Abstract Data Types (ADTs) are an essential concept in computer science and programming. They provide a way to encapsulate data and define operations on that data, allowing for efficient and organized manipulation.
At its core, an ADT is a combination of a data structure and the operations that can be performed on that structure. The data structure represents the organization and storage of the data, while the operations define the actions that can be taken on the data.
Let’s take a closer look at these two components:
Data Structure:
The data structure of an ADT determines how the data is stored and organized. It specifies the format and layout of the data, allowing for efficient access and manipulation. Different ADTs can have different data structures based on the specific requirements of the data and operations.
For example, a common ADT is the “List”. A list can be implemented using an array or a linked list as its underlying data structure. Arrays provide constant time access to elements but have a fixed size, while linked lists allow for dynamic resizing but have slower access times.
Other popular data structures used in ADTs include stacks, queues, trees, and graphs. Each data structure has its own advantages and disadvantages, making it suitable for different scenarios.
Operations:
The operations of an ADT define the actions that can be performed on the data. They specify how the data can be modified, accessed, and manipulated. The operations are the interface through which users interact with the ADT.
Common operations performed on ADTs include insertion, deletion, searching, sorting, and traversal. These operations are designed to work specifically with the data structure of the ADT, ensuring efficient and accurate results.
For instance, in a list ADT, operations like insert, delete, and search allow for adding, removing, and finding elements within the list. These operations can be implemented differently depending on the data structure used.
The combination of the data structure and operations in an ADT provides a powerful tool for organizing and manipulating data. By encapsulating the data and defining specific operations, ADTs enable modularity and reusability in programming.
In programming languages like Java, ADTs are typically implemented using classes or interfaces. The class defines the data structure and operations, while the interface specifies the contract for the operations without providig implementation details.
By using ADTs, programmers can focus on the high-level design of their programs, abstracting away the complexities of the underlying data structures. This allows for better code organization, maintainability, and scalability.
Abstract Data Types (ADTs) combine a data structure and operations to provide a powerful tool for organizing and manipulating data. The data structure determines how the data is stored and organized, while the operations define the actions that can be performed on the data. By encapsulating data and providing a well-defined interface, ADTs enable modularity and reusability in programming, making them a fundamental concept in computer science and programming.
What Are The Two Main Elements Of ADT?
The two main elements of an Abstract Data Type (ADT) are the Data and the Operations.
1. Data: This element describes the structure and organization of the data used in the ADT. It specifies what kind of information can be stored and how it is stored. The data can be a simple value, such as a number or a string, or it can be a more complex structure, like a list or a tree. The data element defines the properties and characteristics of the information that the ADT can handle.
2. Operations: This element defines the valid operations or actions that can be performed on the data stored in the ADT. These operations determine how the data can be manipulated, accessed, or modified. Examples of operations include retrieving a value, adding or removing elements, sorting the data, or performing calculations. The operations define the interface of the ADT, which specifies what actions can be performed and how they can be invoked.
The data element describes the structure of the information stored in the ADT, while the operations element defines the valid actions that can be performed on that data. Together, thee two elements form the foundation of an ADT, providing a clear and consistent way to work with the data and perform useful operations on it.
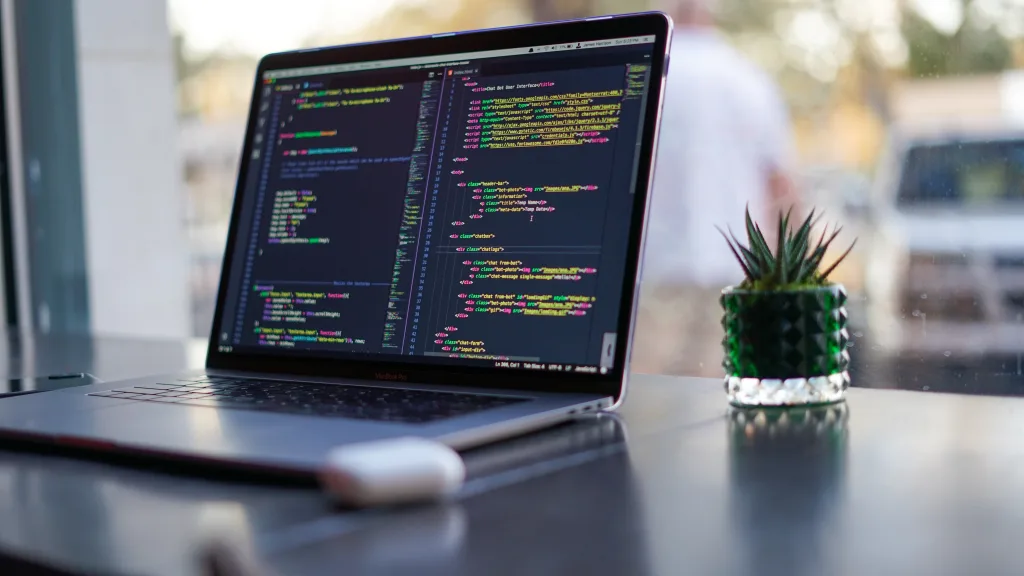
What ADT Contains?
An Abstract Data Type (ADT) contains two main components:
1. Specification of Possible Values: This component defines the set of values that the ADT can take. It describes the characteristics and constraints of these values, such as their data types, range, and any specific rules or conditions they must adhere to. For example, an ADT representing a Stack may specify that the possible values are a collection of elements that can only be accessed in a last-in-first-out (LIFO) manner.
2. Specification of Operations: This component outlines the operations or functions that can be performed on the values of the ADT. It describes the inputs required for each operation, the resulting outputs, and any side effects or changes that occur when the operation is executed. These operations define how the values can be manipulated or accessed. For instance, a Stack ADT may have operations like “push” to add an element to the top of the stack, “pop” to remove and return the top element, and “isEmpty” to check if the stack is empty.
An ADT comprises the specification of the possible values that can be held by the data type and the operations that can be performed on those values. It proides an abstraction of the underlying implementation, allowing users to utilize the data type without requiring knowledge of its internal details.
What Is Called ADT?
ADT, short for Abstract Data Type, is a fancy term used in computer science to describe a mathematical model for data types. Now, I know what you’re thinking – “matematical model? Sounds complicated!” Well, fear not, my friend, because I’m here to break it down for you in the simplest way possible.
So, imagine you have a bunch of data – numbers, strings, objects, you name it. Now, an abstract data type is all about how you can interact with that data. It defines the behavior, or semantics, of the data from the perspective of a user. In other words, it’s like a set of rules that tells you what you can do with the data and how those operations will behave.
Now, let’s talk about the possible values of an abstract data type. Basically, it’s just the dfferent kinds of data that you can have. For example, you can have a data type that represents a person’s name, or one that represents a list of numbers.
But it’s not just about the values themselves – it’s also about the operations youcan perform on those values. This is where things get interesting. An abstract data type defines a set of operations that you can do with the data. These operations can include things like adding two values together, finding the length of a list, or even sorting a set of values.
Now, here’s the cool part – the behavior of these operations is also defined by the abstract data type. So, for example, if you have a data type that represents a list, the abstract data type will tell you how the operations like adding or removing elements from the list will behave.
A abstract data type is like a blueprint for how you can interact with your data. It defines the possible values, the operations you can perform on those values, and how those operations behave. It’s a way to organize and structure your data so that you can work with it in a meaningful and efficient way.
What Is An ADT In Java?
An Abstract Data Type (ADT) in Java is a fancy term for a special kind of data type that we can create using classes or interfaces. It’s like a custom-made data type that we can define ourselves, with its own set of values and operations.
Now, you might be wondering, what’s the point of creating our own data types when Java already has built-in ones like int, double, and String? Well, the thing is, sometimes those built-in types just don’t cut it for our specific needs. We might want a data type that represents something more complex, like a list or a stack.
That’s where ADTs come in. They allow us to define our own data types that have values and operations that aren’t already defined in the Java language. It’s like creating a blueprint for a new type of object.
To implement an ADT in Java, we can use a class or an interface. We define the values that the ADT can hold, as well as the operations that can be performed on those values. For example, if we were creating an ADT for a stack, we might define operations like push, pop, and isEmpty.
The beauty of ADTs is that they provide a way for us to organize and abstract our code. They allow us to encapsulate complex data structures and algorithms into easy-to-use objects. Plus, they make our code more modular and reusable.
So, the next time you find yourself needing a data type that doesn’t quite fit into the built-in ones, consder creating your own ADT. It’s a powerful tool that Java provides for us to create custom data types that perfectly suit our needs.
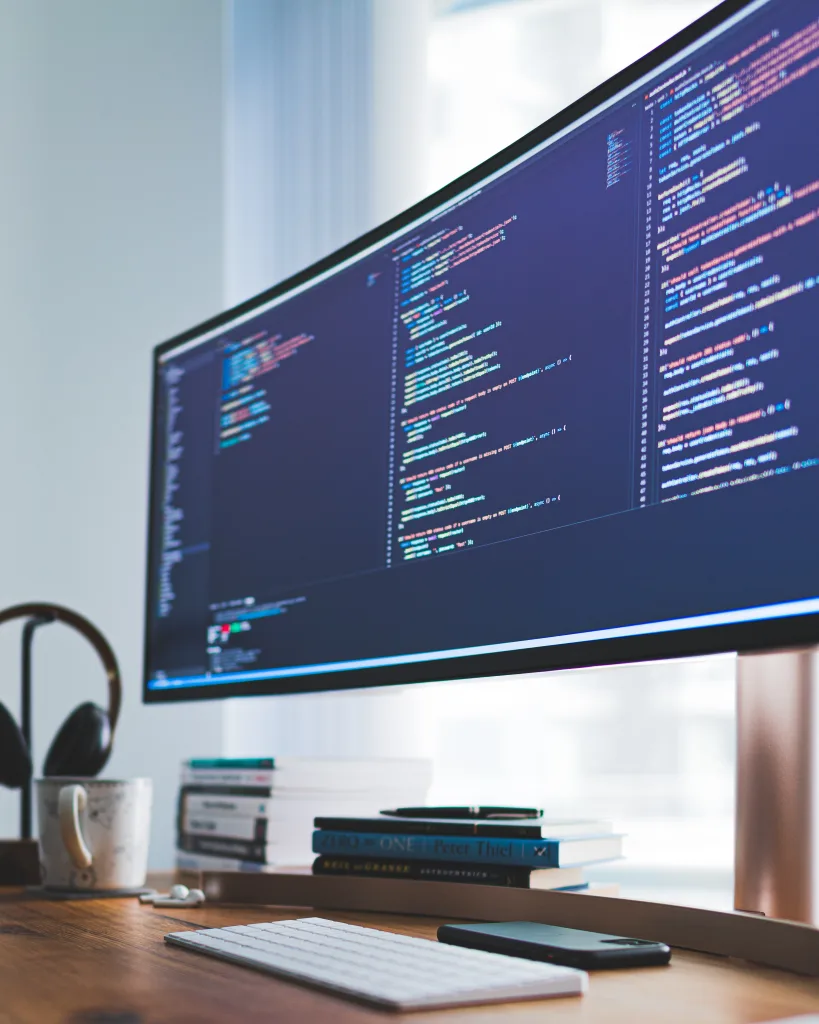
Conclusion
An Abstract Data Type (ADT) is a fascinating combination of both structure and functionality. It encompasses the values and operations that are not inherently defined in a programming language itself. It is like a hidden gem, waiting to be unlocked and utilized to its fullest potential.
Imagine an ADT as a mathematical model for data types, where its behavior is defined from the perspective of a user. It is all about the possile values that can be stored and the operations that can be performed on those values. And these operations have their own unique inputs, outputs, and effects.
When it comes to implementing an ADT in Java, it can be done through a class or an interface. This provides a way to structure the data and define the behavior of the operations associated with the ADT. It’s like creating a blueprint for how the data should be organized and manipulated.
But an ADT is more than just a technical concept. It is a powerful tool that allows us to solve complex problems and create efficient algorithms. It enables us to think abstractly and tackle real-world challenges with elegance and simplicity.
So, next time you encounter an ADT, remember that it’s not just a collection of values and operations. It’s a world of possibilities, waiting to be explored and harnessed. Embrace the beauty of abstraction and let the ADT guide you towards innovative solutions.