Whenever you’re coding in any language, it’s important to be aware of the potential for dereferencing a null pointer. A null pointer dereference occurs when a program ties to access the data stored at a memory location that is set to NULL, often resulting in an error or crash.
Null pointer dereferencing can happen due to a variety of reasons, such as race conditions and programming oversights. It’s important to recognize that if you attempt to access an object through a null pointer, you will get an error or crash. This means that it’s essential to check all pointers for null values before trying to access them.
In languages like C and C++, there are no checks for this type of error, so it is up to the programmer to ensure that no null pointers are accessed. One way to do this is by using a language that does not allow this kind of dereferencing, such as Java. However, if you are working with C or C++ then there are some additional steps you can take.
Firstly, always check pointers for NULL before attempting to access them. If they are NULL then don’t try and use them! Secondly, make sure your variables and pointers are properly initialized and updated at all times while they’re being used in your code. Finally, if possible use functions like memset() or memcpy() instead of manually modifying the values pointed by your pointers – this can help avoid issues related with uninitialized variables or spurious values contained inside them.
All in all, it is important to be aware of the potential consequences of trying to access data through a null pointer – it can cause errors or even crashes! Make sure you take the necessary steps outlined above in order avoid any potential pitfalls associated with dereferencing null pointers when coding in languages like C and C++.
Dereferencing a Null Pointer
Dereferencing a null pointer is an error that occurs when a program attempts to access the memory address referenced by a NULL pointer. This can cause the program to crash, since the memory location being accessed is invalid. In some cases, this type of error can also lead to security breaches, as malicious code may be able to execute in the program’s address space. To prevent this type of error from occurring, it is essential for developers to properly validate pointers before attempting any operations on them.
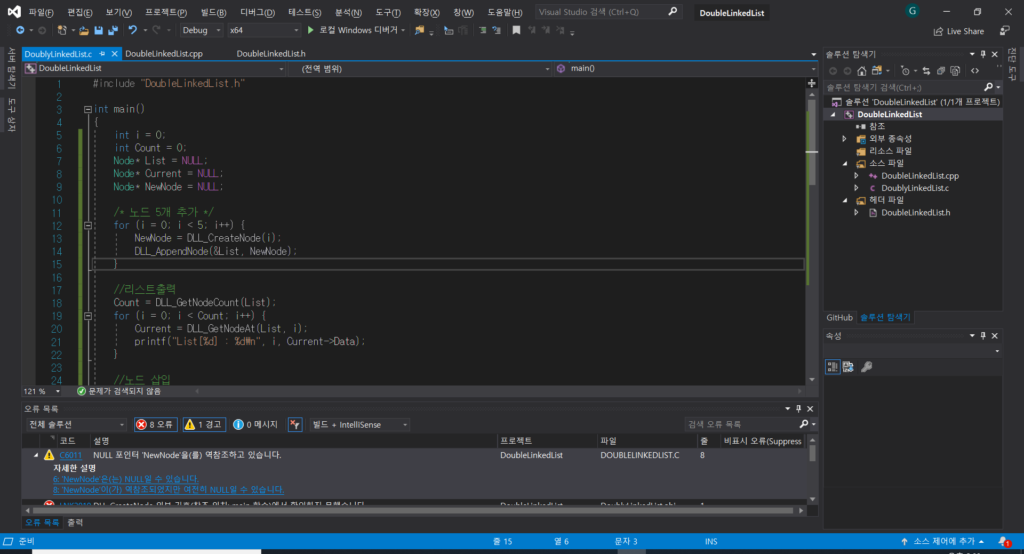
Does Dereferencing a Null Pointer Cause an Interrupt?
No, dereferencing a null pointer does not cause an interrupt. In most cases, attempting to dereference a null pointer will lead to a run-time error or program crash. Interrupts occur when an unexpected event occurs, such as an external hardware device requesting service.
Dealing with Dereferencing Null Pointers in C
The best way to solve dereferencing null pointer errors in C is to check that any pointers you are using are not NULL before attempting to dereference them. In the above code snippet, this can be seen with the two calls to the `if` statement. If either of the variables `data` or `information` is NULL, then the function will return -1 instead of attempting to dereference them. This protects against any undefined behavior that would occur if a pointer were dereferenced when it was set as NULL. It’s also important to note that if you are dealing with strings, you should always check that they have been correctly terminated by a ‘\0’ character before using them. This can be seen in the third `if` statement, where it checks if the name data in the infrmation variable has been correctly terminated before continuing with further processing.
Preventing Null Pointer Dereference
The best way to avoid null pointer dereferences is to use a language that does not allow them, such as Java. Additionally, it is important to always check pointers for NULL befre dereferencing them. If you are using a language that does allow null pointer dereferences, then you should use defensive programming techniques like checking for NULL values in your code, and have good exception handling strategies in place. Additionally, it can be helpful to use frameworks or libraries that do the checks for you. Finally, be sure to test your code thoroughly to catch any potential null pointer dereferencing errors.
Troubleshooting Null Object Reference Errors
The best way to fix an “Attempt to de-reference a null object” error is to ensure that all the sObjects in your class are properly initialized. This should be done in the constructor of your class, preferably at the beginning. It is also important to check that none of the variables have been assigned null values, as this could also lead to this type of error. Additionally, it is a good practice to double-check all of your code for any typos or errors that could result in an uninitialized object reference. If you are stll unable to resolve the issue, it may be helpful to review Salesforce’s documentation on how objects work and how they should be handled.
Benefits of Dereferencing
Dereferencing is an important concept in computer programming that allows us to manipulate data stored in memory locations that are pointed to by a pointer. By dereferencing a pointer variable, we can access the data stored at that memory location, enabling us to modify or use it in our program. This is especially useful when dealing with large and complex data structures like linked lists and binary trees, as it allows us to traverse the structure and access its components without having to know thir exact memory addresses. Dereferencing also provides an efficient way for programs to interact with memory, since it avoids the need for copying large amounts of data when accessing these structures.
Avoiding Null Dereference in Java
One of the best ways to avoid null dereferences in Java is to use the @Nullable and @NotNull annotations. These annotations can be used to indicate whether a given parameter or return value can or cannot be null, respectively. Additionally, it is important to always check for null values before attempting any operations on them, as this will prevent any errors due to trying to access properties or methods on a null object. Finally, when dealing with Strings, it is best practice to use the equals() and equalsIgnoreCase() methods with String literals instead of an unknown object that could potentially be null.
Dereferencing an Uninitialized Pointer
If you dereference an uninitialized pointer, you are essentially telling the computer to access a memory address that it doesn’t know about. This can cause unpredictable results, and in some cases, can even lead to a program crash or system error. In addition, if the memory address that is being accessed is read-protected, then an exception may occur as the computer will not be able to read from it. It’s important to note that comparing uninitialized pointers often leads to undefined behavior, so it’s best to avoid doing so.
Dereferencing a Pointer Before Assigning a Target
If you try to dereference a pointer before it has been assigned a valid target, your program will experience an error called a “segmentation fault” and will likely crash. This is because the pointer does not have any information about where it should be pointing to in memory, so trying to access the data at that location is undefined behavior and can lead to unpredictable results. To avoid this situation, always make sure that your pointer has been assigned a valid target before attempting to dereference it.
Reassigning a Null Pointer
Yes, you can reassign a null pointer. A null pointer is simply a pointer that has no value assigned to it, meaning it does not point to any memory address. When you reassign a null pointer, the original value of the pointer is lost and the new value will be assigned. This means that the pointer no longer has access to whatever data it previously pointed to, so it is important to consider this when reassigning a null pointer.
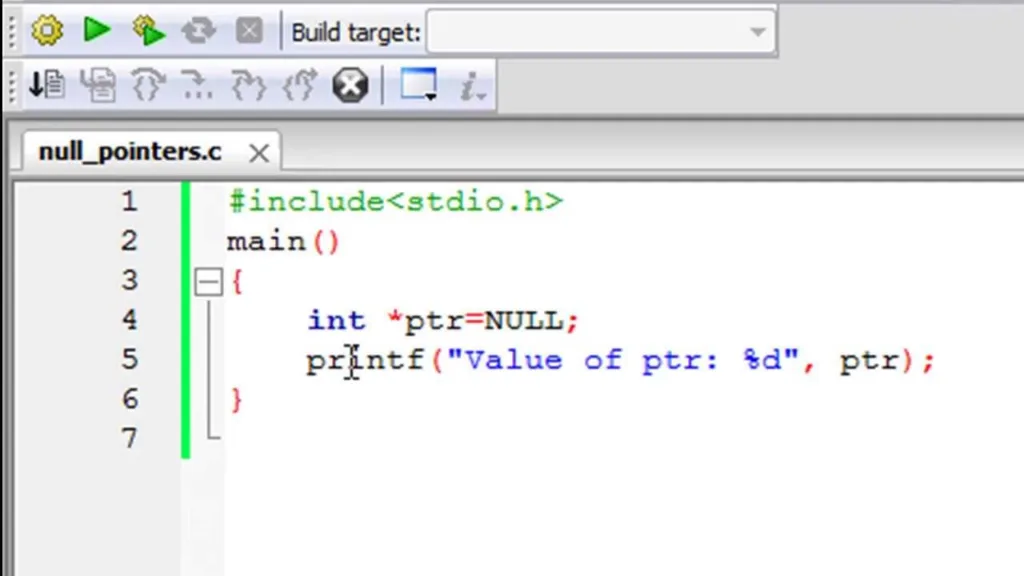
The Cost of Pointer Dereferencing
The answer to this question depends on the context. Generally speaking, pointer dereferencing is not an expensive operation in terms of time or memory usage. The actual cost of dereferencing a pointer will depend on the size of the object being accessed and the number of indirections that need to be made in order to reach it. For example, accessing a single member of a class through a pointer is typically cheap, but navigating a complex data structure with multiple levels of indirection can be more costly. Additionally, some architectures may have optimized instruction sets for quickly accessing objects pointed to by pointers, wich can further reduce the cost associated with dereferencing.
Using the Dereference Operator
The unary operator & is used for dereferencing a pointer. This means that it takes the address of an object and returns the value stored at that address, rather than the address itself. By dereferencing a pointer, you can access the data stored at its memory location. It is important to note that when using the & operator, you must always dereference a pointer; attempting to use it on any other type of data will result in an error.
Dereferencing in Java
Dereferencing in Java is a process of accessing an object’s features through its reference. A reference in Java is the address of the object, and dereferencing involves using that address to access the object’s properties and methods. Primitives, however, cannot be dereferenced as they do not have any associated references. Attempting to dereference a primitive will result in an error indicating that it cannot be dereferenced.
Conclusion
In conclusion, a null pointer dereference is an issue that can cause a program to crash or exit unexpectedly. It is important to clearly understand the consequences of dereferencing a null pointer and take steps to avoid it, such as using languages that do not allow them or always checking pointers for NULL before dereferencing them. Doing so can help ensure programs are more reliable and secure.