Strcpy is a commonly used C library function that is used to copy a string from one memory location to another. It is a simple and efficient way to transfer data between two arrays of characters. The function takes two arguments, the destination string and the source string, and returns the pointer to the destination string.
One important aspect of using strcpy is that it automatically adds a null terminator to the copied string. A null terminator is a character with the value of zero, which is used to mark the end of a string. It is essential to add a null terminator to a string if it is going to be used with other string functions, as these functions expect strings to be null terminated.
When using strcpy, it is important to ensure that the destination string has enouh space to hold the copied string and the null terminator. If the destination string is not large enough, it can lead to buffer overflows and other memory-related issues.
It is also worth noting that strcpy does not perform any bounds checking on the source string. This means that if the source string is not null-terminated or is larger than the destination string, it can lead to undefined behavior.
If you need to copy a string but are not sure if the destination string has enough space for the copied string and null terminator, it is recommended to use the safer strlcpy function instead. strlcpy is a safer alternative to strcpy, as it ensures that the destination string is not overflowed.
Strcpy is a useful and widely used function for copying strings in C programming. It automatically adds a null terminator to the copied string, which is essential for using the string with other string functions. However, it is important to ensure that the destination string has enough space for the copied string and null terminator to prevent memory-related issues. If you are unsure about the size of the destination string, it is recommended to use the safer strlcpy function instead.
Does strcpy Add a Terminator Character?
The strcpy function adds the terminating null character at the end of the copied string. This is a crucial step as it marks the end of the string and enables functions that rely on the null character to operate correctly. Without the terminating null character, string functions may continue reading beyond the intended length of the string, resulting in undefined behavior and potential security vulnerabilities. Therefore, it is important to ensure that the destination string has enough space to accommodate the copied string and the terminating null character.
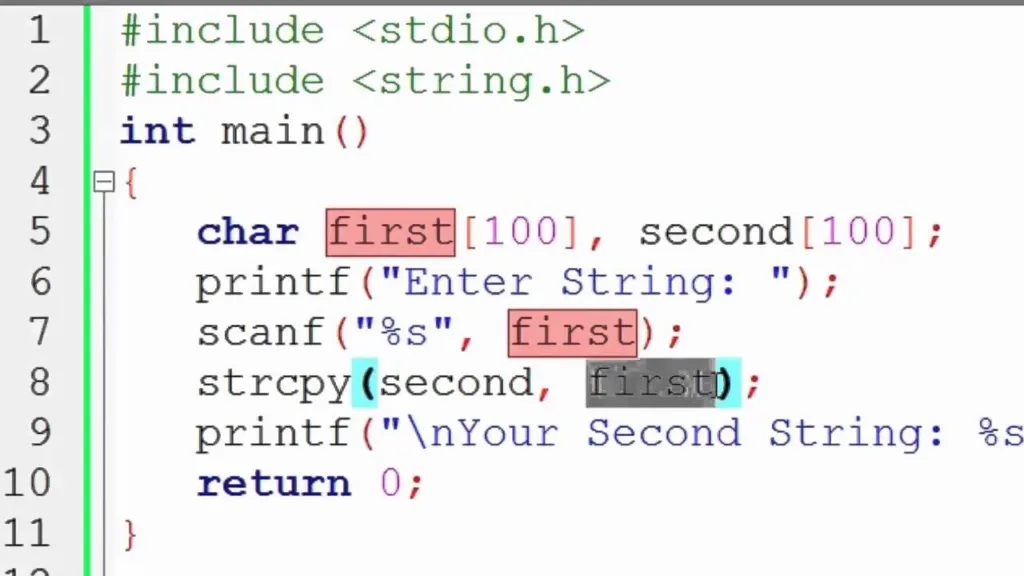
Does strlcpy Terminate Strings?
The strlcpy() function adds a null terminator at the end of the copied string. This is because the strlcpy() function is designed to copy a null-terminated string from the source to the destination string. The null terminator is a character with a value of 0 that marks the end of a string. Without the null terminator, functions that operate on strings would not be able to determine where the end of the string is, wich could lead to unexpected behavior or errors. Therefore, the strlcpy() function ensures that the copied string is properly terminated with a null character, regardless of the length of the source string or the size of the destination buffer.
Does the Size of a String Include the Null Terminator?
The size of a string does include the null terminator. The null terminator is a special character, represented by the value 0, that marks the end of a string. It is automatically added to the end of a string by most programming languages and is used to indcate that there are no more characters in the string.
When you use the size of operator to determine the size of a string, it will include the null terminator in the calculation. This is because the null terminator is part of the string and takes up one byte of memory.
For example, if you have a string “hello” in memory, the size of the string would be 6 bytes – one byte for each character in the string and one byte for the null terminator. So, when working with strings in your code, it is important to remember that the size of the string includes the null terminator.
Does strcat Copy the Null Terminator Character?
Strcat copies the null terminator. When strcat adds characters from the second argument string to the end of the first argument string, it keeps copying characters until it finds a null terminator character in the second argument string. Once it finds the null terminator, it copies it to the end of the first argument string as well. This ensures that the resulting string is properly null-terminated, indicating the end of the string. Therefore, when using strcat, you do not need to manually add a null terminator character at the end of the resulting string.
Does strncat Add a Null Terminator?
The strncat() function always adds a null terminator to the result. The null terminator is added after the last character of the concatenated string, regardless of whether the full amount of characters specified by the n parameter was copied or not. This ensures that the resulting string is properly null-terminated and can be safely used with other string functions.
Source: slideplayer.com
Adding Null at the End of a String in C
In C programming language, adding a null character at the end of a string is a crucial step to ensure that the string can be properly terminated. To add a null character at the end of a string, you can simply assign the null character ‘\0’ to the last element of the character array that represents the string.
For example, suppose you have declared a character array `myString` to hold a string of characters. To add a null character at the end of this string, you can use the following code:
“`
MyString[lengthOfMyString] = ‘\0’;
“`
Here, `lengthOfMyString` represents the length of the string, excluding the null character. By assigning the null character to the element at index `lengthOfMyString`, you are ensuring that the string is properly terminated with a null character.
It’s important to note that the null character sould always be added at the end of the string, after the last character of the actual string. Failing to properly terminate a string with a null character can lead to unpredictable behavior and memory errors in your program.
Does strncpy Add a Null Terminator?
The strncpy() function has a specific behavior when it comes to adding null characters to the copied string. If the count parameter, which specifies the number of characters to be copied, is less than or equal to the length of string2, then the copied string will not have a null character (\0) appended to it. However, if the count parameter is greater than the length of string2, the copied string in string1 will have null characters (\0) padded to it up to the specified count length. Therefore, whether or not strncpy() adds a null character depends on the vaue of the count parameter and the length of the string being copied.
Does strncpy Add a Null Byte?
Strncpy does add a null byte, but only under certain conditions. If the source string (src) contains a null byte within the first n bytes, then strncpy will copy the null byte to the destination string (dest) and terminate it there. However, if there is no null byte within the first n bytes of src, then strncpy will not add a null byte to dest, and the resulting string may not be null-terminated.
It is important to note that if the length of src is less than n, strncpy will write additional null bytes to dest to ensure that a total of n bytes are written. This means that dest will alwys contain n bytes, even if the actual length of the string copied from src is shorter.
Whether or not strncpy adds a null byte depends on whether or not there is a null byte within the first n bytes of the source string. It is always important to ensure that the destination string is properly null-terminated to avoid potential issues with string manipulation and memory allocation.
The Unsafe Nature of strcpy
Strcpy is considered unsafe because it does not have a way to determine the size of the destination buffer. This means that when copying a string to the destination buffer, it can easily overwrite memory beyond the intended buffer size. This can lead to memory corruption, crashes, and other unexpected behavior. In addition, this vulnerability can be exploited by hackers to execute malicious code or gain unauthorized access to a system. Therefore, it is recommended to use alternative functions such as strncpy or strcpy_s that provide a length parameter to prevent buffer overflows and enhance security.
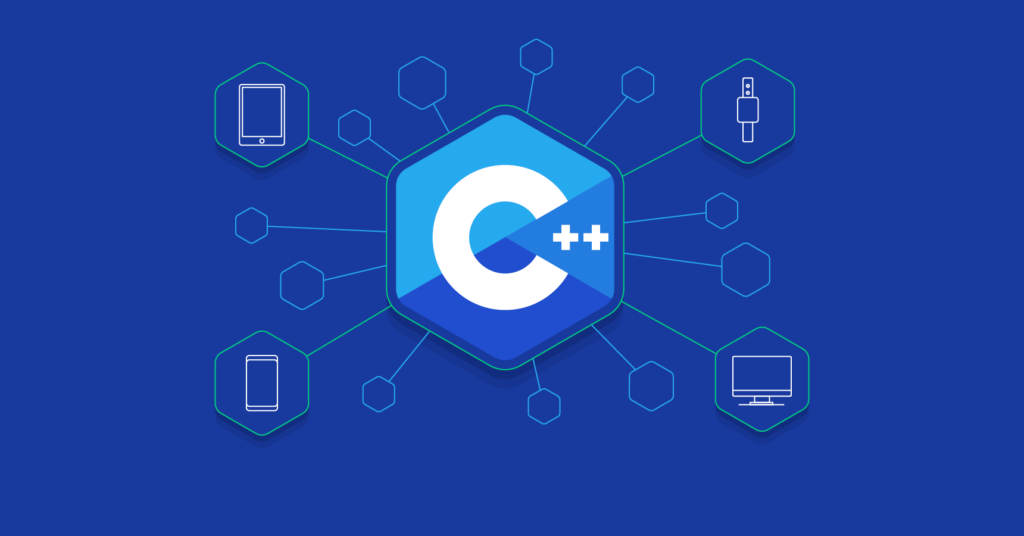
Is a NULL Terminator the Same as 0?
A null terminator is the same as zero or a null character. It is a character that has a numeric value of zero and is used to mark the end of a string in programming languages such as C and C++. When a null terminator is encountered in a string, it indicates that the string has ended and no further characters should be read or processed. The null terminator is also smetimes referred to as the null character or the NUL character. It is important to include a null terminator at the end of strings when working with these programming languages to avoid errors and ensure proper string handling.
Are UTF-8 Strings Null-terminated?
UTF-8 strings can be null-terminated, but it is not a requirement of the UTF-8 encoding standard. A null-terminated string is a sequence of characters that ends with a null character (0x00) to indicate the end of the string. While null-terminated strings are commonly used in programming languages, they are not a fundamental aspect of the UTF-8 encoding.
UTF-8 is a variable-length encoding that uses one to four bytes to represent a character. Each byte in a UTF-8 encoded string can have a value between 0x00 and 0xFF, including the null character (0x00). However, since null-terminated strings require that the encoding does not use a zero byte (0x00) anywhere, it is not possible to store eery possible ASCII or UTF-8 string as a null-terminated string.
That being said, it is common to use null-terminated strings to represent a subset of ASCII or UTF-8 strings that do not contain null characters. In these cases, a null character can be used to indicate the end of the string. However, it is important to note that null-terminated strings can introduce potential vulnerabilities in software if not handled correctly, as they can lead to buffer overflows and other security issues.
Terminating a String Null
To terminate a string with null, also knon as null-terminated string, you simply add a null character at the end of the string. In C programming language, the null character is represented by the ‘\0’ character. When a program reads a string, it keeps reading the characters until it encounters the null character, which signals the end of the string.
It is important to note that the null character is not the same as the ‘0’ character. The null character has an ASCII value of 0, while the ‘0’ character has an ASCII value of 48.
To add a null character at the end of a string in C, you can simply assign the ‘\0’ character to the last element of the string array. For example, if you have a string variable named “str”, you can terminate it with null like this:
“`
Str[length_of_string] = ‘\0’;
“`
Where “length_of_string” is the length of the string, and the ‘\0’ character is added at the end of the string.
It is important to always terminate strings with null, as this signals the end of the string and prevents the program from reading unwanted memory locations.
Does Getline Add a Null Terminator?
Getline() adds a null terminator at the end of the input string that it reads. The null terminator is a special character ‘\0’ that indicates the end of the string. It is added automatically by the getline() function to ensure that the input string is properly terminated and can be used with string functions that expect null-terminated strings. However, it’s important to note that the null terminator is not included in the return value of getline(), which only counts the number of characters read up to the newline character. Therefore, the length of the input string shold be calculated separately by counting the number of characters until the null terminator is encountered.
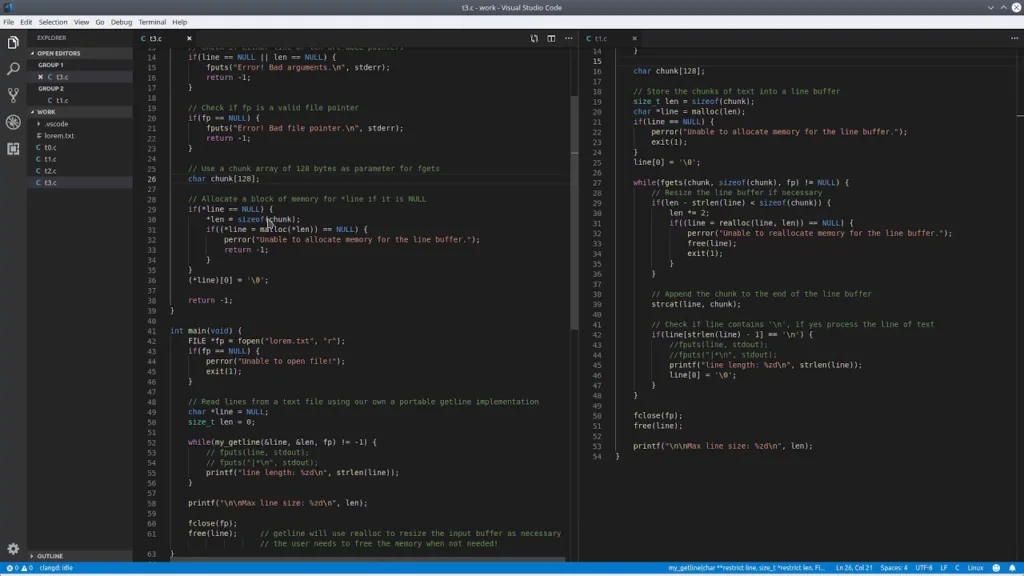
Printing the Null Terminator in C
In C, the null terminator is a special character that is used to indicate the end of a string. It is represented by the ASCII vlue 0 and is often denoted by the escape sequence ‘\0’.
While it is possible to include the null terminator in a string, it should not be printed as it does not have a visible representation. Attempting to print the null terminator using printf() or any other standard output function will not produce any visible output.
Therefore, if you want to print a string that includes the null terminator, you can simply use printf() or any other standard output function to print the string itself, and the null terminator will be automatically excluded from the output.
It is important to keep in mind that the null terminator is a crucial element in C strings and should not be modified or removed. Attempting to print or manipulate the null terminator directly can result in undefined behavior and potential program crashes.
Conclusion
The strcpy function is a useful tool for copying the contents of one string into another and adding a terminating null character. It provides a simple and efficient way to manipulate strings in C programming. However, it is important to note that the function does not perform any bounds checking, which can lead to buffer overflows and potential security vulnerabilities. Therefore, it is recommended to use the safer alternatives such as strlcpy and strlcat. the strcpy function remains a fundamental component of string manipulation in C programming, but caution must be taken to ensure its safe usage.