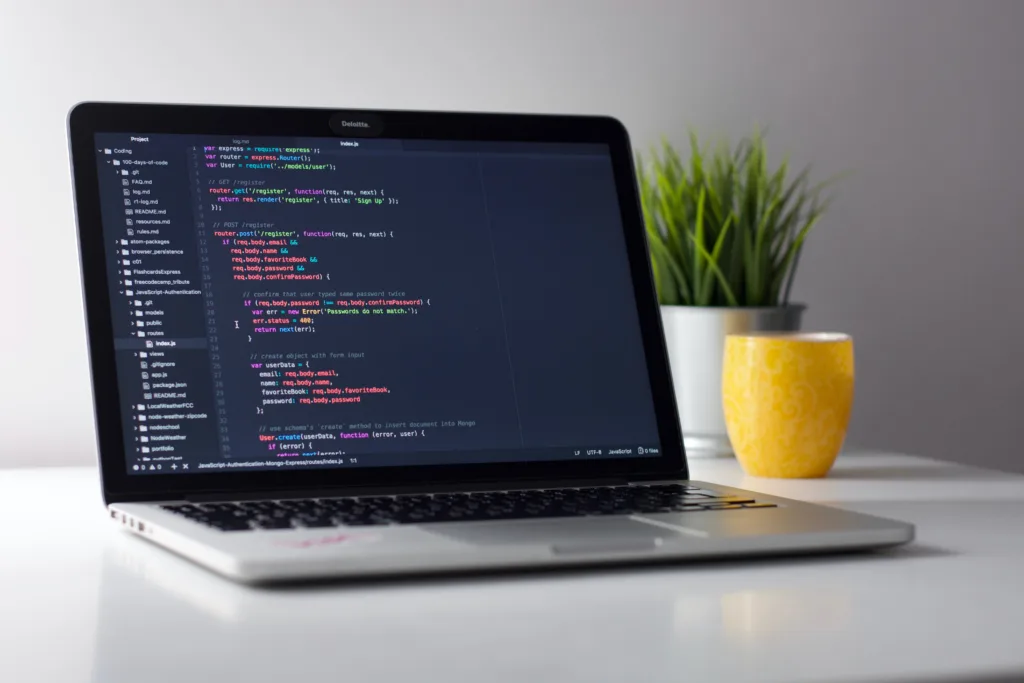
What Are Structs Used For?
Structures, or structs, are used in programming to group several related variables into one place. They are commonly used in situations were you need to store and manipulate multiple pieces of information that are related to each other. Structs are especially useful when you want to pass a large amount of data to a function or when you need to keep track of multiple pieces of data in an organized way.
Here are some common uses for structs in programming:
– Storing information about a person, such as their name, age, and address
– Storing information about a car, such as its make, model, and year
– Storing information about a point in 3D space, such as its x, y, and z coordinates
– Storing information about a date, such as its day, month, and year
Structs can contain many different data types, including integers, floats, characters, and even other structs. By grouping related variables together in a struct, you can make your code more organized and easier to read and maintain. Additionally, you can pass structs as arguments to functions, making it easier to work with large amounts of data.
How To Create Struct In Java?
To create a Struct object in Java, you need to follow these steps:
1. Create an instance of OracleConnection:
You need to create an instance of the OracleConnection class by using DriverManager.getConnection() method which will return you the instance of this class.
2. Create a Java Object array:
You need to create a Java Object array with the same number of elements as there are attributes in the database data type. Populate the array with Java objects of the appropriate data type.
3. Create a StructDescriptor object:
You need to create a StructDescriptor object using the oracle connection and the name of the Struct in the database. This object helps in describing the structure of the Struct in the database.
4. Create a Struct object:
You need to create a Struct object using the StructDescriptor and the Java Object array. This Struct object represents the Struct in Java.
Here’s an example code snippet to create a Struct object in Java:
“`
OracleConnection connection = (OracleConnection) DriverManager.getConnection(url, username, password);
Object[] attributes = new Object[3];
Attributes[0] = “John”;
Attributes[1] = “Doe”;
Attributes[2] = 30;
StructDescriptor structDescriptor = StructDescriptor.createDescriptor(“PERSON”, connection);
Struct struct = new STRUCT(structDescriptor, connection, attributes);
“`
In this example, we have created a Struct object for the PERSON Struct in the database with three attributes – frst name, last name, and age.
What Is Struct Datatype In Java?
In Java, a struct datatype is a composite type that allows you to group different properties together, where each property can have its own type and value. It is a way to represent a real-world entity with its various properties in a program.
One key advantage of usig a struct is that it allows you to access all of the property data as a single entity, which can help simplify your code and make it easier to work with. Additionally, because structs are value types, they can be constructed using the new() function.
Some important features and characteristics of struct datatype in Java include:
– Structs are composite types, meaning they can contain multiple properties with different types and values.
– Structs allow you to group related data together and access it as a single entity.
– Structs are value types, which means they are passed by value rather than reference.
– Structs can be created and initialized using the new() function.
– Structs are often used to represent real-world entities in software applications, such as customers, orders, or products.
A struct datatype in Java is a composite type that allows you to group related data together and access it as a single entity. It is a valuable tool for representing real-world entities in software applications, and can help simplify your code and make it easier to work with.
Conclusion
While the Java language does not support the struct or union data types, developers can still achieve similar functionality trough the use of classes or interfaces. Structs are a way to group related variables together into a single composite type, allowing for easy access and manipulation of the data. With structs, developers can represent real-world entities with their properties and values, and construct them using the new() function. By following the steps to insert an object into a database using a Struct object, developers can efficiently store and retrieve data. understanding and implementing structs in Java can greatly enhance the organization and efficiency of programming projects.