In the world of coding, a string is a fundamental concept that plays a crucial role in manipulating and storing text. It is a sequence of characters, such as letters, numbers, and symbols, that are grouped together to form a meaningful piece of data. Strings are widely used in programming languages like Java, C++, Python, and many others.
To understand the concept of a string, let’s take a closer look at its characteristics and how it is used in coding.
Firstly, a string is represented by a series of characters enclosed within double quotes. For example, “Hello world!” is a string that consists of the characters ‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘ ‘, ‘w’, ‘o’, ‘r’, ‘l’, ‘d’, and ‘!’. The double quotes serve as a delimiter, indicating that the enclosed characters are to be treated as a single entity.
Strings are incredibly versatile and can be used in various ways. They are commonly used to store and manipulate textual data, such as user inputs, messages, file names, and more. For instance, a program that prompts the user to enter their name would store that input as a string.
One of the key features of strings is the ability to concatenate or combine them. This operation allows programmers to join multiple strings together, creating a new string that contains the combined text. Concatenation is achieved using the ‘+’ operator. For example, if we have two strings “Hello” and “world!”, we can concatenate them to form the string “Hello world!”.
In addition to concatenation, strings support various operations and methods for manipulating and analyzing their content. These include finding the length of a string, extracting substrings, converting case (lowercase or uppercase), searching for specific characters or patterns, and replacing characters or portions of the string.
Another important aspect of strings is their immutability. Once a string is created, its content cannot be changed. Instead, any operation that modifies a string actually creates a new string with the desired changes. This immutability ensures that strings remain consistent and avoids unexpected modifications.
Furthermore, strings often play a crucial role in input/output operations. They are used to display information to the user and receive inputs from them. For instance, a program may use strings to show error messages, display results, or ask for user input through text-based interfaces.
It is worth mentioning that strings are not limited to just letters and numbers. They can also include special characters, whitespace, and even control characters. Control characters are non-printable characters used to perform specific actions, such as moving the cursor or changing the display color.
Strings are a fundamental concept in coding that allows programmers to work with and manipulate textual data. They are used to store, combine, and analyze text, making them an essential tool in various programming languages. Understanding the concept of strings is crucial for anyone looking to dive into the world of coding and develop software that deals with textual information.
What Is A String In Coding Example?
A string in coding refers to a sequence of characters, such as letters, numbers, or symbols, that are treated as a single data type. It is represented by placing the characters within double quotes in Java. For instance, the string “hello” consists of the characters ‘h’, ‘e’, ‘l’, ‘l’, and ‘o’ in that order.
Strings are commonly used in programming to store and manipulate textual data. They allow us to work with words, sentences, or any other form of text. Strings are immutable in Java, which means that once a string is created, its value cannot be changed. However, we can perform various operations on strings, such as concatenation, comparison, and extraction of substrings.
Here is an example of a string in Java:
String name = “John Doe”;
In this case, the variable “name” is assigned the string value “John Doe”. We can now use this string to perform different operations or manipulate it as needed.
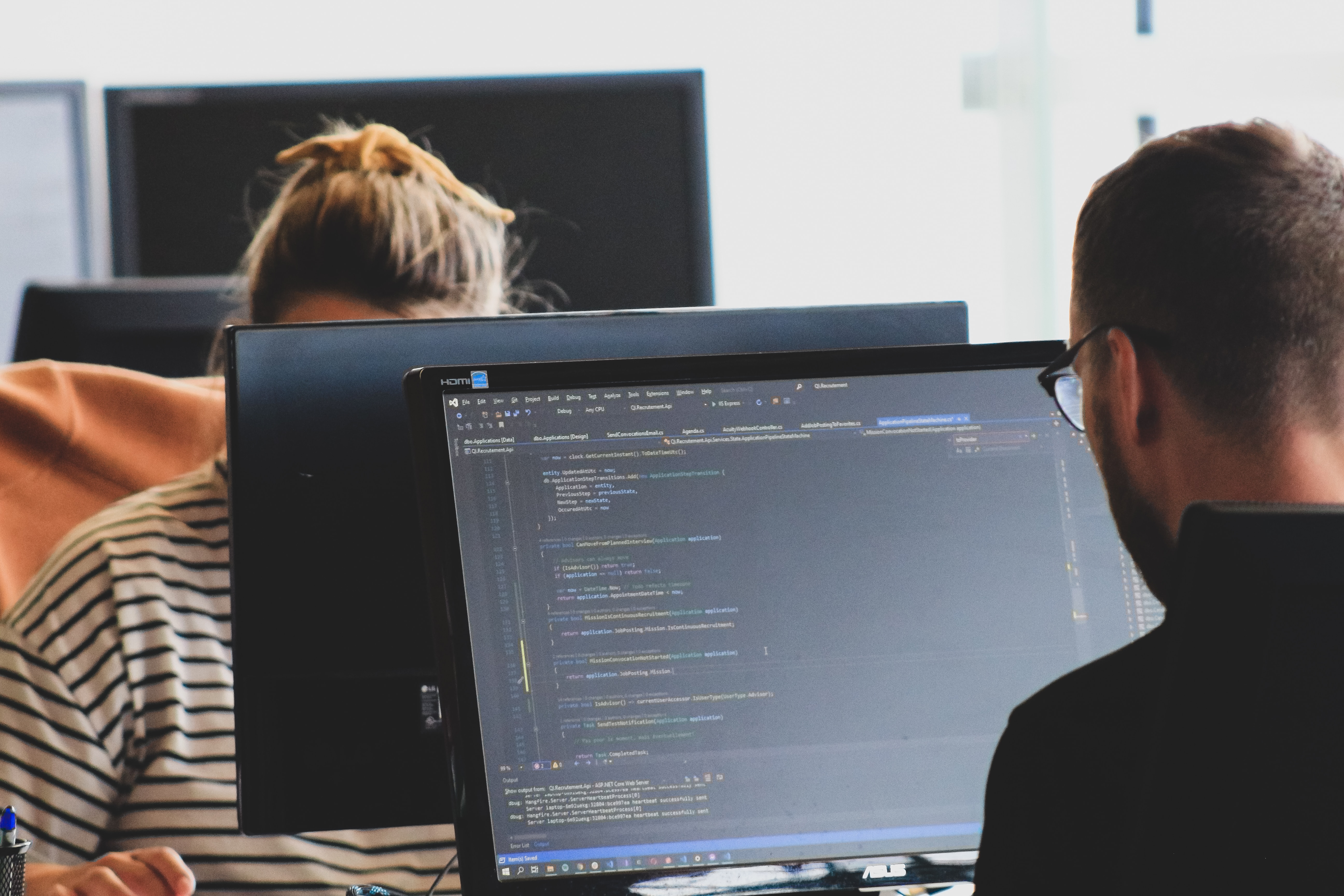
What Is A String In Simple Terms?
A string, in simple terms, is a type of thin rope that is made by twisting together multiple threads. It is commonly used for tying things together or for securing parcels. Strings are usually flexible and can be easily manipulated to form knots or loops. They come in various materials such as cotton, nylon, or polyester, and can vary in length, thickness, and strength depending on their intended use. Strings are versatile and widely used in various industries and everyday tasks, including crafts, packaging, and even musical instruments.
To summarize, a string is a thin and flexible rope-like object made by twisting threads together. It is primarily used for tying and securing objects.
How Do You Write A String In Coding?
To write a string in coding, you can use the String data type, which is commonly used to represent text. There are several ways to create a string in different programming languages, but the most direct and common way is to enclose the text within double quotes.
Here’s a step-by-step guide on how to write a string in coding:
1. Start by declaring a variable of type String. This tells the compiler or interpreter that you want to store a sequence of characters.
2. Assign a value to the string variable by using the assignment operator (=) followed by the desired text enclosed in double quotes.
For example, in Java:
String greeting = “Hello world!”;
In Python:
Greeting = “Hello world!”
In C#:
String greeting = “Hello world!”;
In JavaScript:
Var greeting = “Hello world!”;
3. Make sure to use the appropriate syntax and conventions for the specific programming language you are using. For instance, some languages require a specific data type declaration (e.g., “string” in C#), while others use var or infer the data type automatically (e.g., JavaScript).
4. You can also concatenate strings by using the concatenation operator (+) or by using string interpolation if your language supports it.
For example, in Java:
String name = “John”;
String message = “Hello, ” + name + “!”;
In Python:
Name = “John”
Message = f”Hello, {name}!”
In C#:
String name = “John”;
String message = $”Hello, {name}!”;
In JavaScript:
Var name = “John”;
Var message = `Hello, ${name}!`;
Remember that strings are enclosed in quotes, and you can use any combination of letters, numbers, special characters, or even emojis within the quotes.
By following these steps, you can easily write a string in coding and incorporate it into your program or script to manipulate and display text-based data.
Why Do We Need String In Coding?
In coding, strings are essential for storing and manipulating textual data. They play a crucial role in a variety of applications and are used extensively in programming languages for several reasons:
1. Storing and representing text: Strings allow us to store and represent text-based data, such as names, addresses, sentences, paragraphs, and more. They provide a way to organize and manage textual information within a program.
2. User input and output: Strings are commonly used to handle user input and output. When users interact with a program, they often provide text-based information, such as their name or a message. Strings enable us to capture and process this input, and also to display output to users in a readable format.
3. Manipulating and formatting text: Strings provide a wide range of functions and methods to manipulate and format text. We can concatenate (join) strings together, split them into smaller parts, search for specific characters or words, replace portions of a string, convert case (uppercase or lowercase), and perform various other operations. These operations are crucial for data processing and analysis.
4. Data storage and retrieval: In databases and file systems, strings are used to store and retrieve textual data. They allow us to save and retrieve information, such as records or documents, with a specific format and structure. Strings are commonly employed in database queries, file operations, and data exchange between different systems.
5. Internationalization and localization: With the global nature of software development, strings are essential for supporting internationalization and localization. They enable the storage and display of text in different languages, character sets, and encoding formats. By using strings, developers can easily adapt their programs to different regions and cultures.
6. Error messages and logging: Strings are often used to generate error messages and log information in software applications. When an error occurs or when developers want to track program execution, they can use strings to provide meaningful feedback or record relevant details for debugging purposes.
Strings are fundamental in coding because they allow us to work with text-based data, manipulate and format text, handle user input and output, store and retrieve information, support internationalization, and log messages. They are a versatile tool that plays a vital role in many aspects of programming.
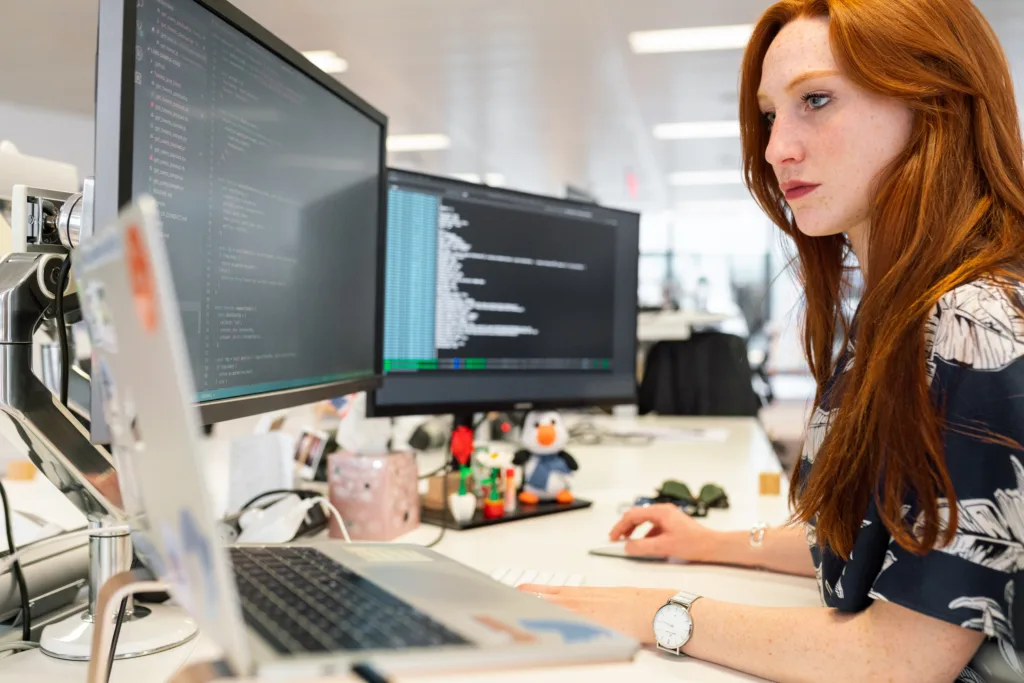
Conclusion
A string in Java is a sequence of characters enclosed in double quotes. It is a fundamental data type that is widely used in programming. Strings are versatile and can be used to represent text-based information such as words, sentences, or even entire paragraphs.
One of the key features of strings is their ability to store and manipulate textual data. They can be concatenated, or joined together, using the “+” operator, allowing for the construction of more complex strings. Additionally, strings can be compared using various methods to check for equality or perform sorting operations.
Strings are extensively used in user interfaces to display messages and prompts to the user. They are also commonly used for data input and output operations. For example, when reading or writing text files, strings are used to represent the content of the file.
In addition to their practical uses, strings also offer a range of methods and functions for manipulating and transforming text. These include methods for converting case, searching for specific characters or substrings, and replacing characters or substrings.
Furthermore, strings are immutable in Java, meaning that once a string is created, its value cannot be changed. This immutability ensures the integrity and consistency of the data stored in strings.
Strings play a crucial role in programming by providing a convenient and effective means of working with textual data. Their versatility, extensive functionality, and immutability make them a powerful tool for developers in a wide range of applications.