When it comes to inheritance in object-oriented programming, the question of whether or not a child class can access private members of its parent class often arises. The answer is a resounding no, but let’s take a closer look at why this is the case.
Inheritance is an incredibly powerful tool in OOP, allowing us to build upon existing classes and create new classes that have all the features of their parent class as well as any additional features we add. However, one thing that inheritance does not give us access to are private members of the parent class. Private members are variables and methods that are declared within a class but cannot be accessed outside of it. This means that even thouh a child class can see private variables and methods declared within it’s parent class, it cannot actually access them directly.
The reason for this is simple: if a child class were able to access and change the private members of its parent class, it could potentially break the functionality of code written for the parent class. For example, if a child class were able to modify or delete private methods or variables from its parent, it could cause unexpected results when those methods and variables are accessed elsewhere in code.
So how can we work around this limitation? The answer lies in using getter and setter methods, which allow us to read data from and write data to private variables without actually accessing them directly. By writing these getter/setter methods into our classes (and marking them public), we can allow other classes to read/write data from our private members without actually exposing those members themselves. This way, we ensure that our code remains secure while still allowing other classes to interact with our private members safely.
In conclusion, while inheritance allows us to create powerful classes by building off existing ones, it does not allow us to directly access the private members of our parents’ classes due to security concerns. The only way around this limitation is by creating public getter/setter methods which allow other classes (including child classes) to interact with those private members without exposing them directly.
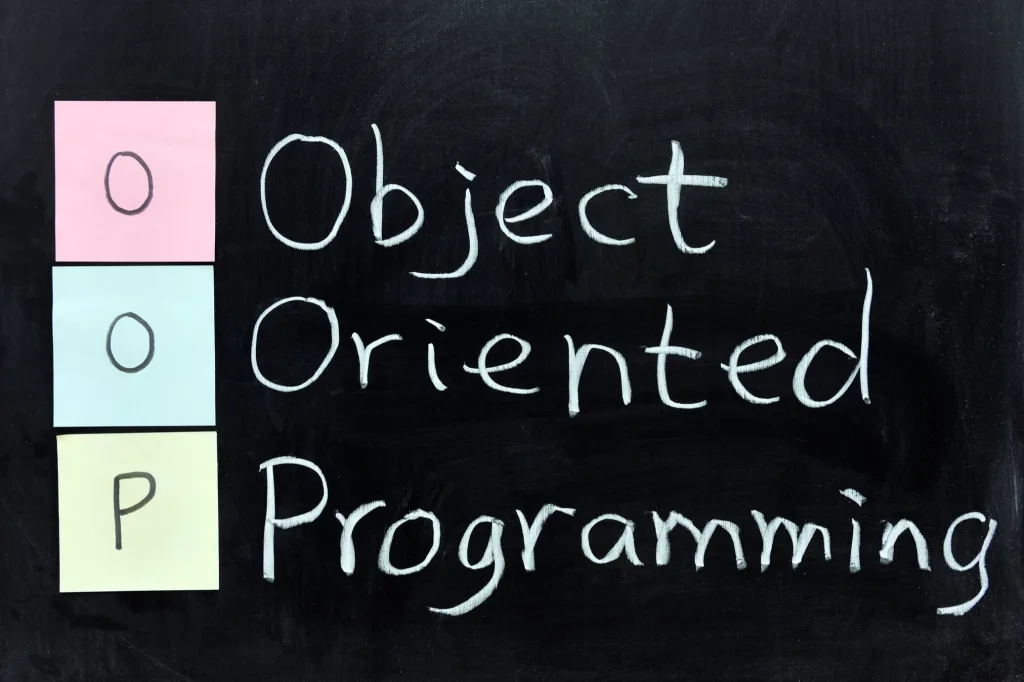
Can a Child Class Access Private Members?
No, a child class cannot directly access private members inherited from its parent class. Private members are only accessible within the class in which they are defined, and cannot be accessed even by subclasses or other classes in the same package. In order to access private fields and methods, the parent class must provide public getter and setter methods, or the child class must override the parent’s methods to access them internally.
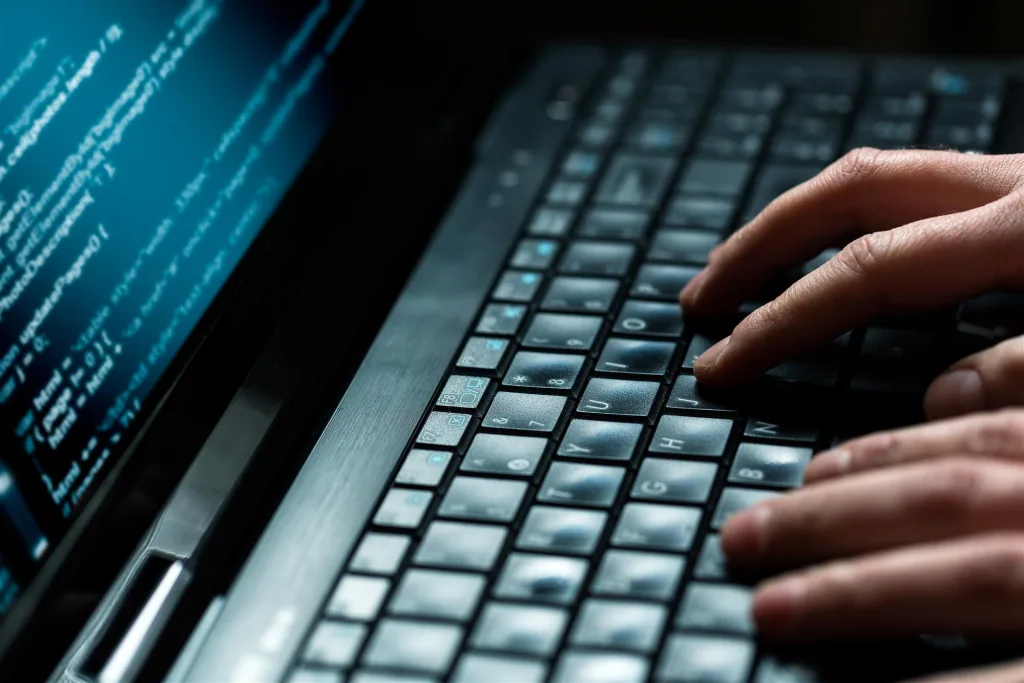
Accessing Private Members of a Parent Class
In order to access the private members of a parent class, you can use either a friend function or a member function of the child class. A friend function is a non-member function that is declared as a friend of a class, and thus has access to the private members of that class. Alternatively, you can create a member function in the child class which uses the ‘protected’ keyword instead of ‘private’, allowing it to access the parent’s private members. It is important to note that tese methods should be used with caution and only when absolutely necessary, as they can potentially lead to security issues and violation of object-orientated programming principles.
Accessing Parent Class from Child Class
Yes, a child class can access its parent class. This is typically done using inheritance, which allows the child class to inherit all of the parent class’ methods and properties. The child class can then use these inherited methods and properties to access any data within the parent class. Additionally, the child can override or extend existing methods or even add new ones of its own. This allows for a greater degree of customization in terms of how the data within the parent class is used.
Accessing Private Members in a Child Class in Java
In Java, private members of a superclass are not accessible to the child class. To access these private members in the child class, you must use setter and getter methods. This is because setter and getter methods allow you to both read from and write to private members of the superclass without having direct access to them. To call these setter and getter methods within the child class, you will need to create an instance of the subclass and then use that instance to invoke the desired method. For example, if we have a subclass called MySubClass with a method called myMethod(), we can create an instance of MySubClass like this:
MySubClass subClassObject = new MySubClass();
We can then use the object created abve (subClassObject) to invoke myMethod():
subClassObject.myMethod();
This way, we can access private members in child classes without breaking encapsulation principles or introducing any security risks.
Accessing Private Members of a Parent Class in Java by a Child Class
No, a child class cannot directly access private members of its parent class in Java. Private members are only accessible by the class in which they are declared. However, if the superclass has public or protected methods for accessing its private fields, these can also be used by the subclass. For example, if the superclass contains a method called “getName()” that returns the value of a private field called “name”, then this method can be accessed from within the child class to return the value of “name”.
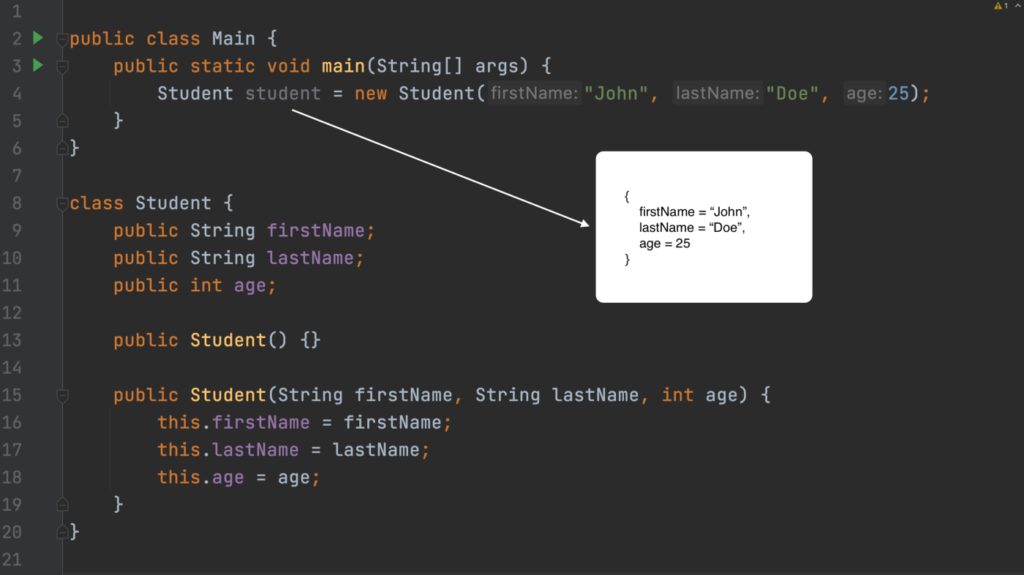
Accessing Private Members of Parent Class in Child Class in C++
No, child classes cannot access private members of parent classes in C++. This is because private members of a class are only accessible to that class itself and its member functions. Even though the child class is derived from the parent class, it does not automatically gain access to the private members of the parent class. In order to allow the child class to access private members of a parent class, friend declarations must be made within the base class explicitly granting such access.
Accessing Private Members in a Java Class
Private members in a Java class are accessible only to the class itself. This means that any methods, fields, or othr properties declared as private can only be accessed within the same class. However, there is a way to access private members from outside of the class using the Java Reflection API. This allows developers to bypass the default access control mechanisms and gain access to private members. The Java Reflection API provides a set of classes and interfaces for reflection which allows for the manipulation of classes at runtime. By using these APIs, developers can create an instance of a class with full access to its private members, allowing them to read and modify them as needed.
Can Private Members be Accessed by Friend Classes?
Yes, friend classes can access private members of another class. A friend class is a special type of class where one class can be declared as a friend of another class, allowing it to access the other class’s private and protected members. The friendship between two classes is mutual and bidirectional, meaning that if Class A is a friend of Class B, then Class B is also a friend of Class A. This means that either class may access the other’s private members.
Accessing Private Members of a Class
The private members of a class can be accessed by two methods: Getter and Setter methods. Getter methods are used to retrieve the value of a private member variable and setter methods are used to assign a value to a private member variable. Both these methods must be declared in the class, so that they can be called from outside the class.
Getter methods typically have no arguments and return the value of the corresponding private member variable. Setter methods usually take an argument and set the corresponding private member variable to that argument’s value.
It is important to note that only these two methods are able to access a class’s private members, because they are specifically declared within the class itself and have been gien permission to access those members. Any other method from outside the class will not be able to access those members, as they do not have permission.
Accessibility of Child Classes
A child class can access all the public, protected, internal and protected internal members of its parent class. This means that any member declared as public in the parent class will be accessible to the child class, as well as any members declared as protected, internal or protected internal. However, private members are not accessible to the child class. Even though they are inherited by the child class, they cannot be directly accessed; instead they must be accessed through a public property (GET/SET modifier).
Can a Child Class Override a Parent Method?
Yes, a child class can override a parent method. This is known as method overriding and it is an important feature of object-oriented programming. It allows the child class to provide its own implementation of a parent class’s method, whle still inheriting the attributes and behaviors of the parent class.
For example, if you have a parent class called Animal, which has a method called move(), then you could create a subclass called Cat which overrides the move() method so that it behaves differently when called from an instance of the Cat class. In this case, the Cat subclass would provide its own implementation of move(), while still inheriting any other attributes and behaviors defined in Animal.
Can a Child Class Throw a Parent Exception?
Yes, a child class can throw a parent exception. This is because when a constructor of the parent class throws any checked exception, then the child class constructor can throw the same exception or its parent exceptions. On the other hand, it is not necessary for a child class to throw any exceptions if the parent class does not throw any exceptions. Furthermore, there is no problem if both the parent and child classes throw unchecked exceptions.
Do Private Variables Get Inherited by Children?
No, children do not inherit private variables. Private variables are members of a class that have been declared as private, meaning they can only be accessed within the class they belong to. Private variables are not visible to other classes, and therefore cannot be inherited by any child classes. If you want a child class to access the value of a private variable, you must prvide public accessor methods for the child class to use. This is how the concept of encapsulation works, by making data private and providing methods to get or set its values so that access can be controlled.
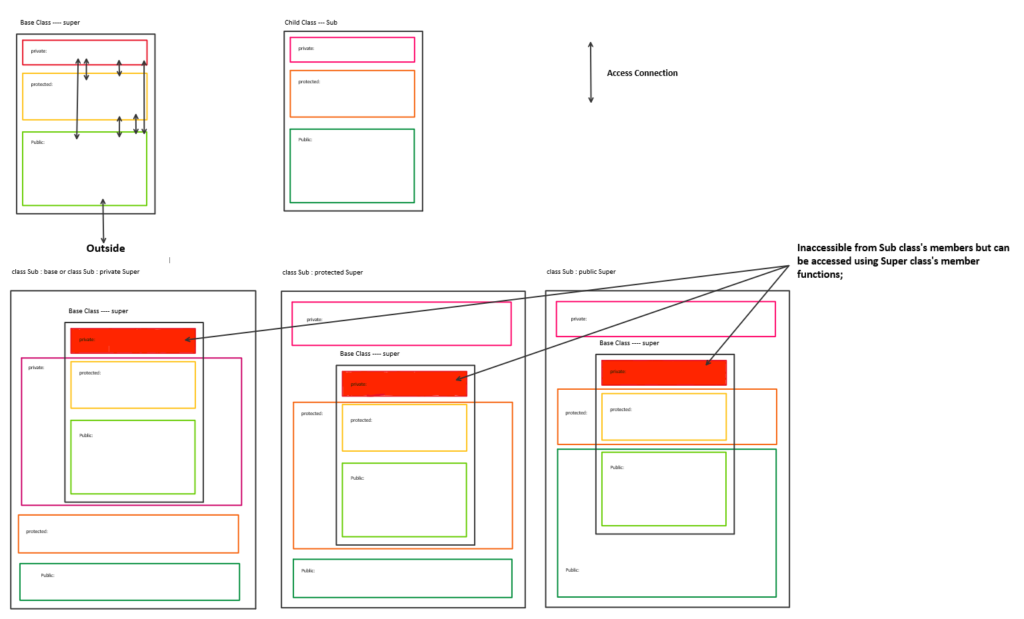
Conclusion
In conclusion, private members of a parent class are not directly accessible by its child class. However, the child class can access the parent’s private members through setter and getter methods. This is done by calling the methods from within the subclass object. This ensures that the data remains secure, while still allowing access to it when needed. As such, it is important to remember that private members of a parent class should be accessed using setter and getter methods in order to ensure secure data access.