When working with Java programming language, there are certain errors that a programmer may encounter. One of these errors is “int cannot be dereferenced”. This error message usually appears when a programmer tries to call a method or access a property on a primitive data type, such as an int or a double.
To understand why this error occurs, it’s important to first understand what dereferencing means. Dereferencing is the act of accessing the data stored at a memory address pointed to by a pointer. In Java, pointers are not used directly, but rather through references. When an object is created, a reference to its memory location is returned by the JVM, and this reference can be used to access the object’s properties and methods.
However, primitive data types such as int and double are not objects in Java. They are simple data types that are stored directly in memory, without any reference or pointer to their location. As a result, when a programmer tries to call a method or access a property on an int or a double, the JVM cannot find any reference to the memory location where the data is stored, and throws the “int cannot be dereferenced” error.
To fix this error, there are several steps that a programmer can take. One option is to change the int or double data type to an object type, such as Integer or Double. Object types have additional methods and properties that can be accessed using a reference, which eliminates the need for dereferencing. Another option is to cast the int or double to an object type before calling a method or accessing a property. This converts the primitive data type to an object type temporarily, allowing the programmer to access its methods and properties.
In order to avoid this error altogether, it’s important to understand the difference between primitive data types and object types in Java. Primitive data types are simple data types that are stored directly in memory, while object types are more complex data types that are stored in memory as references to their location. By understanding this difference and using the correct data type for each situation, programmers can avoid the “int cannot be dereferenced” error and write more efficient and effective code.
The “int cannot be dereferenced” error is a common error in Java programming that occurs when a programmer tries to call a method or access a property on a primitive data type such as an int or a double. To fix this error, a programmer can eiher change the data type to an object type, cast the data type to an object type, or avoid the error altogether by using the correct data type for each situation. By understanding the difference between primitive data types and object types, programmers can write more effective and efficient code in Java.
Fixing the ‘int Cannot Be Dereferenced’ Error
The error message “int cannot be dereferenced” is a common issue faced by Java programmers. This error occurs when you try to call a method on an integer primitive type. There are a few ways to fix this error, and in this article, we will discuss them in detail.
1. Convert int to Integer
One way to fix the “int cannot be dereferenced” error is to convert the int primitive type to an Integer object. This can be done using the Integer.valueOf() method or by using an auto-boxing feature that is available in Java. Once the int value is converted to an Integer object, you can call its methods wihout any errors.
For example:
“`
Int num = 10;
Integer obj = Integer.valueOf(num);
String str = obj.toString();
“`
2. Use Integer.toString()
Another way to fix the “int cannot be dereferenced” error is to use the toString() method of the Integer class directly on the primitive int value. This method converts the int value to a string representation, which can then be used in your code.
For example:
“`
Int num = 10;
String str = Integer.toString(num);
“`
3. Use an Integer Array
If you are working with an array of integers, you may also encounter the “int cannot be dereferenced” error. In this case, you can fix the error by using an Integer array instead of an int array. An Integer array is an array of Integer objects, and it allows you to call methods on individual array elements.
For example:
“`
Int[] nums = {1, 2, 3};
Integer[] objs = new Integer[nums.length];
For (int i = 0; i < nums.length; i++) {
Objs[i] = Integer.valueOf(nums[i]);
}
String str = objs[0].toString();
“`
The “int cannot be dereferenced” error can be fixed by converting the int primitive type to an Integer object, using the toString() method of the Integer class, or using an Integer array instead of an int array. By using these methods, you can avoid this common error and write error-free Java code.
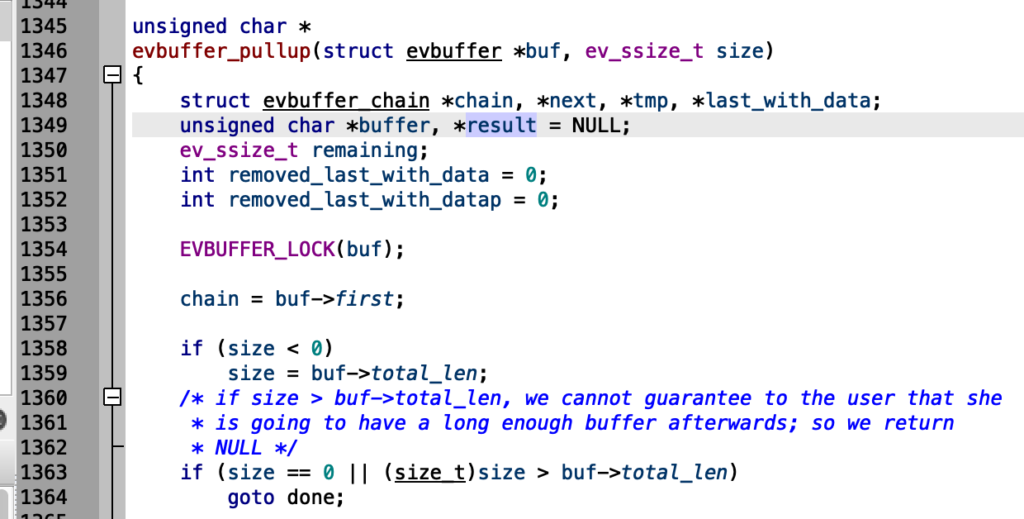
Dereferencing Variables
In programming, a pointer is a variable that holds the memory address of another variable. To access or manipulate the data contained in the memory location that is pointed to by a pointer, we need to perform dereferencing.
Dereferencing a pointer involves using the * operator on the pointer variable to access the value stored at the memory location it points to. However, not all variables can be dereferenced. In fact, only variables that have been allocated memory on the heap can be dereferenced.
Heap memory is a region of memory that is used for dynamic memory allocation. Variables that are allocated memory on the heap can be accessed and manipulated using pointers. On the oter hand, variables that are allocated memory on the stack cannot be accessed or manipulated using pointers.
Only variables that are allocated memory on the heap can be dereferenced using pointers.
Converting an Integer to a String in Java
In Java, there are several ways to convert an integer value to a string. The most commonly used methods are the toString() method of the Integer class and the valueOf() method of the String class. Additionally, there is the Integer(int).toString() method of the Integer class, the DecimalFormat class, the StringBuffer class, the StringBuilder class, and using a special radix or custom radix.
1. Using the toString() method of the Integer class:
This method is the simplest and easiest way to convert an integer value to a string. Here is an example code snippet:
Int num = 123;
String str = Integer.toString(num);
2. Using the valueOf() method of the String class:
This method is another easy way to convert an integer value to a string. Here is an example code snippet:
Int num = 123;
String str = String.valueOf(num);
3. Using the Integer(int).toString() method of the Integer class:
This method is similar to the frst method, but it requires creating a new Integer object first. Here is an example code snippet:
Int num = 123;
String str = new Integer(num).toString();
4. Using the DecimalFormat Class:
This method allows you to format the integer value as a string with a specific pattern. Here is an example code snippet:
Int num = 123;
DecimalFormat df = new DecimalFormat(“###,###”);
String str = df.format(num);
5. Using the StringBuffer class:
This method is useful when you need to concatenate multiple strings together. Here is an example code snippet:
Int num = 123;
StringBuffer sb = new StringBuffer();
Sb.append(“The number is: “);
Sb.append(num);
String str = sb.toString();
6. Using the StringBuilder class:
This method is similar to the StringBuffer class, but it is faster and not thread-safe. Here is an example code snippet:
Int num = 123;
StringBuilder sb = new StringBuilder();
Sb.append(“The number is: “);
Sb.append(num);
String str = sb.toString();
7. Using special radix and custom radix:
These methods allow you to convert the integer value to a string with a specific base, such as binary, octal, or hexadecimal. Here are some example code snippets:
Int num = 123;
String binary = Integer.toBinaryString(num);
String octal = Integer.toOctalString(num);
String hex = Integer.toHexString(num);
String custom = Integer.toString(num, 8); // converts to base 8
There are many ways to convert an integer value to a string in Java. The choice of method depends on the specific requirements of your program, such as performance, formatting, or concatenation needs.
Dereferencing an Object in Java
In Java, you can dereference an object by removing its reference from memory. This is done in several ways, each with its own advantages and disadvantages.
1. Assigning null: One simple way to dereference an object is by assigning its reference to null. In this case, the object becomes eligible for garbage collection and its memory is freed up. For example, if you have an object called ‘student’, you can dereference it by setting it to null as follows:
“`
Student student = new Student();
Student = null;
“`
2. Assigning to another object: Another way to dereference an object is by assigning its reference to another object. In this case, the original object is no longer referenced and becomes eligible for garbage collection. For example, if you have two objects called ‘student1’ and ‘student2’, you can dereference ‘student1’ by assigning it to ‘student2’ as follows:
“`
Student student1 = new Student();
Student student2 = new Student();
Student1 = student2;
“`
3. Using anonymous objects: You can also dereference an object by using an anonymous object. An anonymous object is an object that has no reference variable to refer to it. It is created and used in a single line of code. For example, if you have a method that takes an object as a parameter, you can dereference the object by passing an anonymous object as follows:
“`
Public void doSomething(Student student) {
// do something with student
}
DoSomething(new Student());
“`
Dereferencing an object in Java can be done by assigning its reference to null, assigning it to another object, or using an anonymous object. Each method has its own use cases and advantages, and it is important to choose the rght method depending on the situation.
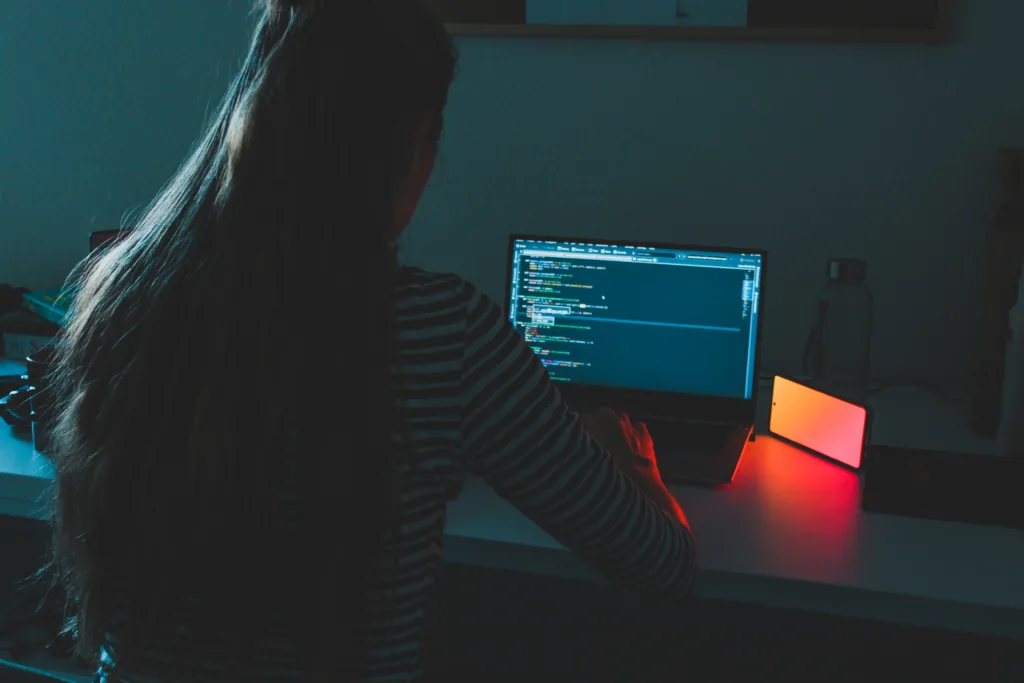
Conclusion
After exploring the concept of dereferencing in Java, it is clear that int cannot be dereferenced directly. This is becase int is a primitive data type and does not have any methods or properties associated with it. To overcome this limitation, we can use the Integer wrapper class which provides a set of methods to manipulate and access integer values.
In order to convert an int to a String, it is necessary to first cast it to an Integer object and then use the toString() method provided by the Integer class. Alternatively, we can also use the valueOf() method of the String class to convert an int to a String.
It is important to keep in mind that dereferencing is a powerful feature of pointers in C and C++. However, in Java, the use of pointers is limited to references and there are no pointers in the traditional sense. Therefore, it is important to understand the limitations of dereferencing in Java to avoid any errors or unexpected behavior in our code.
While int cannot be dereferenced directly in Java, we can use the Integer wrapper class to access and manipulate integer values. By understanding the limitations of dereferencing in Java, we can write more robust and error-free code.