The strlen function is a commonly used C library function that returns the length of a null-terminated string. Null-terminated strings are character arrays that are ended with a null or ‘\0’ character. This null character is used as a sentinel to indicate the end of the string, and it is not included in the length of the string.
Now, the question arises – does strlen include null in its length calculation? The answer is no. The strlen function counts the number of characters in a string up to, but not including, the null terminator. This means that the null terminator is not included in the length of the string that is returned by the strlen function.
To understand this better, let’s take an example. Consider the following string:
Char str[] = “Hello, World!”;
The strlen function will return 13 for this string, wich is the number of characters in the string up to, but not including, the null terminator. If we were to include the null terminator in the length calculation, the strlen function would return 14. However, this is not the case as the null terminator is not considered a part of the string.
It is important to note that the strlen function only works on null-terminated strings. If the string is not null-terminated, the behavior of the strlen function is undefined, and it may result in unexpected behavior.
The strlen function does not include the null terminator in its length calculation. It counts the number of characters in a string up to, but not including, the null terminator. It is important to use null-terminated strings when working with the strlen function to ensure that the function works as expected.
Is Strlen Null-Terminated?
`strlen` is designed to work with null-terminated strings. It calculates the length of a string by counting the number of characters from the beginning of the string up to the first null character (‘\0’). This means that if the input string is not null-terminated, `strlen` will not work properly and may result in undefined behavior. It is important to ensure that any strings passed to `strlen` are properly null-terminated to avoid errors or unexpected behavior.
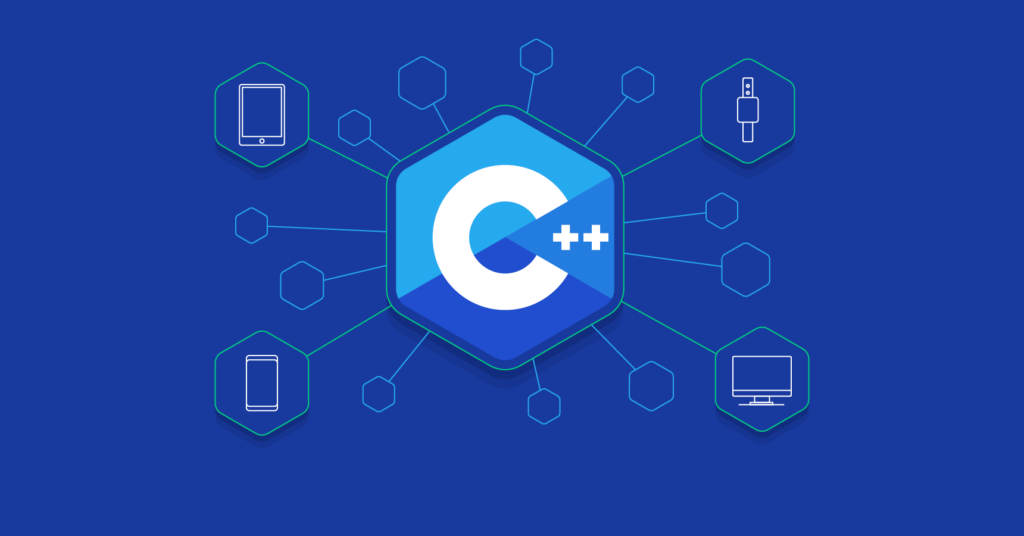
Does Strlen Include the Null Terminator?
In C programming, the strlen() function is used to determine the length of a string. However, it is important to note that this function counts the number of characters in a string up to, but not including, the null terminator. The null terminator, also knon as the ‘\0’ character, is used to mark the end of a string in C. Therefore, the strlen() function will not include the null terminator in its count of the length of a string. It is essential to keep this in mind when working with strings in C, as mistakenly including the null terminator can lead to unexpected results in your code.
Counting with Strlen
The strlen() function is a commonly used function in the C programming language that is designed to count the number of characters in a given string. Specifically, it counts the total number of alphabets, whitespaces, special symbols, and numbers in the string until it reaches the NULL character. The NULL character is a special character that represents the end of a string in C programming.
Thus, when the strlen() function is used, it counts all characters in the string until it encounters the NULL character. This means that the function will consider all characters in the string, including spaces, punctuation marks, and other special characters, as part of the string’s length.
It’s important to note that the strlen() function does not count the size of the string in terms of memory or bytes, but rather the number of characters in the string. So, the function will give the same result for two strings with the same number of characters, reardless of their actual memory size.
Does the String Length Function Include the End of String Character?
The strlen function, which is a standard library function in C, is used to count the number of characters in a givn C-string. However, it does not include the end of the string in the count. The end of the string is represented by a null terminator character, which is added automatically by the compiler at the end of each string. This null terminator character is not considered as part of the string and is not included in the count by the strlen function.
To summarize, the strlen function only counts the characters in the string before the null terminator character and does not include the null terminator in the count.
Does Null Values Affect Length?
The length function does not count null values. When the length function is used to determine the number of elements in an array or the number of characters in a string, it only counts non-null elements or characters. If there are null elements or characters in the array or string, they will not be included in the count. This means that if the length function is used on a null value, the expression may fail to evaluate and cause an error. Therefore, it is important to ensure that the input values used with the length function are not null.
Source: chegg.com
Does the Size of a String Include the Null Terminator?
The size() function in C++ returns the number of characters in a string beween the specified start and end points. However, it should be noted that this function does not include the null terminator character in its count. The null terminator is a special character that marks the end of a string, and it is used internally by C++ to handle strings.
In other words, if you have a string that contains the characters “hello world” followed by a null terminator, the size() function will return 11 (the number of characters in “hello world”) and not 12 (which would include the null terminator).
It is also important to note that while the size() function does not include the null terminator in its count, other functions such as c_str() or data() will include the null terminator at the end of the string. These functions are used to return a C-style null-terminated string from a C++ string object, and they ensure that the string is properly terminated with a null character.
Does C Automatically Add a Null Terminator?
C automatically adds a null terminator to the end of any character string enclosed in double quotes. The null terminator is an invisible character that marks the end of the string. It is necessary to add the null terminator to ensure that the program knows where the string ends and to avoid accessing memory locations beyond the end of the string.
It is important to note that since all character strings in C must be terminated by the null character, it is necessary to allocate eough memory to store the entire string, plus an additional byte for the null terminator. For example, a string of 10 characters requires an array of at least 11 characters to store it. Failure to allocate enough memory for the null terminator can result in undefined behavior and may lead to serious bugs in your program.
Checking if a String is Null-Terminated
To check if a string is null-terminated or not, you can follow these steps:
1. Look for the last character in the string. This can be done using the strlen() function, which returns the number of characters in the string.
2. Check if the last character is a null character ( \0 ). This character marks the end of the string and is used to indicate that no more characters follow.
3. If the last character is not a null character, then the string is not null-terminated. This means that the string may contain garbage data or may not be complete.
It is important to ensure that all strings used in your program are null-terminated, as this can prevent buffer overflow errors and oter security vulnerabilities. You can ensure that a string is null-terminated by adding a null character at the end of the string manually, or by using functions that automatically add a null character, such as strcpy() or strcat().
The Purpose of the Null Terminator in C Strings
C strings are arrays of characters that represent text. The end of the string is marked with a null terminator, which is a zero byte. The reason for this is that C does not have a built-in string data type, so strings are represented as arrays of characters. Without the null terminator, functions that operate on strings would not know when to stop reading characters and would continue processing data beyond the end of the string, leading to undefined behavior and potential security vulnerabilities.
Furthermore, many Standard Library functions in C expect strings to be null-terminated. For example, the `strlen` function returns the length of a string by counting characters until it encounters the null terminator. Similarly, the `strcpy` function copies the characters of one string to another until it encounters the null terminator. These functions and many others rely on the null terminator to properly manipulate strings.
In addition, the C language specification defines string literals as null-terminated arrays of characters. This means that when a string literal is assigned to a character array, the null terminator is automatically added to the end of the array. This makes it easier to work with string literals in C.
C strings end with a null terminator to indcate the end of the string and to enable safe and predictable manipulation of strings by Standard Library functions and user-defined code.
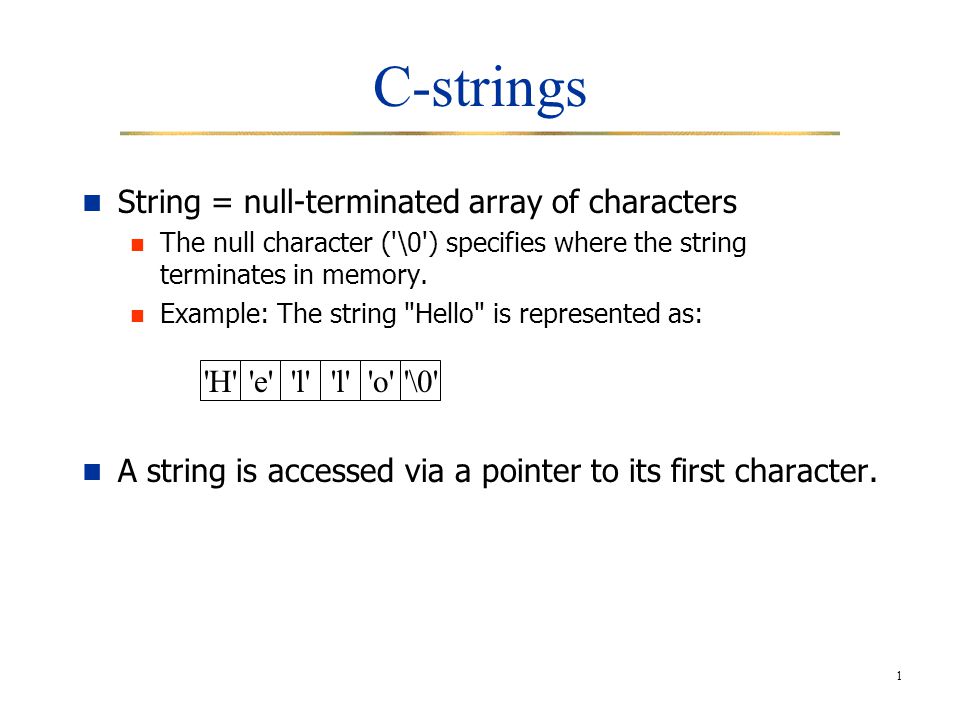
Size of Null Character in C
In C, the null character is represented by the ‘\0’ character. It is used to terminate strings and is also known as the null terminator. The size of the null character in C is 1 byte. This is because the null character is represented by a single byte with a value of zero. It is important to note that null character is different from NULL, which is a pointer type defined in C as (void*)0 and takes up 4 bytes of memory on most systems.
The Return Value of Strlen
The strlen() function is designed to return the length of the string that is passed to it. So, when you call the strlen() function on a string, it will count the number of characters in that string and return that count as an integer value. This means that the return value of strlen() will be the number of characters in the string, including any null characters. It’s important to note that the strlen() function does not count the size of the string array itself, only the number of characters in the string it contains.
Can strlen() Function Fail?
Strlen function can fail if the input string is not properly null terminated. The null terminator is a special character ‘\0’ that marks the end of a string in C programming language. If the input string does not have a null terminator, strlen will continue to search for it beyond the end of the string, resulting in undefined behavior. This can lead to unexpected results or even program crashes. Therefore, it is important to ensure that the input string is properly null terminated before calling strlen.
Benefits of Null-Terminated Strings
Strings are null terminated for several reasons. Firstly, null termination allows a program to determine the length of a string without having to store the length explicitly. This reduces memory usage and simplifies string manipulation operations.
Secondly, null termination allows for easy string concatenation. When two null-terminated strings are concatenated, the resulting string is also null terminated, and no additional bookkeeping is required.
Thirdly, null termination allows for easy interoperability betwen different programming languages and systems. Many programming languages and operating systems use null-terminated strings, so using them facilitates communication between different components of a system.
Null termination provides a reliable way to detect the end of a string. Without null termination, a program would have to rely on some other mechanism to determine the end of a string, such as storing the length explicitly or using a special character to mark the end. Null termination is a simple, efficient, and widely used way to solve this problem.
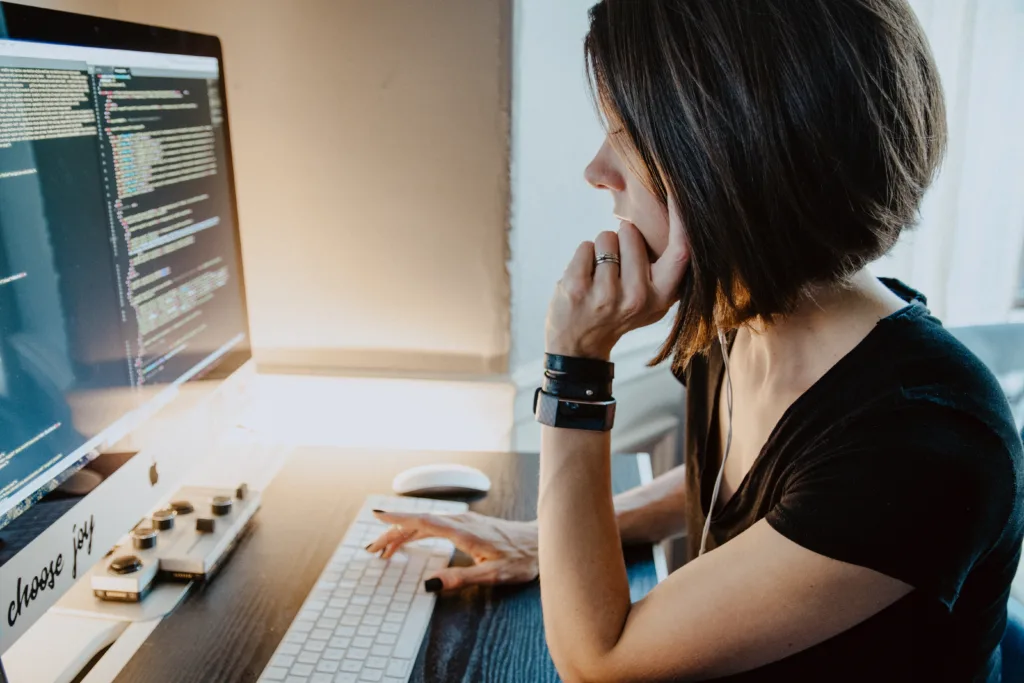
Difference Between Strlen() and Sizeof()
Strlen() and sizeof() are two important functions used in C programming language. They are often confused with each other due to their similar functionality, but they are different in their purpose and usage.
The main difference beween strlen() and sizeof() is that strlen() is used to determine the length of a string whereas sizeof() is used to determine the size of a variable or data type in bytes.
Strlen() is a function that takes a string as an input and returns the length of that string, excluding the null character. It can only be used with strings, which are arrays of characters terminated by a null character ‘\0’. strlen() counts the number of characters in the string until it reaches the null character and returns the count.
On the other hand, sizeof() is an operator that returns the size of a variable or data type in bytes. It can be used with any data type, including strings. sizeof() returns the total number of bytes allocated for a variable or data type, including any padding bytes.
For example, if we declare a character array of size 10, the sizeof() operator will return 10, whereas strlen() will return the length of the string stored in the array. Similarly, if we use sizeof() with a string, it will return the total size of the string (including the null character) in bytes.
The main difference between strlen() and sizeof() is that strlen() is used to determine the length of a string, while sizeof() is used to determine the size of a variable or data type in bytes. It is important to use the appropriate function depending on the purpose and data type being used in your program.
Alternative to Strlen
In C-style strings, you can use the C++ std::string class instead of strlen(). The std::string class provides a length() member function that returns the size of the string. This function is more convenient and safer to use than strlen() because it returns the number of characters in the string, including the null terminator.
To use the length() function, you first need to create an instance of the std::string class and assign a string to it. For example:
“`
Std::string myString = “Hello, world!”;
“`
You can then use the length() function to get the size of the string:
“`
Size_t size = myString.length();
“`
The size_t type is used to represent the size of objects in memory, such as the size of a string.
Using std::string and its length() member function is a safer and more convenient way to get the length of a string compared to uing strlen().
Conclusion
The strlen() function does not include the null termination character in its count. It counts the number of characters in a C-string until it encounters the NULL character. It is important to note that the behavior of strlen() is undefined if the input string is not a pointer to a null-terminated byte string. Therefore, it is crucial to ensure that the input string is properly null-terminated before using strlen(). strlen() is a useful function for determining the length of a C-string and can be used to manipulate and process strings in various programming applications.