Welcome to our blog post on how to print size_t variables in C!
Size_t is an unsigned integer type that is used in the C and C++ programming languages. It is the type of the result returned by the sizeof operator. Its size is chosen so that it can store the maximum size of any theoretically possible array of any type. On a 32-bit system, size_t will take up 32 bits, while on a 64-bit one, it will take up 64 bits.
The correct way to print size_t variables is using “%zu” format. The z in this format indicates a length modifier, while u stands for unsigned type. This makes sense sine size_t is an unsigned integer type.
Let’s look at an example of how to print a size_t variable:
size_t x = 10;
printf(“The value of x is %zu\n”, x);
In this example, we declared variable x with a data type of size_t and assigned it an initial value of 10. When we call printf, we use %zu as the format specifier for variable x since it is a size_t variable. This will result in the following output: The value of x is 10.
If you want to print a floating point number with precision set to 3 decimal places, you should use %.3f as your format specifier instead. For example:
float y = 3.1415;
printf(“The value of y is %.3f\n”, y);
This would result in: The value of y is 3.142 being printed on the console screen.
We hope this blog post provided some useful information about printing size_t variables in C! If you have any further questions or would like more information about this topic please feel free to contact us at anytime!
Printing Size_t Values
To print a size_t variable, you should use the “%zu” format. The “z” in this format is a length modifier and the “u” stands for unsigned type. This is the correct way to print size_t variables as it ensures that the size_t value will be printed correctly regardless of its size. It also ensures that any output from printing a size_t variable can be properly parsed and interpreted by other programs or systems.
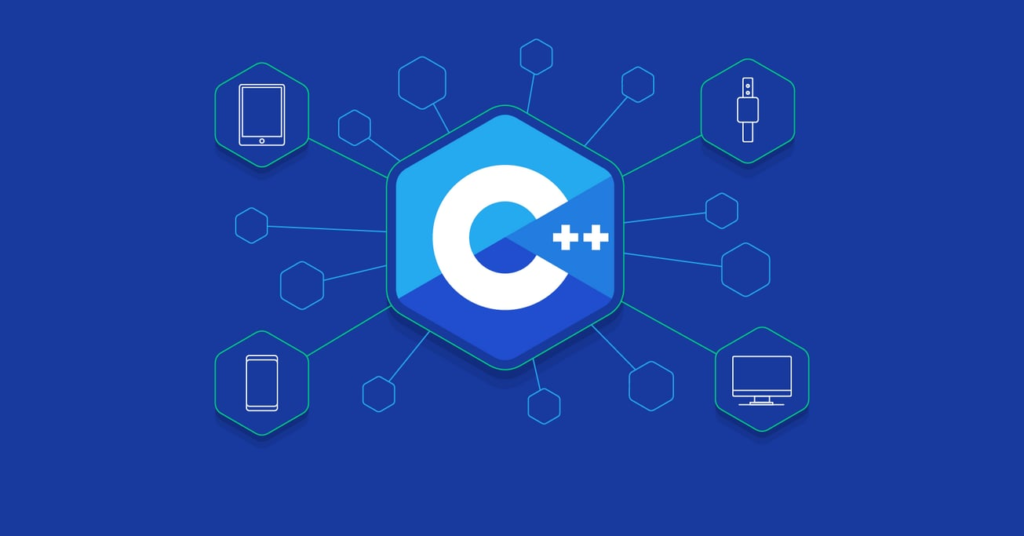
What is size_t in C Programming?
Size_t in C is an unsigned integer data type that is widely used in the C and C++ programming languages. It is used to represent the size of memory blocks or objects, such as arrays, strings, and structs. Size_t is defined in the standard header file and its size depends on the architecture of the computer it’s being run on: for example, on a 32-bit system, it will take up 32 bits; on a 64-bit system, it will take up 64 bits. The size_t type is also used as the return type of the sizeof operator, wich returns the size of an object or type in bytes.
What Does the ‘%3f’ Symbol Represent in C Programming?
In C, the ‘%3f’ symbol is a placeholder for a float variable. When used in a printf() statement, it sets the precision of the float variable to 3 decimal places. For example, if you have the float variable x = 1.234567 and use ‘printf(“%.3f”, x);’, the output would be “1.235”.
Printing an Integer in C
Printing an int in C is very straightforward. All you need to do is use the printf() method, passing the integer as a parameter. The syntax for this is printf(“%d”, variableOfIntegerType). Here, %d is used to indicate that the data type of the parameter passed is of type int. After you write this statement, simply run the program and the int value stored in the variable will be printed on the console screen.
Does Size_t Exist in C?
Yes, size_t is a data type that exists in the C language. It is an unsigned integral type that can hold any value between 0 and the maximum value that the host system can handle. It is typically used to represent the size of objects in bytes, and is therefore often used as the return type by the sizeof operator. This data type is defined in several header files, including , and .
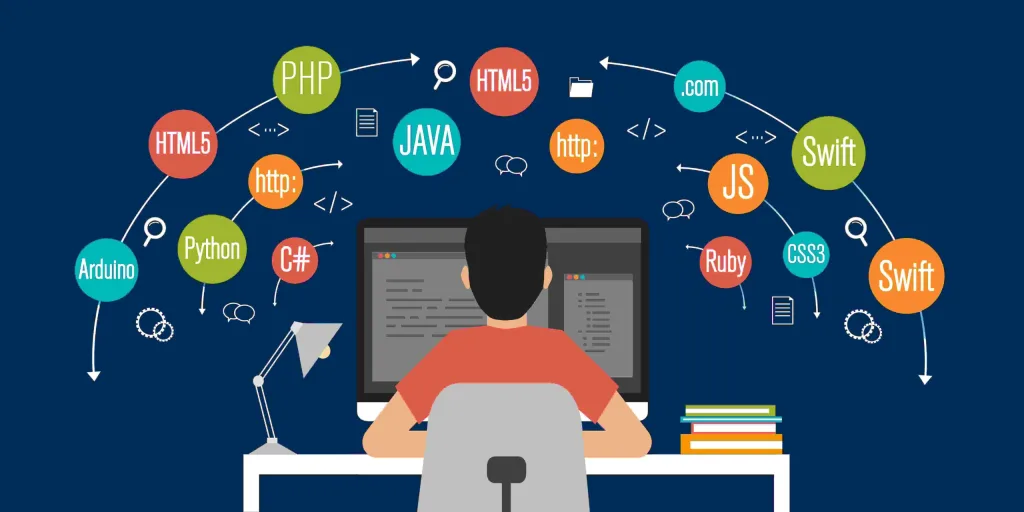
Printing Variable Sizes in C
In C, the sizeof operator can be used to get the size of a variable in bytes. To print the result returned by sizeof, one needs to use either the %lu or %zu format specifier. For example, if we want to find the size of an integer (int) variable in C, we can write:
printf(“Size of int: %lu bytes\n”, sizeof(int));
Similarly, for other data types like float and double variables, we can use the folowing code respectively:
printf(“Size of float: %lu bytes\n”, sizeof(float));
printf(“Size of double: %lu bytes\n”, sizeof(double));
And for character (char) variables, it would be like this:
printf(“Size of char: %lu bytes\n”, sizeof(char));
Are Size_t and Int the Same?
No, size_t and int are not the same. Size_t is an unsigned integer type that is used to represent the size of an object, whereas int is a signed integer type typically used for arithmetic operations. Typically, size_t is larger than int on 64-bit systems. The size and range of both types vary depending on the system architecture and compiler implementation. For example, on a 32-bit system, size_t may be 32 bits while int is 16 bits. As such, they cannot be used interchangeably and one should use the correct type when writing code.
Using size_t in C++
It is generally preferable to use std::size_t over ::size_t, as it is more descriptive and allows the compiler to identify the size of the object. If you have included , then either type can be used. However, if you have not included , then using std::size_t is recommended.
The Benefits of Using Int vs. Size_t
The answer to this question depends on what you’re trying to accomplish. If you are dealing with memory ranges, then size_t is the better choice as it is an unsigned integer type specifically designed for representing the size of objects in bytes. On the other hand, if you’re dealing with integer arithmetic operations, then int is more appropriate as it is designed for that purpose. It’s important to consider both types when deciding which one to use in a given situation so that your code will perform optimally and be easy to read and maintain.
What Does %4d Mean in C Programming?
The format specifier %4d is used in C programming to print a value using the %d format conversion with a minimum field width of 4 characters. This means that if the value is less than 4 characters wide, printf() will automatically insert extra blanks to align the output. For example, if you use %4d to print the integer 5, it would be printed as ” 5″, with three blank spaces before the number.
Using %4d in C Programming
We use %4d in C programming to format and align the output to 4 digits. This is useful when printing out numbers that need to be presented in a specific way. For example, if you wanted to print out a number such as 12345678, you culd use %4d to make it look like this: 1234 5678. By using %4d, the number is aligned in a neat and organized manner. This ensures that the numbers are easy to read and understand by both humans and computers. Not only does this make the output more readable, it also makes it easier for programs to process the data efficiently.
Understanding the Meaning of %2d in C
%2d in C is a printf format specifier which is used to print a signed integer in decimal notation. It is composed of two parts: the percent sign ‘%’ and the number ‘2d’, which together indicate that the output should be printed as a decimal number with a minimum width of two characters. If the number to be printed is less than two digits, then it will be padded with spaces on the left so that its total width is at least two characters.
Using Integers in C
Yes, the %d specifier is used for decimal integers in C. It assumes that the integer is in base 10, meaning that it will only recognize numbers from 0-9. If you want your integer to be interpreted as a different base (e.g. octal or hexadecimal), you should use the %o or %x specifiers respectively.
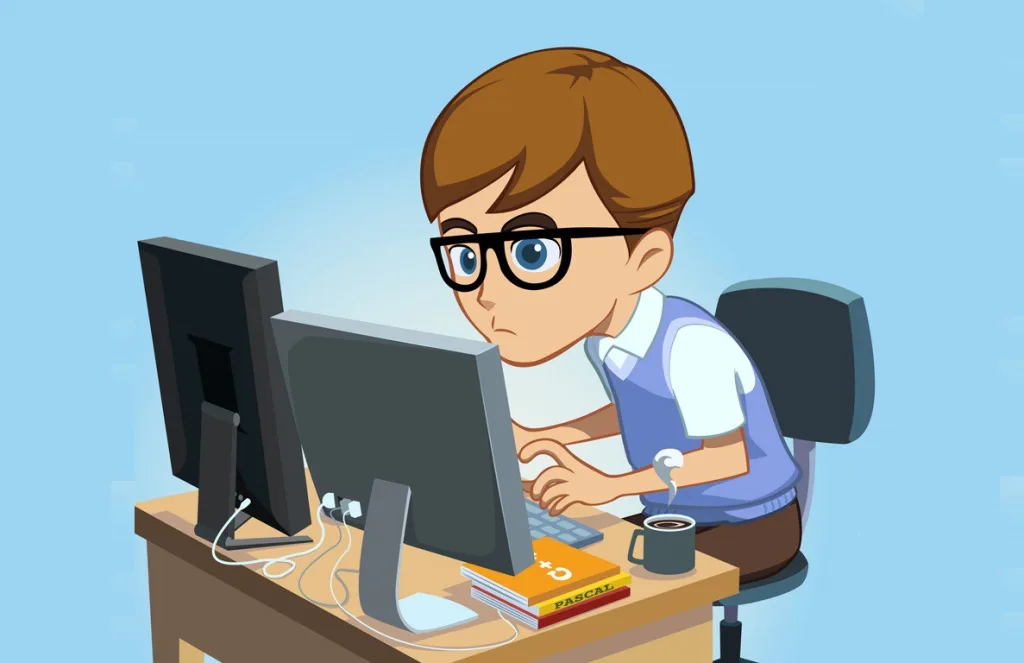
Using %d for Integers
We use the %d specifier in the printf statement to print integer values. It is used to represent signed decimal integers, which means it can represent both positive and negative numbers. The %d specifier is usually used when we need to print values that are of the int data type. Additionally, if we try to print a value of a different data type using %d, it will result in an unpredictable output or garbage value.
Printing Data Types in C
In C, you can print data type values by usig the printf() function. This function takes two parameters. The first parameter is the format specifier – this is a character that tells the computer what type of data to expect when printing. The second parameter is the value of the data type you want to print. For example, if you want to print an integer, you would use the %d format specifier and then provide an integer value as the second parameter. Similarly, if you want to print a float value, you would use %f as your format specifier and provide a float value as your second parameter. By using these format specifiers and providing appropriate values, you can easily print any data type values in C.
Conclusion
In conclusion, size_t variables can be printed in C using the “%zu” format. This is because size_t is an unsigned integer type used to store the maximum size of theoretically possible arrays. It is important to note that on a 32-bit system size_t will take 32 bits and on a 64-bit one 64 bits. Furthermore, the printf() method can be used to print the value of any type on the console screen by replacing the X with the appropriate type int. With this information it should now be easier for you to print size_t variables in C.