Recursion and iteration are two fundamental concepts in computer programming. Both methods are used to solve problems that require repetitive execution of a particular set of instructions. However, the question that arises is which of these two methods is faster and more efficient? In this article, we will explore the differences between recursion and iteration and determine whether recursion is actually faster than iteration.
Recursion is a technique in which a function calls itself during its execution. In other words, it is a process of solving a problem by breaking it down into smaller sub-problems of the same type. Recursion is widely used in computer science and is especially useful for solving problems that can be expressed recursively. Some examples of such problems include the Fibonacci sequence, the factorial of a number, and the traversal of tree structures.
Recursion is implemented using a call stack. When a function is called, the computer stores the current state of the function on the call stack. The function then executes its code until it reaches its base case, which is a condition that stops the recursion. At this point, the function returns the result to the previous function on the call stack, which continues its execution.
One of the main advantages of recursion is that it can be used to create concise and elegant code. Recursive code is usually shorter and easier to understand than its iterative counterpart. However, recursion is not always the most efficient method, and it can sometimes lead to stack overflow errors.
Iteration is a technique that involves executing a set of instructions repeatedly until a particular condition is met. It is a fundamental construct in most programming languages and is used for a wide range of purposes, such as traversing arrays, lists, and other data structures.
Iteration is implemented using loops, which can be either for, while, or do-while loops. The loop executes its code until a specified condition is met. The advantage of using iteration is that it is usually faster and more memory-efficient than recursion. This is because iteration does not require the computer to store the current state of the function on the call stack.
While recursion is a powerful and elegant technique, it is not always the fastest or most efficient method. Iteration is generally faster and more memory-efficient than recursion, especially for large data sets or problems that require many iterations. This is because iteration does not require the computer to store the current state of the function on the call stack.
That being said, recursion can be useful in crtain situations, such as when the problem can be expressed recursively or when the code needs to be concise and easy to understand. In such cases, recursion can lead to more elegant and readable code.
Both recursion and iteration are important techniques for solving problems in computer science. While recursion is useful in certain situations, it is not always the most efficient method. Iteration is generally faster and more memory-efficient than recursion, especially for large data sets or problems that require many iterations. Ultimately, the choice between recursion and iteration depends on the specific problem being solved and the requirements of the program.
How Much Faster Is Recursion Than Iteration?
Recursion is significantly faster than iteration in many cases. In fact, the recursive version of a function can run thousands of times faster than the iterative version, depending on the specific implementation and the problem being solved.
There are a few reasons why recursion can be faster than iteration. One is that recursive functions often have a simpler and more elegant structure than iterative functions, which can make them easier to optimize. Additionally, recursive functions can take advantage of the call stack, which allows them to quickly and efficiently store and access data.
To illustrate this point, let’s consider a specific example. Suppose we have two functions, one that uses recursion to calculate the factorial of a number, and one that uses iteration to do the same thing. If we compare the performance of thse two functions for large values of n, we would likely find that the recursive version runs much faster.
For instance, if we try to calculate the factorial of 1000 using the iterative function, it would take a very long time and may even cause a stack overflow error due to the large number of iterations needed. On the other hand, the recursive version can handle this calculation with ease and would likely run much faster as a result.
Recursion can be significantly faster than iteration in many cases due to its simpler structure and ability to take advantage of the call stack. However, the specific performance difference will depend on the implementation and problem being solved.
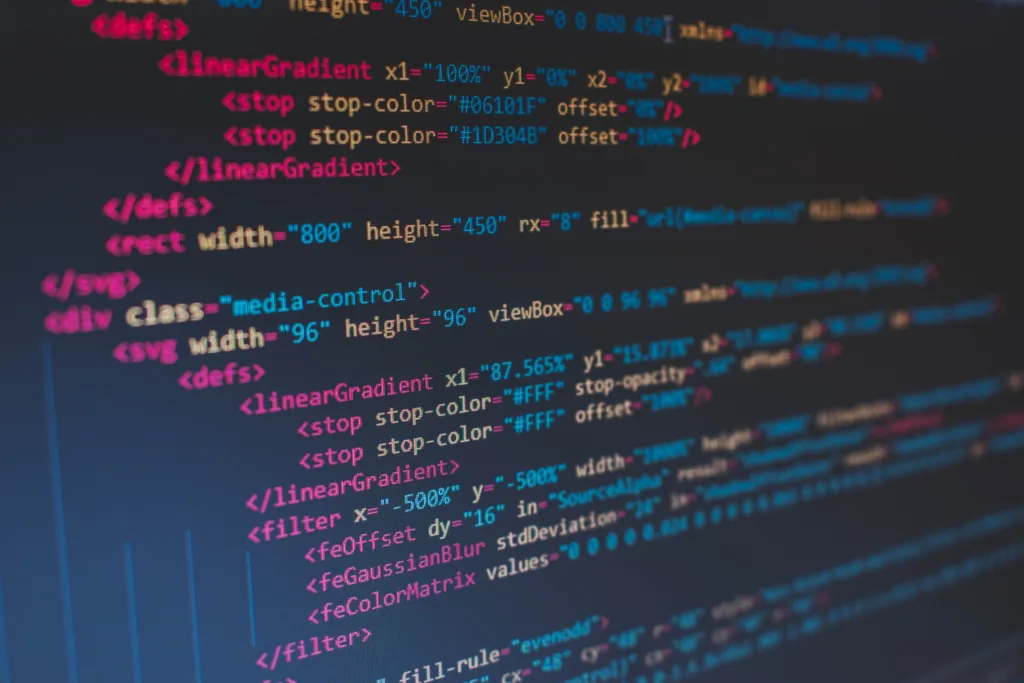
How Is Recursion Better Than Iteration?
Recursion and iteration are two common programming techniques used to solve problems. Recursion is a technique in which a function calls itself repeatedly until a base condition is met. On the other hand, iteration is a technique in which a loop is used to execute a set of statements repeatedly until a condition is met.
Recursion is better than iteration in certain situations because of the following reasons:
1. Code simplicity: Recursive code is often shorter and simpler than iterative code. It can be easier to read and understand because it is based on the natural way of thinking about the problem.
2. Fewer variables: Recursive code often requires fewer variables than iterative code. This is because the function calls itself, passing the necessary information as arguments.
3. Memory management: Recursion can be more memory-efficient than iteration in some cases. This is because the function calls are stored on the stack, which is a finite resource. However, in some cases, recursion can lead to a stack overflow if the recursion depth is too large.
4. Tail recursion optimization: In some languages, tail recursion optimization can be applied to recursive functions. This optimization allows the compiler to convert a recursive function into an iterative one, which can improve performance and reduce memory usage.
5. Recursive thinking: Recursion is a powerful way of thinking about problems. It can be used to solve a wide range of problems, from mathematical calculations to complex programming tasks.
Recursion is better than iteration in certain situations because it can simplify code, require fewer variables, be more memory-efficient, alow tail recursion optimization, and promote recursive thinking.
Is Iteration Faster Than Recursion In Python?
Iteration is generally faster and more memory-efficient than recursion in Python. The reason for this is that recursion involves repeated function calls, which can cause a stack overflow if the recursion depth is too large. In contrast, iteration uses a loop to repeatedly execute a block of code, withot the need for function calls.
Another factor that contributes to the speed and efficiency of iteration is that Python does not store any information about previous iteration steps, whereas recursion requires the storage of a call stack. This means that iteration does not require as much memory as recursion.
To summarize, here are some key points to keep in mind:
– Iteration is generally faster and more memory-efficient than recursion in Python.
– Recursion can cause a stack overflow if the recursion depth is too large.
– Iteration uses a loop to execute a block of code repeatedly, while recursion involves repeated function calls.
– Python does not store any information about previous iteration steps, which contributes to the speed and efficiency of iteration.
Do Recursive Methods Run Faster?
Recursive methods do not necessarily run faster than iterative methods. In fact, iterative methods are generally faster and more efficient than recursive methods beause they avoid the overhead of function calls. Recursive methods have to repeatedly call the same function, which can be slower and consume more memory.
However, recursive methods have their own advantages. They can be more straightforward and easier to understand, especially when dealing with complex recursive data structures like trees. Recursive methods can also be more concise and elegant, as they often require fewer lines of code than iterative methods.
It is important to note that the performance of recursive methods depends on the specific task and the implementation of the algorithm. In some cases, a recursive approach may be the most efficient option. In other cases, an iterative approach may be preferable. It is always important to carefully consider the requirements of the task and choose the appropriate method accordingly.
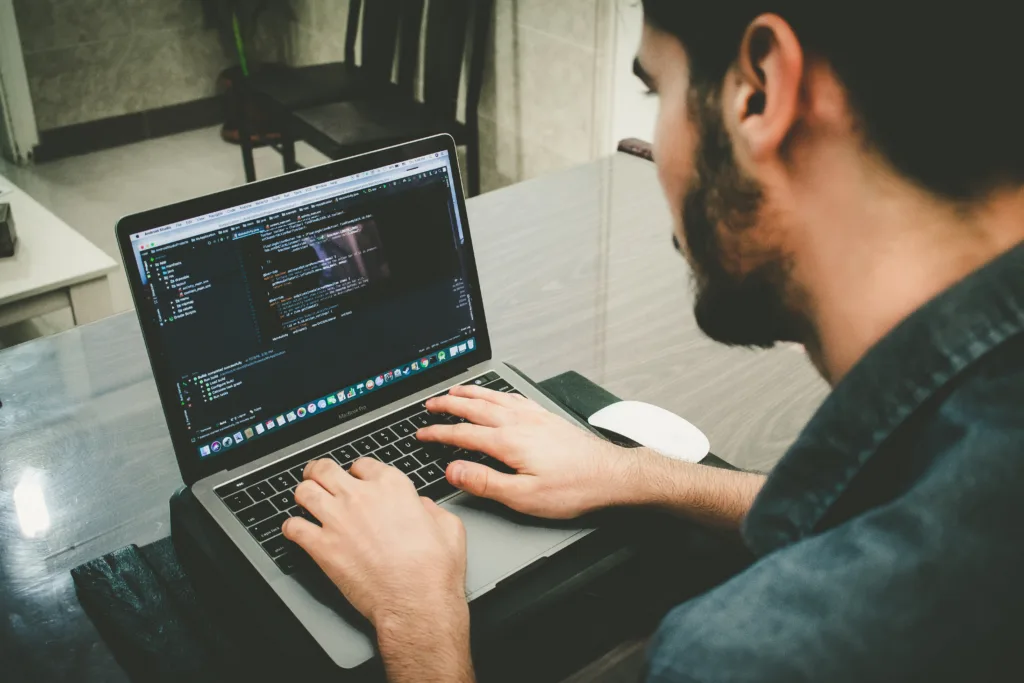
Conclusion
Recursion is a powerful technique in programming that allows a function to call itself in order to solve a problem. While recursion may be slower and less memory-efficient than iteration, it can be a useful tool for solving certain types of problems such as tree traversal and finding the Fibonacci series. Recursive functions are also often easier to read and write than ther iterative counterparts, making them a valuable addition to any programmer’s toolbox. However, it is important to use recursion judiciously and to be aware of its potential limitations in terms of performance and memory usage. recursion is a versatile and valuable tool for solving a wide range of programming problems.