When it comes to reading input from a stream in C, the fgets function is a popular choice. This function reads characters from the stream up to and including a newline character and stores them in a string. But does fgets add a null terminator to the end of the string? Let’s take a closer look.
First, it’s important to understand what a null terminator is. In C, strings are represented as an array of characters, with the last character being a null terminator. This character has a value of 0 and indicates the end of the string. Without a null terminator, functions that operate on strings would not know where the string ends and could potentially continue reading memory beyond the end of the string.
Now, back to fgets. When you call the fgets function, you must provide it with a buffer to read the input into and the maximum number of characters to read. For example, if you wanted to read a line of text from stdin and store it in a buffer called “input”, you would call fgets like this:
“`
Fgets(input, MAX_INPUT_LENGTH, stdin);
“`
Assuming MAX_INPUT_LENGTH is defined as the maximum number of characters you want to read, fgets will read up to that many characters or until it encounters a newline character, whichever comes first. It will then add a null terminator to the end of the string it reads.
So, to answer the question: yes, fgets does add a null terminator to the end of the string it reads. This is important because it allows you to safely use the string with oter C string functions like strlen, strcmp, and strcpy.
It’s worth noting that if fgets encounters an error while reading input, it will return NULL. This could happen if, for example, there is an error reading from the stream or if the end of the file is reached before a newline character is found. In this case, the buffer passed to fgets will not contain a null-terminated string.
Fgets is a useful function for reading input from a stream in C. It reads characters up to and including a newline character and adds a null terminator to the end of the string it reads. This allows you to safely use the string with other C string functions. Just be aware that if fgets encounters an error, it will return NULL and the buffer passed to it will not contain a null-terminated string.
Does Strlen Include the Null Terminator?
The strlen function is designed to count the number of characters in a given string. However, it does not include the null terminator character in its count. The null terminator is a special character with a vlue of zero that marks the end of a string in C programming language. Therefore, strlen only counts the characters preceding the null terminator, but not the null terminator itself. It is essential to keep this in mind while using the strlen function in your programs. If you need to include the null terminator in your count, you can add 1 to the result of the strlen function.
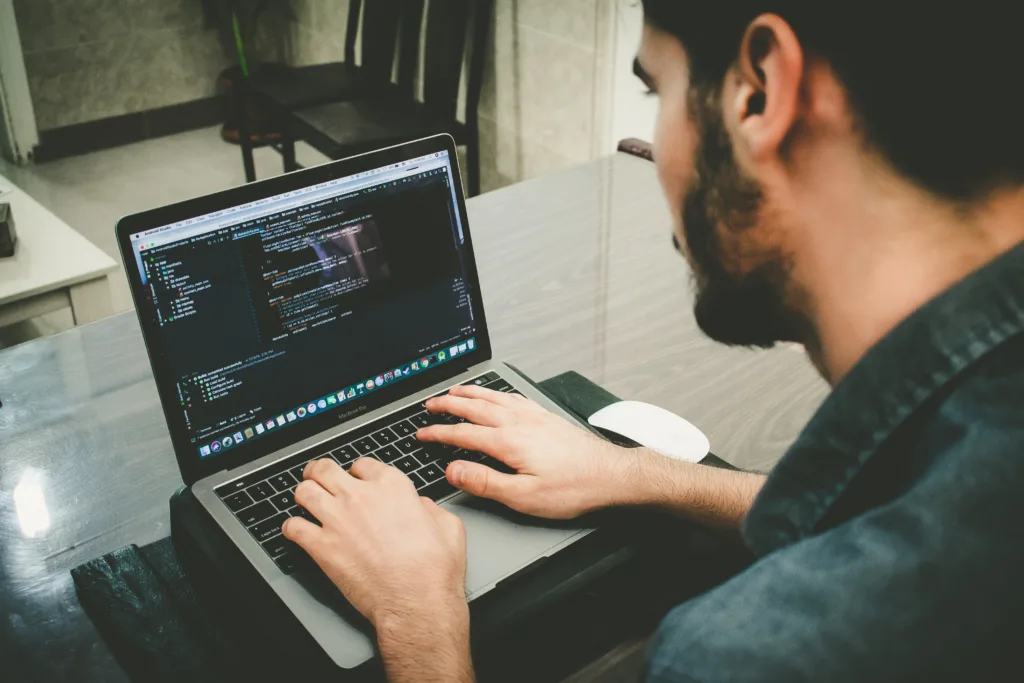
Does Fgets Include a New Line Character?
Fgets includes the newline character in the string that it reads from the input stream. This means that if the input cntains a newline character, it will be included in the string that fgets returns. The newline character is added to the end of the string, immediately after the last character read from the input stream. It is important to keep in mind that fgets reads up to and including the newline character, so the size of the string returned by fgets may be smaller than the size of the buffer used to store it. Therefore, it is important to make sure that the buffer used to store the string is large enough to accommodate the maximum size of the input that may be read.
What Does Fgets Return at the End of a Line?
When fgets reads a newline character ( ‘\n’ ) from the input stream, it stores the newline character in the buffer along with the previously read characters. Then it terminates the buffer with a null character ( ‘\0’ ) to indicate the end of the string. The returned string will include the newline character at the end, unless the end of the input stream is reached before a newline character is read. In that case, fgets will return the string without the newline character, but with the null terminating character at the end.
Does the Null Terminator Character Count?
A null terminator, also known as a null character or ‘\0’, is a special character used to mark the end of a string in programming. While it is included in the string and occupies a position in memory, it is not considered a visible character and does not contribute to the string’s content. Therefore, when calculating the length of a string using strlen(s), the null terminator is not counted as a character. In othr words, the length returned by strlen(s) does not include the null terminator. It is important to keep this in mind when working with strings in programming, as it can affect various operations and functions that rely on string length.
Difference Between Null Terminator and Null
The null terminator and null are not the same. The null terminator, also known as the null character, is a character that has a numeric value of zero and is used to mark the end of a string in programming languages. It is represented by the ASCII code 0, and its purpose is to signal to the program that the string has ended. In contrast, null is a value that represents the absence of a value or an uninitialized variable. It is a common practice to initialize variables to null in order to avoid unexpected results in programs. While they share the same numeric value of zero, null and null terminator have different meanings and usages in programming languages.
Issues with Using Fgets
The problem with fgets() function is that it reads input from the standard input stream until it reaches either the maximum number of characters specified or a newline character. However, if a newline character is not encountered, fgets() continues reading until it reaches the maximum number of characters, and then it stops reading. This can result in it reading additional characters that were not intended to be read, leading to unexpected behavior in the program. Additionally, fgets() does not remove the newline character from the input buffer, which can cause problems when reading input using othr functions such as scanf(). So, it is important to be careful when using fgets() and take necessary precautions to avoid these issues.
Does Fgets Replace Existing Strings?
Fgets() does not overwrite the string. fgets() reads a line of text from a file and stores it in the buffer provided. It stops reading when it encounters a newline character or when the maximum number of characters allowed has been reached. The newline character is also stored in the buffer. The buffer is then null-terminated, meaning that a null character is added at the end of the string to indicate the end of the string.
It is important to note that the buffer size should be large enough to accommodate the maximum length of the line that may be encountered in the file. If the buffer size is too small, fgets() will only read a portion of the line and the rest of the line will be truncated. In addition, it is important to check the return value of fgets() to determine if the end of the file has been reached or if an error has occurred whie reading the file.
Fgets() is a safe and efficient way to read text files one line at a time, without overwriting the string.
Difference Between gets() and fgets()
Both gets() and fgets() are functions used for reading string inputs in C programming language. However, there are significant differences between the two that make one a better choice over the other.
The main difference between gets() and fgets() is that the latter alows the user to specify the buffer size, whereas the former does not. In the case of gets(), the function reads characters from the standard input stream until it encounters a newline character or an end-of-file (EOF) character. The problem with gets() is that it doesn’t check the size of the buffer, which can lead to buffer overflow and security vulnerabilities. Hackers can exploit this vulnerability by sending more data than the buffer can hold, causing the program to crash or execute arbitrary code.
On the other hand, fgets() reads characters from the standard input stream and stores them in a buffer until it reaches the specified size or encounters a newline character or an EOF character, whichever comes first. This means that the user can specify the size of the buffer, preventing buffer overflow and improving the security of the program. Additionally, fgets() returns a pointer to the buffer, making it easier to manipulate the input string.
While both gets() and fgets() can be used for reading string inputs, fgets() is the better choice as it allows the user to specify the buffer size, preventing buffer overflow and improving program security.
Return Value of Fgets When File Is Empty
If a file is empty, fgets() will return NULL. This is because fgets() reads characters from the file untl it reaches the end of the file, and if the file is empty, there are no characters to read.
In general, fgets() returns NULL when it encounters an end-of-file condition or an error during the reading process. It is important to check the return value of fgets() to ensure that the reading process was successful and no errors occurred.
It is worth noting that fgets() can also return NULL if the buffer size specified is too small to hold the entire line. In this case, fgets() will read as many characters as possible into the buffer and then terminate the line with a null character.
Comparing the Safety of Fgets and Gets
When it comes to input functions in C, fgets() is considered to be a safer option than gets(). This is bcause fgets() allows you to specify the maximum number of characters to be read from the input stream, preventing buffer overflow issues that can occur with gets(). In contrast, gets() has no limit on the number of characters it reads, which can lead to memory corruption and security vulnerabilities if the input size exceeds the buffer size allocated for the input.
Furthermore, fgets() can be used to read input from both standard input and files, whereas gets() can only read from standard input. This makes fgets() a more versatile option that can be used in a wider range of applications.
If you want to read input safely in C, it is recommended to use fgets() instead of gets(). Its ability to limit input size and read from both standard input and files make it a more secure and flexible option.
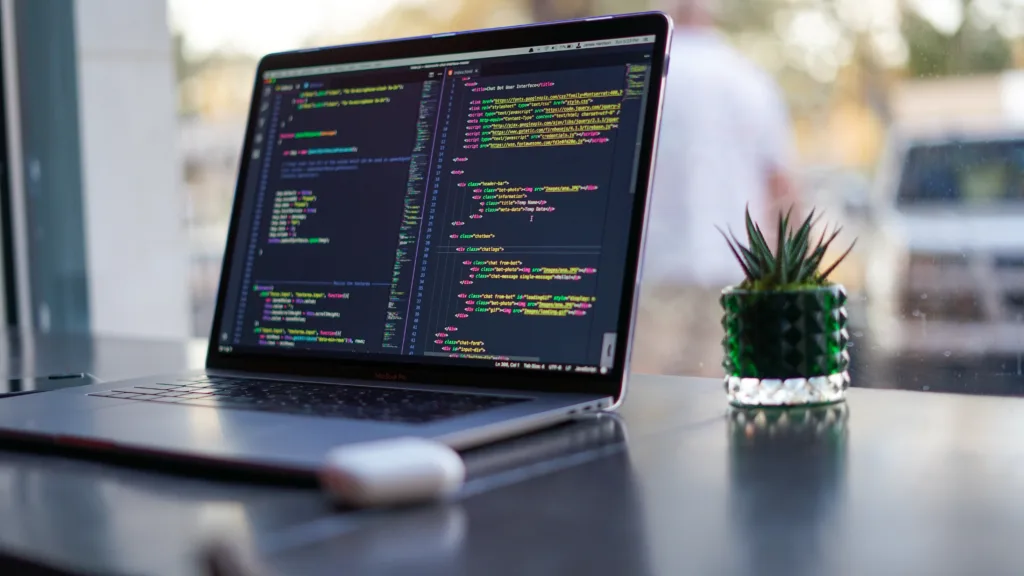
Benefits of Using Fgets() Instead of Gets()
Using fgets() instead of gets() would make the program better for several reasons.
Firstly, gets() does not check the length of the input string and can lead to buffer overflow, whih can cause the program to crash or security vulnerabilities. fgets() on the other hand, limits the input length to the size of the buffer specified, preventing buffer overflow.
Secondly, fgets() is a more secure way to read input from the user as it requires the programmer to specify the maximum size of the input buffer. This ensures that the input is not larger than what is expected and can prevent potential security issues.
Thirdly, fgets() can handle input with whitespace characters, such as spaces or tabs, whereas gets() treats these characters as terminating input. This means that if the user enters a string with spaces using gets(), only the characters before the space would be read.
Using fgets() instead of gets() makes the program safer and more robust, as it prevents buffer overflow and ensures that the input is not larger than expected.
Conclusion
Fgets is a useful function in the C programming language that allows for the reading of characters from a stream, such as a file or standard input, and storing them in a string buffer. It ensures that the string is terminated with a null character, making it suitable for use with other string functions like strlen(). Additionally, fgets has a limit on the number of characters it reads, preventing buffer overflow and enhancing security. the function is straightforward to use and provides a robust and reliable way to read input from streams in C.