If you’re new to Python programming, you may be wondering what parentheses are and how they’re used. Parentheses are a type of punctuation mark that are used in Python to group expressions, define tuples, and call functions. In this article, we’ll explore each of these uses in detail.
One of the most common uses of parentheses in Python is to group expressions. When you place an expression inside parentheses, it tells Python to evaluate that expression first. For example:
X = 2 + 3 * 4
Y = (2 + 3) * 4
In the first line, Python evaluates 3 * 4 first, then adds 2 to the result. In the secod line, Python evaluates 2 + 3 first, then multiplies the result by 4. Without the parentheses, Python would evaluate the expressions from left to right, resulting in a different answer.
Another use of parentheses in Python is to define tuples. A tuple is an ordered, immutable collection of elements. You can define a tuple by placing comma-separated values inside parentheses. For example:
My_tuple = (1, 2, 3)
Tuples are similar to lists, but they cannot be modified once they are created. You can access individual elements of a tuple using indexing, just like with a list:
First_element = my_tuple[0]
Parentheses are used in Python to call functions. A function is a block of code that performs a specific task. When you call a function, you pass in any necessary arguments inside parentheses. For example:
Print(“Hello, world!”)
In this line of code, we’re calling the print() function and passing in the string “Hello, world!” as an argument. The function then prints that string to the console.
Parentheses are a fundamental part of Python programming. They’re used to group expressions, define tuples, and call functions. By understanding how to use parentheses correctly, you can create more complex and powerful programs in Python. Remember, always use parentheses to group expressions when you want to ensure the correct order of operations, define tuples when you need an immutable collection of elements, and call functions to execute blocks of code. Happy coding!
What Is () And [] In Python?
In Python, () and [] are both symbols that are used to denote different types of collections. () refers to a tuple, wich is an immutable collection of values. This means that once a tuple is created, its contents cannot be changed. Tuples can contain values of different types, such as integers, strings, or even other tuples.
On the other hand, [] refers to a list, which is a mutable collection of values. This means that lists can be modified after they are created by adding, removing, or changing their contents. Lists can also contain values of different types, just like tuples.
It is important to note that while tuples and lists may have some similarities, they are fundamentally different types of collections. Tuples are often used when you want to group together related values that should not be changed, while lists are used when you want to maintain a dynamic collection of values that can be updated as needed.
() and [] are symbols used to represent different types of collections in Python. () represents a tuple, which is an immutable collection of values, while [] represents a list, which is a mutable collection of values.
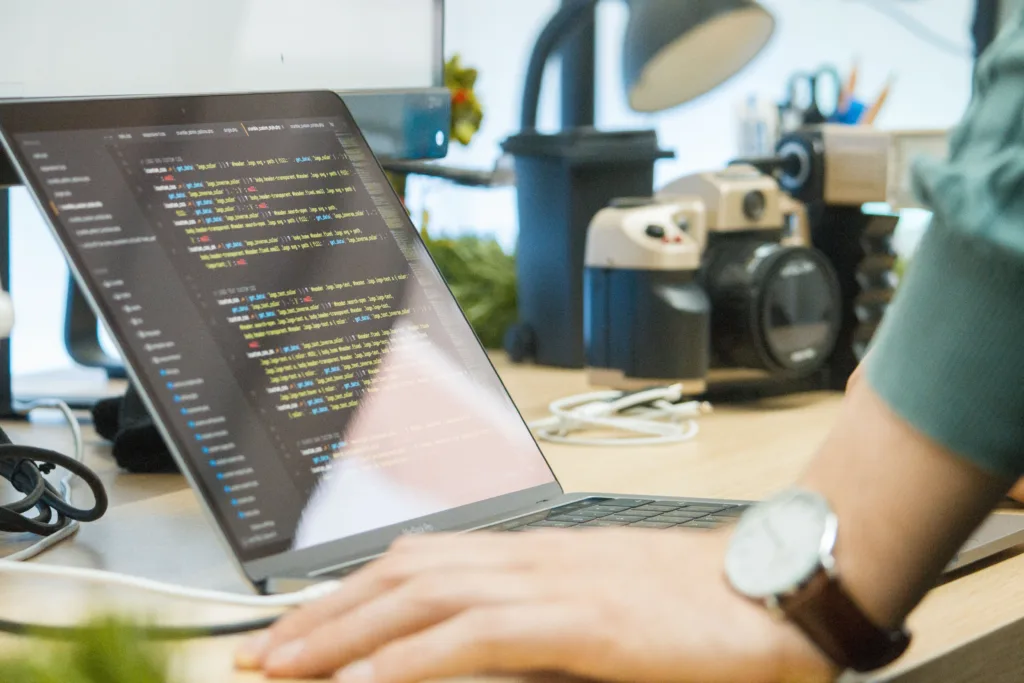
What Data Type Uses Parentheses In Python?
In Python, the data type that uses parentheses is called a tuple. Tuples are similar to lists, but they are immutable, meaning that once they are created, their values cannot be changed. The syntax for creating a tuple involves enclosing the elements within parentheses and separating them with commas. Tuples are often used for grouping related data together, such as coordinates or pieces of information that belong together. Unlike lists, tuples cannot be modified by adding, removing or changing their elements.
What Is A () Called?
A parenthesis is a punctuation mark that consists of a pair of curved vertical lines: ( ). It is primarily used to add nonessential information or an aside to a sentence. The term “parenthesis” can refer to a single one of these punctuation marks or to a set of them used together. Parentheses are commonly used in writing, particularly in academic or technical writing, to provide additional information or clarify a point without disrupting the flow of the main sentence. They can also be used to indicate an interruption or change in thought.
Conclusion
Parentheses are an important part of the Python programming language. They are used for a variety of purposes, including grouping expressions, passing arguments to functions, and creating tuples. While parentheses may seem simple, understanding their proper usage can greatly enhance the readability and functionality of your code. By using parentheses correctly, you can ensure that your Python programs are efficient, effective, and easy to understand. Remember to always use parentheses when they are required, and to use them in a clear and consistent manner throughout your code.