Parallelization is a crucial concept in the field of computer programming, particularly when it comes to optimizing the performance of software. By utilizing parallel programming techniques, developers can significantly reduce the time it takes to solve complex problems by dividing the workload among multiple resources.
At its core, parallelization involves the design and implementation of computer programs or systems that can process data in parallel. Traditionally, programs have executed tasks sequentially, one after the other, which can lead to inefficient use of resources and longer processing times. However, with parallel programming, developers can harness the power of multiple cores or processors to perform tasks simultaneously, thereby accelerating the overall execution speed.
One common strategy for parallelization is to split iterations of a loop into separate computations and distribute them among parallel tasks. This approach allows multiple tasks to work on different parts of the problem concurrently, effectively dividing the workload and reducing the time required for completion.
Parallel programming in C is particularly important for enhancing software performance. C is a widely used programming language known for its efficiency and low-level control over hardware resources. By leveraging parallel programming techniques in C, developers can take full advantage of the available hardware, such as multicore processors, to achieve higher levels of performance.
There are various parallel programming models and frameworks available to facilitate the implementation of parallelization in C. These include OpenMP, MPI (Message Passing Interface), and CUDA (Compute Unified Device Architecture), among others. Each of these frameworks provides different levels of abstraction and functionality, allowing developers to choose the most suitable approach for their specific needs.
In addition to improving performance, parallelization also offers other benefits. For instance, it can enhance the scalability of software, allowing it to efficiently handle larger datasets or more complex computations. Moreover, parallel programming can lead to better resource utilization, as it enables the efficient usage of available CPU cores or processors.
However, it’s important to note that parallelization is not a one-size-fits-all solution. The effectiveness of parallel programming depends on various factors, including the nature of the problem being solved, the available hardware resources, and the programming model or framework being used. It requires careful consideration and analysis to determine the optimal level of parallelism and design an efficient parallel program.
Parallelization is a fundamental concept in computer programming that involves dividing the workload among multiple resources to solve problems more efficiently. Parallel programming in C, using frameworks such as OpenMP or MPI, can significantly enhance the performance of software by leveraging the power of multiple cores or processors. However, successful parallelization requires careful analysis and design to ensure optimal performance and resource utilization.
What Is The Meaning Of Parallelization?
Parallelization refers to the process of dividing a task or problem into smaller, more manageable parts that can be executed simultaneously. It involves breaking down a task or problem into independent components, which can then be processed concurrently, in parallel. This approach allows for faster and more efficient execution, as multiple parts of the task can be executed simultaneously by different processors or threads.
To understand the meaning of parallelization, it can be helpful to draw parallels with a familiar scenario. Imagine you have a large pile of laundry that needs to be washed, dried, and folded. If you were to tackle this task sequentially, you would first wash all the clothes, then dry them, and finally fold them. This would require a significant amount of time and effort, as each step would need to be completed one after the other.
However, if you were to parallelize this task, you could divide it into smaller sub-tasks that can be performed concurrently. For example, one person could start washing the clothes while another person simultaneously begins drying the already washed clothes. And yet another person could start folding the dry clothes as they become available. By dividing the task and executing the sub-tasks in parallel, you can significantly reduce the overall time required to complete the laundry.
Parallelization is the process of breaking down a task or problem into smaller, independent parts that can be executed simultaneously. This approach allows for faster and more efficient execution by leveraging the power of parallel processing.
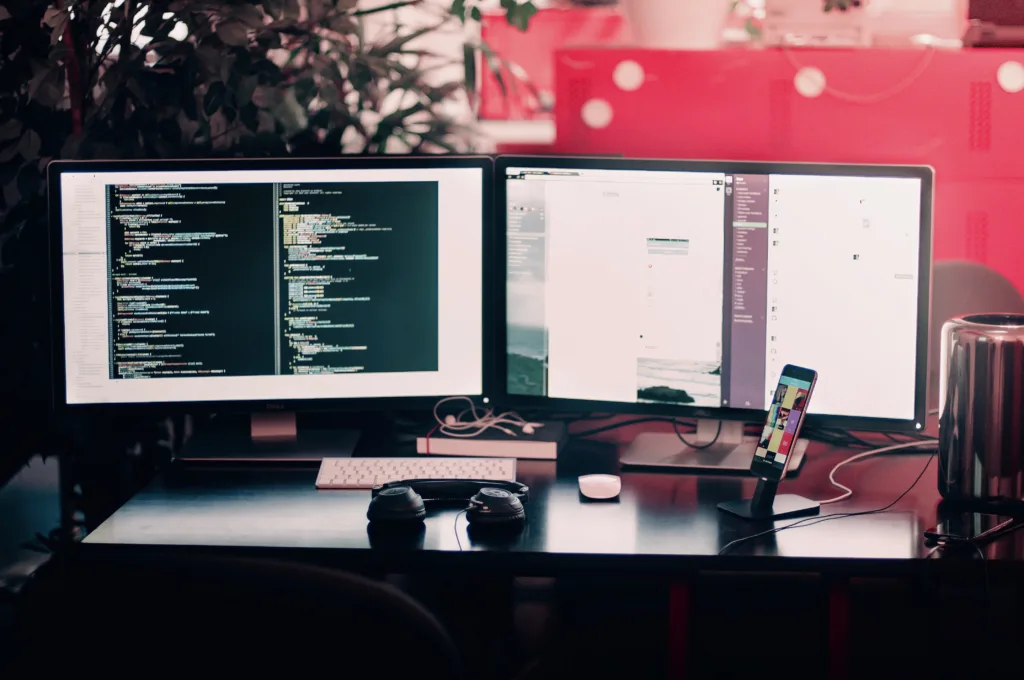
What Is Parallelization Programming?
Parallel programming, also known as parallelization programming, refers to the technique of breaking down a computational problem into smaller tasks that can be executed simultaneously on multiple processors or processor cores. This approach aims to improve the performance and efficiency of software by leveraging the capabilities of modern computer systems.
In traditional sequential programming, a problem is solved by executing a series of instructions in a single thread, one after another. This can lead to slower execution times and limited utilization of available resources. Parallel programming, on the other hand, allows for the distribution of work across multiple processors, enabling multiple tasks to be executed concurrently.
The main goal of parallel programming is to reduce the overall execution time of a program by dividing the workload among multiple processors, which can work on different parts of the problem simultaneously. By doing so, parallel programming can significantly increase the speed and efficiency of computationally intensive tasks.
There are different approaches to parallel programming, including shared memory and message passing. In shared memory parallel programming, multiple threads or processes share a common memory space and can directly read from or write to shared variables. This approach requires careful synchronization to avoid conflicts and ensure data consistency.
Message passing parallel programming, on the other hand, involves passing messages between different processes or threads. Each process has its own memory space, and communication is achieved through passing messages, which can be more flexible and scalable in certain scenarios.
Benefits of parallel programming include:
1. Improved performance: By distributing the workload among multiple processors, parallel programming can significantly reduce the overall execution time of a program.
2. Increased scalability: Parallel programming allows for the efficient utilization of resources in modern computer systems, enabling software to scale and handle larger and more complex problems.
3. Better resource utilization: By leveraging multiple processors, parallel programming maximizes the utilization of available computational resources, leading to improved efficiency.
4. Enhanced responsiveness: Parallel programming can improve the responsiveness of software by allowing certain tasks to be executed concurrently, ensuring a smooth user experience.
5. Future-proofing: As computer systems continue to evolve with more processors and cores, parallel programming ensures that software can fully leverage these advancements and maintain optimal performance.
Parallel programming is the technique of dividing a computational problem into smaller tasks and executing them simultaneously on multiple processors or cores. By doing so, parallel programming improves performance, scalability, resource utilization, responsiveness, and future-proofing of software.
What Is Parallelization In Parallel Computing?
Parallelization in parallel computing refers to the technique of designing and implementing computer programs or systems to process data simultaneously, or in parallel, instead of sequentially. In traditional serial computing, tasks are executed one after the other, where the output of one task serves as the input for the next. However, in parallel computing, multiple tasks are executed simultaneously, allowing for faster and more efficient data processing.
Parallelization is achieved by dividing a large problem into smaller sub-problems, which can be solved independently and concurrently. These sub-problems are then assigned to different processing units, such as multiple processors or cores, within a computer system. Each processing unit works on its assigned sub-problem simultaneously, utilizing its computational resources independently. Once all the sub-problems are solved, the results are combined to obtain the final solution.
The main advantage of parallelization is its ability to significantly reduce the overall processing time for computationally intensive tasks. By distributing the workload across multiple processing units, parallel computing enables tasks to be completed in parallel, leading to faster execution and improved performance.
Parallelization can be achieved at different levels of granularity, ranging from fine-grained parallelism to coarse-grained parallelism. Fine-grained parallelism involves breaking down a task into smaller parts that can be executed simultaneously, while coarse-grained parallelism involves dividing the task into larger chunks that can be processed concurrently.
Parallelization can be applied to various types of computations, such as numerical simulations, data analysis, image processing, and machine learning, among others. It requires careful consideration of the problem’s characteristics, the available hardware resources, and the programming model or framework being used.
Parallelization in parallel computing is the technique of dividing a large problem into smaller sub-problems and processing them simultaneously on multiple processing units. It enables faster and more efficient data processing by harnessing the power of parallel execution.
What Is Parallelization Strategies?
Parallelization strategies refer to the techniques and approaches used to divide a task or computation into smaller parts that can be executed simultaneously by multiple processors or threads. The goal is to improve overall performance and reduce the time required to complete the task. Parallelization is particularly effective in scenarios where a computation can be broken down into independent or loosely coupled subtasks.
There are several parallelization strategies that can be employed depending on the nature of the problem and the available hardware resources. Some common strategies include:
1. Task parallelism: In this strategy, different tasks or subtasks are assigned to different processors or threads. Each processor works on its assigned task independently and concurrently. Task parallelism is suitable for problems where the subtasks are independent and do not require much communication or synchronization between them.
2. Data parallelism: This strategy involves dividing the data into smaller chunks and assigning each chunk to a different processor or thread. Each processor then operates on its assigned data independently and concurrently. Data parallelism is useful when the same operation needs to be performed on different parts of the data simultaneously, such as in image or video processing.
3. Loop parallelism: Loop parallelism focuses on parallelizing iterations of a loop. Each iteration is split into separate computations, and these computations are distributed among parallel tasks. Loop parallelism is commonly used in scientific and numerical computations where loops are a prevalent construct.
4. Pipeline parallelism: In pipeline parallelism, the overall computation is divided into a series of stages, and each stage is executed concurrently by different processors or threads. Each stage processes a portion of the data and passes it to the next stage. Pipeline parallelism is suitable for problems with a sequential dependency between stages, such as in video or audio processing.
5. Hybrid parallelism: This strategy combines multiple parallelization techniques to achieve better performance. For example, a combination of task parallelism and data parallelism can be used to divide both tasks and data among parallel resources.
It’s important to note that the choice of parallelization strategy depends on the problem at hand, the hardware architecture, and the scalability requirements. It requires careful analysis and consideration to determine the most suitable strategy for a given situation.
Conclusion
Parallelization is a crucial concept in computer programming that involves the use of multiple resources to solve a problem in a shorter amount of time. By dividing the work and assigning it to separate tasks or threads, parallel programming allows for simultaneous processing of data, resulting in improved performance and efficiency.
In the context of C programming, parallelization plays a significant role in enhancing the overall speed and effectiveness of software. By utilizing parallel programming techniques, developers can effectively distribute the computational workload across multiple processors or cores, enabling the program to execute multiple tasks simultaneously.
The primary goal of parallelization is to reduce the overall execution time and maximize resource utilization. By breaking down complex computations or tasks into smaller, manageable chunks, parallel programming enables efficient utilization of available resources, resulting in faster and more efficient processing.
Parallelization strategies can vary depending on the specific problem or program being addressed. One common strategy involves dividing iterations of an outer loop into separate computations and distributing these computations among parallel tasks. This approach allows for efficient utilization of resources by assigning independent tasks to separate processing units.
Parallelization is a powerful technique that allows for the efficient utilization of resources and improved performance in computer programming. By leveraging multiple processors or cores, developers can significantly enhance the speed and efficiency of their software, ultimately leading to improved user experience and increased productivity.