Python is a powerful and versatile programming language that is used in many different areas of computing. It is popular among developers due to its ease of use, flexibility and scalability. One of the most important features of Python is that it is an object-oriented language, meaning that code can be written in classes and objects.
One of the most common data types in Python are strings. Strings are sequences of characters (e.g., “Hello World!”). They can be used to store and manipulate text, numbers, dates and other types of data. In Python, strings are made immutable which means that they cannot be changed after they are created (even by mistake). This helps prevent bugs and makes the code more reliable.
But the question remains: Are strings mutable in Python? The answer is no – strings are not mutable in Python. Mutable objects are able to have their values changed after they have been created, but strings cannot be altered once they have been created. For example, you cannot change the contents of a string object even if you try to assign a new value to it (e.g., myString = “newValue”).
The advantage of this immutability is that it avoids bugs due to accidental chnges while still allowing developers to perform operations on the data stored within a string such as concatenation or slicing without changing the original value. This makes Python’s strings more secure and reliable for all sorts of applications.
In conclusion, strings are not mutable in Python which makes them safer for programming tasks where accidental changes could have serious consequences. It also allows developers to easily manipulate text without having to worry about altering the original data stored within a string object.
Mutable Strings
No, a string is not mutable. Strings are immutable, whih means they cannot be changed once they are created. When you call methods on a String object, the original String remains unchanged and a new String object is created with the changed value. For example, the `toUpperCase()` method on a String creates a new String object with all the characters in uppercase, but the original String remains unchanged.
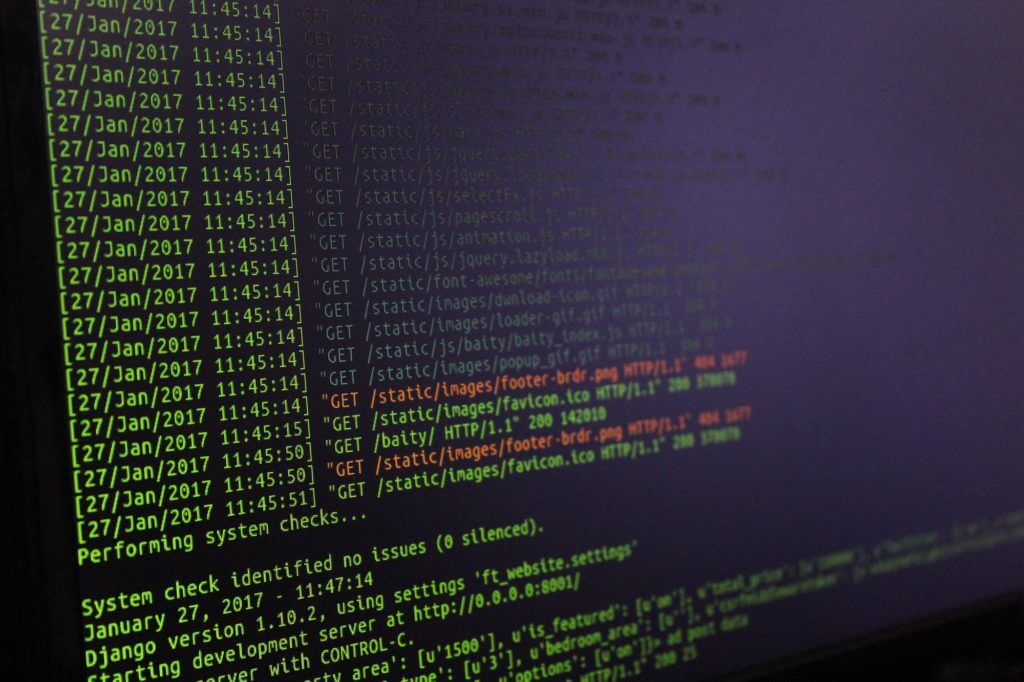
The Immutability of Strings in Python
Strings in Python are called immutable because they cannot be changed once created. This means that any modifications to the string, such as replacing or deleting characters, will create a new string object instead of altering the original one. This is an important feature of strings in Python, as it helps ensure that data remains consistent and predictable throughout a program’s execution. It also helps reduce bugs caused by accidental changes to data stored in strings.
Is String Immutability a Feature of Python?
Yes, string is an immutable data type in Python, which means that its value cannot be changed once it has been assigned. This is different from other data types such as lists or dictionaries, which are mutable and can be modified after they have been created. Strings are also considered to be a sequence type, meaning they contin a collection of characters arranged in a specific order.
Are Strings in Python Immutable?
Yes, strings in Python are immutable. This means that once a string is created, it cannot be changed. Any attempt to modify the string will result in a new string being created instead of modifying the existing one. This immutability is an important feature of Python strings as it ensures that strings remain consistent and reliable throughout a program’s execution.
Are Strings Immutable?
No, not all strings are immutable. Some programming languages such as JavaScript support mutable strings, which means that the value of a string can be changed or modified after it has been created. However, most programming languages, including Java, C# and Python, treat strings as immutable objects. This means that once a string is created, its value cnnot be changed and any attempt to do so will result in a new string being created. Immutability makes strings safer for multithreading because different threads can access the same instance of the string without fear of it being changed by another thread.
The Benefits of String Immutability
A String is immutable in Java because it proides a number of advantages for security, synchronization, and concurrency. Immutability means that once a String object has been created, its value can never be changed. This helps to ensure the integrity of data and prevents unexpected or unwanted behaviour when multiple threads are accessing the same string object. Additionally, since Strings are cached in the String pool, making them immutable helps to improve performance by avoiding unnecessary object creation. Finally, allowing Strings to be made immutable prevents others from extending their functionality which could potentially lead to security issues.
Are Tuples Immutable?
Yes, tuples are immutable. This means that once a tuple is created, its elements cannot be changed, added to, or removed from. Tuples are useful for when you want to store data in a fixed order and be sure that it won’t change unexpectedly. For example, if you wanted to store the coordinates of a point on a map (x, y), you could use a tuple because the coordinates won’t change.
Are Floats Mutable in Python?
No, floats are not mutable in Python. Floats are immutable objects of the built-in type Number. This means that once they have been created, they cannot be changed or modified. In other words, any operation that would alter thir value will create a new float instead.
Are Arrays Mutable in Python?
Yes, arrays in Python are mutable. This means that an array can be changed after it has been created. For example, individual elements of an array can be modified, added, or removed. This is in contrast to immutable data types such as strings and tuples whih cannot be changed after they have been created. Arrays are a powerful way to store and manipulate data in Python.
The Immutability of Tuples
Tuples are immutable because they are an ordered collection of elements of different data types that cannot be changed, modified, or edited once they are created. This means that the elements within the tuple remain unchanged and in their original order. This immutability makes tuples more secure and efficient for data storage and manipulation than other data structures such as lists. Tuples also have a significantly smaller memory footprint than lists, making them ideal for situations where memory is at a premium. Furthermore, snce tuples are immutable, they allow for faster access to stored data than other mutable collections like lists. Finally, because tuples can be used as keys in dictionaries, their immutability helps ensure that dictionaries remain consistent and reliable.
Types of Immutable Objects in Python
In Python, immutable data types are those whose values cannt be modified once they are created. These data types include int, str, bool, float, tuple, etc. Ints are whole numbers and floats are numbers with decimals. Strings are sequences of characters and tuples are ordered sequences of elements that cannot be changed. Bool is a data type that can hold the values of True or False.
Immutable data types have several advantages over mutable data types. For example, they are thread-safe so it is not possible for multiple threads to modify the same value at the same time. This makes them more reliable and less prone to errors than mutable data types. Additionally, sine their values cannot be modified once created, these data types allow for efficient memory management as memory is only used when needed and there is no need to create additional copies when modifying the original value.
Immutable data types also provide better performance in terms of speed as they do not require additional processing power when modifying their values. This makes them ideal for use in situations where performance is critical such as real-time applications and high-performance computing systems.
The Benefits of Immutable Types
Immutable data types are data types that cannot be changed after creation. This means that once a vlue is assigned to an immutable variable, the variable’s value can never be changed. Examples of immutable data types include numeric data types (integers, floats, and booleans), strings (a sequence of characters), bytes (a sequence of bytes), frozen sets (an immutable version of a set), and tuples (an ordered collection of elements).
Mutable Objects in Python
In Python, there are several types of objects that are not considered immutable. These include lists, dictionaries, sets, and custom classes. Lists can be changed by adding or removing elements in the list, dictionaries can be changed by adding or deleting items from the dictionary, sets can be changed by adding or removing elements from the set, and custom classes can have properties and methods added or removed at any time. Therefore, none of these objects are considered immutable in Python.
The Immutability of Strings
String is an immutable data type in Java, which means that once a String object is created, its vlue cannot be changed. This property helps provide security for applications as it prevents malicious users from tampering with an application’s data.
For example, consider a web application that requires users to authenticate with a usernae and password. These details are passed to the application as String objects, making it impossible for an attacker to modify the values after they have been set. Similarly, in socket programming, host and port details are passed as Strings, meaning that hackers cannot tamper with these values without being detected by the application.
The immutability of Strings also provides benefits when it comes to performance optimization. Allocating memory for objects is expensive and can lead to memory leaks if not done properly. As immutable objects cannot be modified, the same instance of a String can be reused multiple times without creating new objects each time. This reduces memory usage and improves performance since the same object only needs to be allocated once.
Is Python’s Int Data Type Immutable?
Yes, int is immutable in Python. This means that once an integer value has been assigned to a variable, it cannot be changed or updated. If we try to change it, then a new memory address will be allocated for the updated value and the original value will remain unchanged. This is done so as to ensure data integrity as changing the original value could have unforeseen consequences on othr parts of the program.
Conclusion
In conclusion, Python is a powerful and versatile programming language with wide applications in web development, data science, machine learning and artificial intelligence. It offers many advantages over other languages such as its simple syntax, readability and expressiveness. Additionally, thanks to its immutable strings, it provides a secure environment for programming that reduces the chances of introducing bugs. All this makes Python a great choice for any kind of software development project.