If you’re working with Git, you might sometimes encounter untracked files that you don’t want to include in your repository. Unfortunately, these files can sometimes cause issues when you try to pull from a remote repository. In this blog post, we’ll walk through the steps you can take to properly git clean untracked files and ensure a smooth git pull.
Step 1: Run ‘git clean -n’
Before you start deleting untracked files, it’s important to see a dry run of what will be deleted. To do this, run ‘git clean -n’. This will show you a list of untracked files that will be deleted if you proceed with the next step.
Step 2: Run ‘git clean -f’
Once you’ve reviewed the list of files that will be deleted, you can proceed with actually deleting them. Run ‘git clean -f’ to force the deletion of untracked files. This will remove any untracked files that are not igored by your .gitignore file.
Step 3: Use ‘git clean -f -d’
If you have untracked directories that you want to delete, you can use ‘git clean -f -d’. This will remove untracked directories and their contents from your repository.
Step 4: Use ‘git clean -f -x’
If you have untracked files that are ignored by your .gitignore file, you can use ‘git clean -f -x’ to remove them. This will force the deletion of all untracked files, including those that are ignored.
Step 5: Add the -i switch for an interactive git clean
If you want to interactively choose which files to delete, you can add the -i switch to your git clean command. This will prompt you to confirm each deletion before it occurs.
Step 6: Run ‘git reset –hard origin/master’ to force a git pull
Now that you’ve properly cleaned up your untracked files, you can safely pull from a remote repository. Run ‘git reset –hard origin/master’ to force a git pull from the remote repository. This will overwrite any local changes you have that are not committed, so be sure to commit any changes you want to keep before running this command.
Cleaning up untracked files can be a bit tricky in Git, but by following these steps, you can ensure that your repository is clean and ready for a smooth git pull. Remember to always review the list of files that will be deleted before running ‘git clean -f’, and use the -i switch if you want to interactively choose which files to delete. With these tips in mind, you’ll be able to keep your Git repository organized and efficient.
Pulling Untracked Files in Git
When working with Git, you may come across untracked files that you want to pull into your repository. Fortunately, Git provides a simple command to accomplish this task.
To pull untracked files in Git, follow these steps:
1. First, make sure you are in the correct directory. You can use the command `cd` followed by the directory path to navigate to the correct folder.
2. Next, use the `git add` command to add the untracked files to the staging area. You can spcify the files you want to add by name, or use a wildcard to add all untracked files. For example, to add all untracked files, use the command `git add .`.
3. Once the files are added to the staging area, use the `git commit` command to commit the changes to the repository.
4. use the `git pull` command to pull any changes from the remote repository. This will ensure that your local repository is up to date with the latest changes.
It’s important to note that if there are any conflicts between the changes you’ve made locally and the changes in the remote repository, you may need to resolve them before you can successfully pull the changes. In this case, you can use the `git stash` command to temporarily save your changes, pull the changes from the remote repository, and then apply your changes again using the `git stash apply` command.
In summary, to pull untracked files in Git, you’ll need to add the files to the staging area, commit the changes, and then use the `git pull` command to pull any changes from the remote repository.
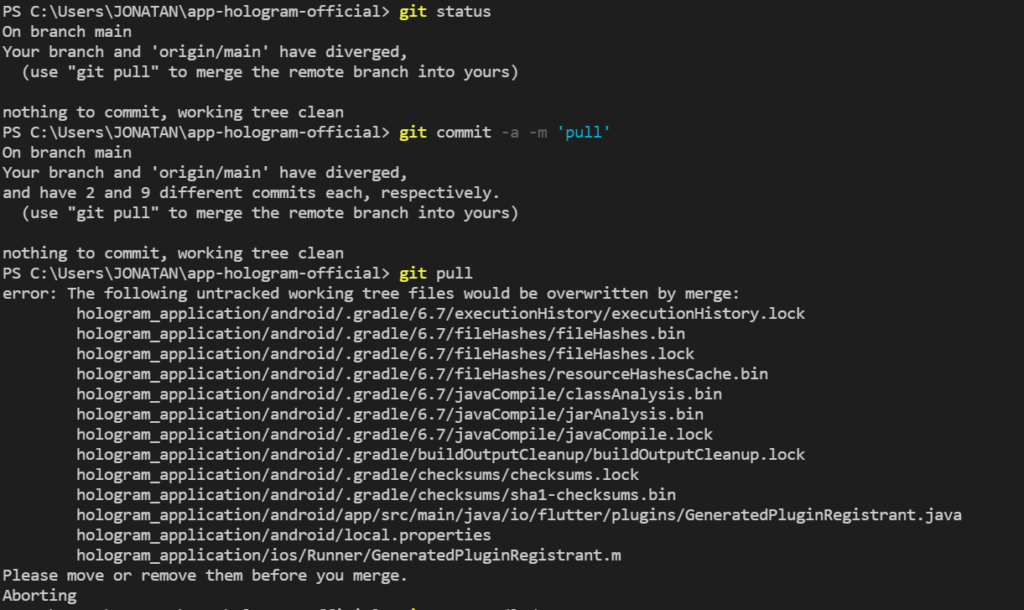
Does Git Pull Affect Untracked Files?
Git pull is a command that is used to update a local repository with the changes made to a remote repository. When you use the git pull command, it will fetch the changes from the remote repository and merge them into your local repository. However, it will not affect any untracked files in your working directory.
Untracked files are files that have not been added to the Git repository yet. These files are not bing tracked by Git, so Git does not know about them. When you use the git pull command, it will only affect the files that are being tracked by Git. Untracked files will not be affected by the git pull command.
It is important to note that if you have made changes to any untracked files in your working directory, those changes will not be lost when you use the git pull command. Git will not delete or modify any untracked files in your working directory.
The git pull command only affects tracked files in your repository and does not affect any untracked files in your working directory.
Handling Untracked Files in Git
When working with Git, you may come across untracked files. These are files that Git is not currently keeping track of. They are usually new files that have not yet been added to the repository.
If you don’t want these files to be included in your repository, you have a few options:
1. Delete the file: If you don’t need the file, you can simply delete it from your filesystem. Git won’t track it anymore, and it won’t cause any issues with your repository.
2. Ignore the file: If you want to keep the file, but don’t want Git to track it, you can add it to your .gitignore file. This file contains a list of files and directories that Git should ignore. You can create a .gitignore file in the root directory of your repository, and add the filenames or patterns of the files you want to ignore.
3. Add the file to Git: If you want to include the file in your repository, you can add it to Git using the command “git add “. This will stage the file for commit, and it will be included in your next commit.
To check the status of your files in Git, you can use the command “git status”. This will show you whch files are untracked, modified, or staged for commit. By understanding the status of your files, you can make informed decisions about what to do with them in your repository.
Forcing Git to Pull a File
Git is a powerful tool for version control and collaboration on software projects. It allows developers to work on the same codebase simultaneously, and merge chnges seamlessly. However, there are times when you may need to force Git to pull a file from a remote repository, in order to ensure that your local copy is up-to-date.
To force Git to pull a file, you can use the command “git reset –hard origin/master”. This command will reset your local copy of the repository to the state of the “origin” (i.e. the remote) repository’s “master” branch, discarding any local changes you may have made.
Before running this command, it is important to make sure you have a backup of any changes you have made locally, in case you accidentally lose them. To do this, you can use the command “git stash” to temporarily store your changes, and then use “git stash apply” to reapply them after the reset.
In addition to the command mentioned above, there are a few other commands you may need to use in order to ensure that your local copy is fully up-to-date with the remote repository. These commands include:
– “git fetch –all”: This command will fetch all branches from the remote repository, so that you have the latest changes available locally.
– “git checkout master”: This command will switch your local copy to the “master” branch of the repository, so that you can ensure you are pulling the latest changes from that branch.
– “git pull”: This command will pull the latest changes from the remote repository into your local copy, so that you have the most up-to-date version of the code.
By using these commands in combination, you can ensure that your local copy of the repository is fully up-to-date with the remote repository, and that you have the latest changes available to work with.
Understanding Git Fetch — Unshallow
Git is a popular version control system that allows developers to collaborate on a project, keep track of changes, and manage their codebase. When you clone a Git repository, you create a local copy of the repository on your machine. By default, Git clones the repository as a “shallow” clone, which means that it only downloads the most recent version of the repository’s history.
If you want to access the full history of a repository, you can use the “git fetch –unshallow” command. This command converts a shallow clone to a regular one by downloading the full history of the repository, including all the branches and tags.
Here’s how you can use the “git fetch –unshallow” command:
1. Open your terminal or command prompt and navigate to the directory where your Git repository is located.
2. Use the following command to convert the shallow clone to a regular one:
Git fetch –unshallow
3. Git will download the full history of the repository and update your local copy. This may take some time depending on the size of the repository and your internet connection speed.
4. Once the command completes, you can use the “git branch” command to see all the branches in the repository, including the ones that were not avilable in the shallow clone.
“git fetch –unshallow” is a Git command that allows you to convert a shallow clone to a regular one by downloading the full history of the repository. This command is useful if you want to access all the branches and tags in the repository and work with the complete history of the project.
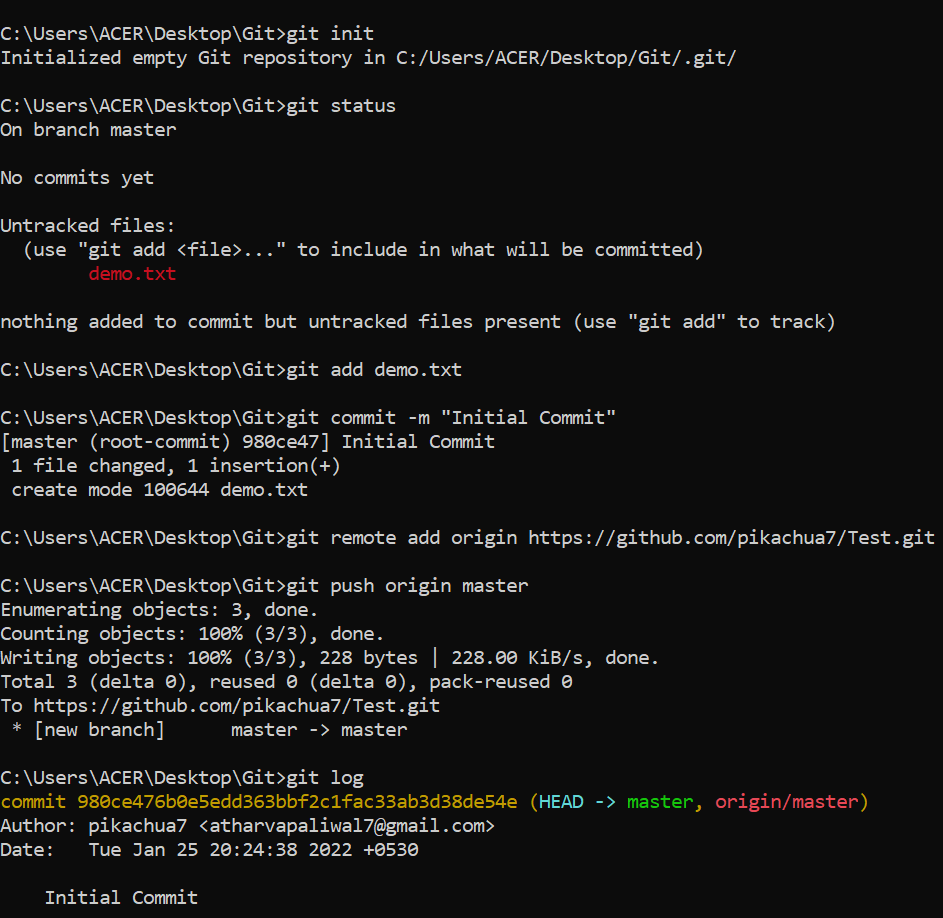
Retrieving All Files From Git
To pull all files from git, follow these steps:
1. Open your terminal or command prompt and navigate to your desired directory where you want to clone the repository.
2. Use the git clone command to clone the repository. For example, if the repository URL is https://github.com/username/repo.git, the command would be:
“`
Git clone https://github.com/username/repo.git
“`
3. Once the repository is cloned, navigate to the cloned directory using cd command. For example, if the repository is named “repo”, the command would be:
“`
Cd repo
“`
4. To pull all the changes made in the repository, run the git pull command. This will update your local copy of the repository with the latest changes made in the remote repository. For example:
“`
Git pull
“`
5. If tere are multiple branches in the repository and you want to pull changes from all the branches, use the git fetch –all command. This will fetch all the changes made in all the branches. For example:
“`
Git fetch –all
“`
6. if there are any branches that no longer exist in the remote repository and you want to delete them from your local repository, use the git fetch –all –prune command. This will remove any local branches that no longer exist in the remote repository. For example:
“`
Git fetch –all –prune
“`
By following these steps, you can pull all files from git and keep your local copy of the repository up-to-date with the latest changes made in the remote repository.
Why Git Pull Is Not Functioning Properly
When you execute the git pull command, it performs two separate actions: fetching new commits from the remote repository and merging them into your local branch. If you have uncommitted changes in your local branch, the merge part of the git pull command will fail because Git does not want to overwrite your changes with potentially conflicting changes from the remote repository. Therefore, you need to commit your local changes before pulling new commits from the remote repository.
To commit your changes, use the git add command to stage the changes you want to include in the commit, followed by the git commit command to create a new commit with a descriptive message. Once you have committed your changes, you can safely execute the git pull command to fetch and merge new commits from the remote repository.
It’s also worth noting that there are oter reasons why the git pull command may fail, such as conflicts with the remote repository or issues with your network connection. In these cases, you may need to troubleshoot the specific error message or consult with your team or Git support resources to resolve the issue.
Does ‘Git Pull’ Pull All Files?
Git pull is a command used to fetch and download content from a remote repository and immediately update the local repository to match that content. However, it is important to note that git pull does not pull everything by default.
When you run git pull, Git will fetch the changes from the remote repository and merge them into your local repository. This process will only pull the changes made to the branch that you have specified. If you have multiple branches in your remote repository, you need to specify which branch you want to pull from.
Git pull command can also be used with options to control the extent of the fetch and merge operations. For example, if you only want to fetch the changes from the remote repository without merging them into your local repository, you can use the –no-merge option. Similarly, you can use the –rebase option to rebase your local repository onto the updated remote repository instead of merging it.
The git pull command does not pull everything by default. It only pulls the changes made to the spcified branch in the remote repository and merges them into the local repository. However, you can use options with the git pull command to control the extent of the fetch and merge operations.
Ignoring Untracked Files
Ignoring untracked files in Git is a crucial task to keep your repository clean and organized. Fortunately, Git provides a simple and effective solution to this issue by using a .gitignore file.
The .gitignore file is a plain text file that contains a list of files and directories that Git should ignore. To create this file, you can use any text editor and save it to the root directory of your repository. You can name the file .gitignore, and Git will automatically recognize it.
Inside the .gitignore file, you can specify the files and directories that you want Git to ignore by adding their names or patterns. For example, if you want to ignore all files with the .log extension, you can add the following line to the .gitignore file:
*.log
This will tell Git to ignore all files with the .log extension in your repository.
In addition to the .gitignore file, Git also provides two commands to remove untracked files from your repository: git clean -fx and git clean -fd.
The git clean -fx command will remove all untracked files, including ignored files, from your repository. This command is useful when you want to clean up your repository and remove all unnecessary files.
The git clean -fd command will remove all untracked files and directories from your repository. This command is useful when you want to remove entire directories that cntain untracked files.
Ignoring untracked files in Git is crucial to keep your repository clean and organized. You can achieve this by using a .gitignore file, which contains a list of files and directories that Git should ignore. Additionally, you can use the git clean command to remove untracked files from your repository.
Source: code.visualstudio.com
Understanding Untracked Files in Git
When you add a new file to your Git repository, it is initially untracked. This means that Git is aware of the file’s existence, but it has not yet been added to Git’s internal database. Untracked files are essentially ignored by Git until you explicitly tell it to start tracking them.
There are a few reasons why a file might be untracked in Git. One common scenario is when you create a new file in your working directory. Git will not automatically start tracking this file, as it doesn’t know whether or not you want it to be part of your repository.
Another reason why a file might be untracked is if you have recently cloned a repository from a remote source. When you clone a repository, Git copies all of the files and commits from the remote server to your local machine. However, these files are not automatically tracked until you explicitly add them to your Git repository.
To start tracking an untracked file in Git, you need to use the “git add” command. This command tells Git to add the file to its internal database and start tracking it. Once a file is tracked, Git will start monitoring it for changs and include it in future commits.
Here are some key takeaways about untracked files in Git:
– Untracked files are files that have not yet been added to Git’s internal database.
– There are several reasons why a file might be untracked, including creating a new file or cloning a repository.
– To start tracking an untracked file, you need to use the “git add” command.
– Once a file is tracked, Git will start monitoring it for changes and include it in future commits.
Adding All Untracked Files to Git
When working with Git, it’s common to have new or untracked files that need to be added to your repository. Fortunately, thre is a simple command you can use to add all untracked files to your Git repository.
First, open your command line interface and navigate to the root directory of your repository. Once there, use the following command:
“`
Git add -A
“`
This command tells Git to add all files, including new and untracked files, to your staging area. The “-A” option stands for “all” and is what tells Git to add everything.
If you only want to add new files and not modified files, you can use the following command instead:
“`
Git add .
“`
This command will only add new files to your Git repository, but not modified files.
It’s important to note that once you’ve added files to your staging area, you still need to commit them to your repository. To do this, use the following command:
“`
Git commit -m “Your commit message here”
“`
This command will commit all staged changes to your repository, along with a message describing the changes you made.
In summary, to add all untracked files to your Git repository, use the “git add -A” command. Remember to also commit your changes after adding them to your staging area.
Untracked Git Files
Git is a popular version control system used by developers worldwide to manage changes to their codebase over time. When you create a new file in a git repository, it is not automatically tracked by the version control system. Instead, it is considered an “untracked” file until you explicitly tell git to start tracking it.
There are several reasons why a file may be untracked in a git repository:
1. New files: When you create a new file in the repository, it is not automatically tracked by git. You need to use the “git add” command to add the file to the staging area, after which it will be tracked by git.
2. Ignored files: Sometimes, you may have files in your repository that you do not want to track with git. These files can be ignored by creating a “.gitignore” file in the root directory of your repository and listing the files or directories to be ignored.
3. Deleted files: If you delete a file from your local repository, it will still be tracked by git until you use the “git rm” command to remove it from the repository.
4. Renamed files: When you rename a file in your local repository, git considers it as an untracked file until you use the “git add” command to add the renamed file to the staging area.
Untracked files in git are those that are not being monitored by the version control system. They can be new files, ignored files, deleted files, or renamed files that have not yet been added to the staging area using the “git add” command.
The Benefits of Using Git Force Pull
Git force pull is a command used to forcefully update the local branch of a Git repository with the changes made in the remote branch, even if there are conflicts. This command is useful when you want to discard your local changes and replace them with the changes made in the remote repository.
The standard Git pull command merges the changes made in the remote branch with the local branch. However, if there are conflicts betwen the files in the local and remote branches, the merge may fail. In such cases, you can use the Git force pull command to overwrite the local branch with the changes from the remote branch.
It is important to note that using Git force pull can result in the loss of local changes. Therefore, it should be used with caution, and you should always ensure that you have a backup of your local changes before using this command.
Here are the steps to perform a Git force pull:
1. Open the Git Bash or Terminal and navigate to the local repository.
2. Run the command “git fetch” to fetch the changes from the remote repository.
3. Run the command “git reset –hard origin/branch-name” to reset the local branch to the state of the remote branch.
4. Run the command “git pull” to merge the changes from the remote branch into the local branch.
Git force pull is a command used to override the local branch’s history and files with the changes from the remote branch. This command should be used with caution as it can result in the loss of local changes.
Source: gitforwindows.org
Pulling Files From a Branch
To pull files from a branch in Git, you need to follow a few simple steps. First, make sure you are in the branch where you want to pull the files. You can do this by using the command `git checkout branch_name`.
Once you are in the right branch, you can use the `git pull` command to fetch and merge chnges from the remote repository. This will update your local branch with the latest changes from the remote branch.
If you only want to pull specific files from the branch, you can use the `git checkout` command with the file path to retrieve the file from the branch. For example, to pull a file named `example.txt` from a branch named `feature-branch`, you can use the following command:
“`
Git checkout feature-branch — path/to/example.txt
“`
This will retrieve the file from the `feature-branch` and place it in your current working directory.
Alternatively, you can use the `git fetch` command to retrieve the changes from the remote branch without merging them into your local branch. This can be useful if you want to review the changes before merging them into your local branch.
Once you have pulled the files or changes from the remote branch, you can commit them to your local branch using the `git commit` command and push them to the remote repository using the `git push` command.
The steps to pull files from a branch in Git are:
1. Switch to the branch where you want to pull the files.
2. Use the `git pull` command to fetch and merge changes from the remote branch.
3. Use the `git checkout` command with the file path to retrieve specific files from the remote branch.
4. Use the `git fetch` command to retrieve changes from the remote branch without merging them.
5. Commit the changes to your local branch using `git commit`.
6. Push the changes to the remote repository using `git push`.
Enabling a Pull Request
If you’re the owner or collaborator of a repository, enabling a pull request is a simple process. When someone submits a pull request, you’ll receive a notification, and you can access the pull request by navigating to the “Pull requests” tab on the repository’s main page.
To enable the pull request, click on the pull request to open it. You’ll see a green “Merge” button if the pull request can be merged wihout any conflicts. If there are conflicts, you’ll see a message indicating that the pull request cannot be merged automatically.
To merge the pull request, click the “Merge” button, and then click the “Confirm merge” button to complete the process. Once you’ve merged the pull request, the changes will be added to the repository.
If you want to allow others to make changes to your pull request, you can select the “Allow edits from maintainers” option. This will allow anyone with push access to the upstream repository to make changes to your pull request.
Enabling a pull request involves opening the pull request, checking for conflicts, and clicking the “Merge” button to merge the changes. You can also allow edits from maintainers to enable others to make changes to your pull request.
Conclusion
When it comes to managing files in Git, it’s important to understand how to properly clean up untracked files and directories. To do so, you can use the git clean command with various options such as -n, -f, -d, and -x. The -n option will show a dry run of what files and directories will be deleted, while -f will force the deletion of untracked files. The -d option is used to remove untracked directories, and the -x option will remove untracked files that are ignored by Git.
It’s worth noting that git pull does not delete uncommitted changes, and it won’t affect your working directory. Therefore, any changes or new files will not be touched unless they are committed. If you want to force a git pull from a remote repository, you can use the command git reset –hard origin/master. However, it’s recommended to make a backup of your data to prevent any data loss.
Understanding how to manage untracked files in Git is essential for maintaining a clean and organized repository. By using the git clean command with the approprite options, you can remove unwanted files and directories safely. Additionally, it’s important to remember that git pull won’t delete uncommitted changes and to always make a backup of your data before making any significant changes to your repository.