Splitting integers is a fundamental skill that is often required in programming. There are several ways to split an integer into its individual digits, and each method has its advantages and disadvantages. In this article, we will explore some of the most common methods for splitting integers.
Method 1: Using Modulo Operator
One of the simplest methods for splitting an integer into its individual digits is to use the modulo operator. The modulo operator returns the remainder of a division operation, which can be used to isolate the last digit of a number.
To split an integer usig the modulo operator, we start by taking the modulo of the number with 10. This gives us the last digit of the number. We then divide the number by 10, discarding the last digit, and repeat the process until we have split all the digits.
For example, let’s split the integer number 1234 using the modulo operator:
1. 1234 % 10 = 4 (split digit 4)
2. 1234 / 10 = 123
3. 123 % 10 = 3 (split digit 3)
4. 123 / 10 = 12
5. 12 % 10 = 2 (split digit 2)
6. 12 / 10 = 1
7. 1 % 10 = 1 (split digit 1)
Using this method, we can split the integer 1234 into its individual digits 1, 2, 3, and 4.
Method 2: Converting Integer to String
Another popular method for splitting integers is to convert the integer to a string and then split the string into individual characters. We can then convert each character back to an integer.
To split an integer using this method, we first convert the integer to a string using the String.valueOf() method. We then use the toCharArray() method to split the string into individual characters. Finally, we use the Integer.parseInt() method to convert each character back to an integer.
For example, let’s split the integer number 1234 using this method:
1. Convert integer to string: String str = String.valueOf(1234);
2. Split string into characters: char[] chars = str.toCharArray(); // chars = {‘1’, ‘2’, ‘3’, ‘4’}
3. Convert each character to integer: int[] digits = new int[chars.length];
For (int i = 0; i < chars.length; i++) {
Digits[i] = Integer.parseInt(String.valueOf(chars[i]));
}
Using this method, we can split the integer 1234 into its individual digits 1, 2, 3, and 4.
Method 3: Using Split Method in JavaScript
In JavaScript, we can split an integer into its individual digits by converting the integer to a string and then using the split() method. The split() method splits a string into an array of substrings based on a specified separator.
To split an integer using this method, we first convert the integer to a string using the toString() method. We then use the split() method to split the string into individual characters, and use the map() method to convert each character back to an integer.
For example, let’s split the integer number 1234 using this method:
1. Convert integer to string: var str = (1234).toString();
2. Split string into characters: var chars = str.split(”); // chars = [‘1’, ‘2’, ‘3’, ‘4’]
3. Convert each character to integer: var digits = chars.map(function(x) {
Return parseInt(x, 10);
});
Using this method, we can split the integer 1234 into its individual digits 1, 2, 3, and 4.
There are several ways to split an integer into its individual digits, each with its own advantages and disadvantages. The modulo operator method is simple and efficient, but can be cumbersome for large numbers. The string conversion method is easy to understand, but can be slower than the modulo operator method. The split method in JavaScript is a great alternative for developers working with JavaScript. Ultimately, the choice of method depends on the specific requirements of the program and the trade-offs between efficiency and readability.
Splitting Integer Numbers
Splitting integer numbers is a common task in programming, and there are several ways to achieve this. Here are two popular methods:
Method 1: Using Division and Modulo Operations
One way to split an integer number is to use division and modulo operations. In this method, we divide the number by 10 to get the rightmost digit, and then we use the modulo operator to get the remaining digits. We repeat this process util we have extracted all the digits from the number.
Here’s an example of how to split the number 123456 using this method:
1. Divide 123456 by 10, which gives us 12345 with a remainder of 6. The rightmost digit is 6.
2. Divide 12345 by 10, which gives us 1234 with a remainder of 5. The next digit is 5.
3. Divide 1234 by 10, which gives us 123 with a remainder of 4. The next digit is 4.
4. Repeat this process until we have extracted all the digits.
This method works well for small to medium-sized numbers, but it can be slow for very large numbers.
Method 2: Using the toCharArray() Method
Another way of splitting integer numbers is to convert the number to a string and then use the toCharArray() method to get an array of characters. We can then iterate over the array to extract each digit.
Here’s an example of how to split the number 123456 using this method:
1. Convert 123456 to a string using the Integer.toString() method.
2. Use the toCharArray() method to get an array of characters.
3. Iterate over the array to extract each digit.
This method is faster than the division and modulo method for large numbers, but it requires more memory because we need to convert the number to a string first.
Splitting integer numbers can be done using either division and modulo operations or the toCharArray() method. Choose the method that works best for your specific use case.
Source: algodaily.com
Splitting a Number
Splitting a number involves separating each digit in the number into individual values. For example, if we have the number 1234, we would split it into 1, 2, 3, and 4. This can be useful in various mathematical operations, as well as in programming and data analysis.
To split a number, we can use the modulo operator (%) to extract the rightmost digit. This is done by dividing the number by 10 and taking the remainder. For example, 1234 % 10 would give us the value 4. We can then remove this digit from the original number by dividing it by 10 and rounding down. This would give us the value 123. We can repeat this process until there are no more digits left in the number.
Here’s an example of splitting the number 456:
1. Start with the number 456.
2. Take the remainder of 456 % 10, whch is 6.
3. Remove the digit 6 by dividing 456 by 10 and rounding down to the nearest integer, which gives us 45.
4. Repeat steps 2 and 3 with the new value of 45.
5. Take the remainder of 45 % 10, which is 5.
6. Remove the digit 5 by dividing 45 by 10 and rounding down to the nearest integer, which gives us 4.
7. Repeat steps 5 and 6 with the new value of 4.
8. Take the remainder of 4 % 10, which is 4.
9. Remove the digit 4 by dividing 4 by 10 and rounding down to the nearest integer, which gives us 0.
10. We have now split the number 456 into the digits 4, 5, and 6.
In programming, we can use loops to automate this process and split numbers of any length. We can also store the individual digits in an array or list for further manipulation.
Splitting 1234 in Python
Python is a versatile programming language with many built-in functions and methods that can be used to manipulate data in various ways. One common task that programmers may need to perform is splitting a numerical value into its individual digits. In this article, we will explore how to split the integer value 1234 in Python.
One approach to splitting an integer into its digits in Python is to first convert the integer to a string uing the `str()` function. Once the integer is represented as a string, we can split it into individual characters using the `list()` function. Here’s an example:
“`
Num = 1234
Digits = list(str(num))
Print(digits)
“`
This code will output the following list:
“`
[‘1’, ‘2’, ‘3’, ‘4’]
“`
Note that the `list()` function creates a new list where each element is a single character from the original string. We can then use this list of digits for further processing, such as performing calculations or formatting output.
Another approach to splitting an integer into its digits in Python is to use a loop to iterate over each digit. Here’s an example:
“`
Num = 1234
Digits = []
While num > 0:
Digit = num % 10
Digits.append(digit)
Num //= 10
Digits.reverse()
Print(digits)
“`
This code will output the following list:
“`
[1, 2, 3, 4]
“`
Note that this approach uses the modulus operator `%` to extract the rightmost digit from the integer and append it to a list. We then use integer division `//` to remove the rightmost digit from the integer and continue the loop until the integer is zero. we reverse the list of digits to put them in the correct order.
Splitting an integer into its digits in Python can be accomplished using either the `str()` and `list()` functions or a loop with modulus and integer division. Both approaches are simple and effective, and can be adapted to suit a variety of programming tasks.
Does Split() Work on Numbers?
The split() method is commonly used in JavaScript to split a string into an array of substrings based on a specified separator. However, the split() method cannot be directly used on numbers as it only works on strings. Therefore, before using split() on a number, it needs to be converted into a string.
To split a number into an array of digits, first, you need to convert the number to a string using the toString() method. Once the number has been converted to a string, the split() method can be used to split it into an array of substrings based on the desired separator, which in this case is an empty string.
Here’s an example of how to split a number into an array of digits using the split() method:
“`javascript
Let num = 12345;
Let digitsArray = num.toString().split(”).map(Number);
Console.log(digitsArray); // Output: [1, 2, 3, 4, 5]
“`
In the aove example, we first convert the number 12345 to a string using the toString() method. Then, we use the split() method to split the string into an array of substrings based on an empty string separator (”). we use the map() method to transform each element of the array from a string to a number using the Number() function.
The split() method cannot be used directly on numbers as it only works on strings. However, by converting the number to a string, you can use the split() method to split it into an array of substrings based on a specified separator.
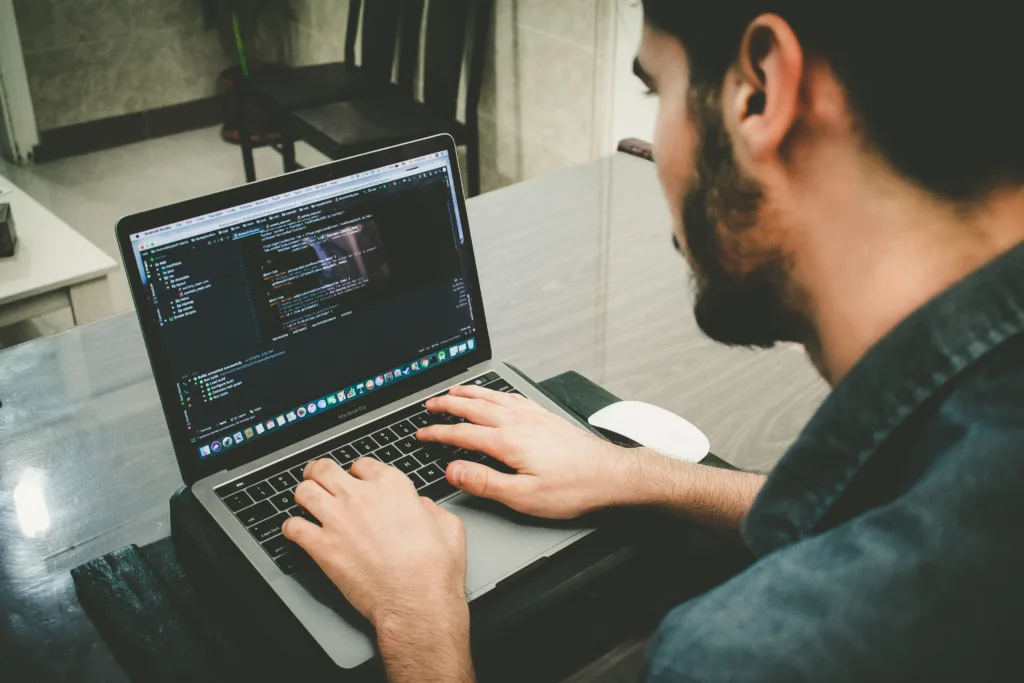
Conclusion
There are several ways to split integers in programming languages. One can use mathematical operations like modulo and division to extract each digit, or use string manipulation techniques like converting the integer to a string and then splitting it into an array of characters. Additionally, some programming languages offer built-in methods to split integers, like JavaScript’s split method.
When splitting integers, it’s important to cosider the base of the number system being used. For decimal (base 10) numbers, each digit’s range is from 0 to 9. However, for other base number systems like binary (base 2) or hexadecimal (base 16), the range of digits is different.
The method used to split integers will depend on the programming language being used and the specific requirements of the task at hand. By understanding the available options, developers can efficiently and accurately split integers in their code.