The default boolean value in Java is an important concept to understand, as it is used in a variety of ways in the programming language. Boolean values are used to represent the truth of a statement and are often used to control program flow. Knowing what the default boolean value is can help you write more efficient and accurate code.
In Java, the default boolean value is false. This means that if no other value is assigned to a boolean variable, then it will evaluate to false by default. This behavior can be overridden by explicitly assigning a different value (true or false) to the variable. It’s important to note that this default behavior only applies to variables declared as booleans; for any other data type, the default value will depend on that type’s individual rules.
Boolean variables are stored as 16-bit (2-byte) values, which means they take up less memory than other types of data such as integers and strings. Because of this, booleans can be used frequently in programs without taking up too much memory space. This makes them partiularly useful for controlling complex logic within your code – for example, you could use them to check whether certain conditions have been met before executing certain lines of code.
It’s also worth noting that there are some cases where the default boolean value may not be what you expect it to be – for example, when dealing with “null” references (which have no value). In these cases, it’s important to understand how null references behave so that you don’t accidentally assign an unexpected result when using them with boolean variables.
Overall, understanding how the default boolean value works in Java can help make your code more efficient and reliable. Knowing when and how to override it can also help you avoid potential errors and save time during development.
Setting Default Values for Booleans in Java
In Java, you can set the default value for a boolean by declaring it with either the true or false keyword. For example: boolean myBoolean = false; This would set the default value of myBoolean to false. You can also set a default value for a Boolean object by using the Boolean class’ constructor. For example: Boolean myObject = new Boolean(false); This would set the default value of myObject to false. If you do not specify a value when declaring or constructing your Boolean, it will be initialized to null.
Source: javahungry.blogspot.com
Default Value of Boolean
The default value of the bool type is false. A boolean, often referred to as a “bool,” is a data type used in programming languages that can be either true or false. The default value for a boolean variable is false, meaning that if no value is explicitly assigned to the boolean then its value will remain false.
Default Values in Java
Default values in Java refer to the values assigned to a variable when it is declared, but no value is explicitly assigned. For instance, if an integer variable is declared as int num; then its default value wold be 0. Similarly, if a boolean variable was declared without any value being assigned, like boolean flag; then its default value would be false.
When it comes to primitive data types in Java, the following default values are assigned: float – 0.0, double – 0.0, boolean – false, char – \u0000′ or null. For reference types such as objects and arrays, their default value is null.
It’s important to note that these default values can be overridden by assigning a specific value to the variables while they are declared.
Default Boolean Size
The default boolean size is 16 bits, or 2 bytes. This means that each boolean value (either True or False) is stored in two bytes of memory. This is the same for most programming languages, so it’s important to be aware of this when designing a program.
Initializing Boolean Variables in Java
In Java, boolean variables are declared using the keyword ‘boolean’. For example, a variable named ‘flag’ can be initialized as follows:
boolean flag = false;
The boolean variable is initialized to either true or false. It is important to note that booleans cannot be assigned any other value than true or false.
Source: twitter.com
Setting Default Values
To set a default value in a database field, you need to first identify the data type of the field. The data type determines what types of values are allowed for that field. After selecting the approprite data type, you can enter your desired default value in the “Default Value” box. For example, if your field is of type Date/Time, you can enter =Date() to insert the current date as the default value.
If you want to set a default value for a Text or Number field, you can simply enter the desired value into the “Default Value” box. You may also use expressions and functions when entering text or number values. For example, if you want to set a default string such as “Hello World”, you can use =”Hello World”.
Once you have entered your desired default value, remember to save your changes.
Is a Bool Always 0 or 1?
No, bool is not always 0 or 1. The bool type is a type of data that can only represent two values: true or false. When a bool is cast into an integer, true becomes 1 and false becomes 0; however, the bool type itself does not necessarily have to be represented as a 0 or 1.
Is the Boolean Value True Represented as 1 or 0?
No, bool true is not 1 or 0. The boolean data type is a special type of data that can only have two values: true or false. In programming languages, these values are usually represented as 1 (for true) and 0 (for false). However, it is still best practice to use the words true and false when writing code, rather than using the numbers 1 and 0.
Is Boolean a True or False Value?
A boolean value is either True or False. The Tableau INT() function converts a boolean to a number, returning 1 for True and 0 for False. This means that a boolean value can be either True or False, or it can be represented as 1 or 0 depending on the context of the situation.
Source: youtube.com
What Does the Unicode Character \u0000 Represent in Java?
\u0000 in Java is a Unicode character that represents the null (or 0) value. It is the minimum value that a char data type can hold. This symbol is used to indicate the absence of any other valid character or value in a program, making it particularly useful when dealing with strings and other data manipulation.
Finding the Default Value in Java
To find the default value in Java, you can use the getDefaultValue() method from the java.lang.reflect.Method class. This method returns the default value of an annotation member that is represented by a Method object. For example, if you have a method called “myMethod” in your application, you can retrieve its default value using this method:
MyObject myObject = new MyObject();
Method myMethod = myObject.getClass().getDeclaredMethod(“myMethod”);
Object defaultValue = myMethod.getDefaultValue();
In this example, we create an instance of MyObject and then retrieve the declared Method object for our method called “myMethod”. Finally, we use the getDefaultValue() method to retrieve its default value as an Object type which can then be cast into any other type depending on the type of data stored in it.
The getDefaultValue() method is useful when we want to access annotation members with their default values and not just their actual values in our application code. It can also be used to check whether a partcular annotation member has been set or not in our code and provide appropriate logic accordingly.
Setting Default Values in Java
Setting default values in Java is a very simple task. All you have to do is assign the desired values to the variables you wish to set as default. For example, if you have a boolean variable called val1, and you want it to have a default value of false, all you need to do is assign val1=false;. Similarly for oter data types such as int, long, float, double and String, the syntax would be = . For example if you want to set a default value for a long variable called val5, the syntax would be long val5 = 0L;. This way by assigning different values to different variables depending on their data type, we can easily set default values in Java.
Understanding Boolean Values in Java
Boolean value in Java is a primitive data type used to store only two possible values: true or false. It is mainly used to evaluate a condition, such as in an “if” statement. Boolean values are typically stored in variables and can also be used with methods. By default, all boolean values are initialized to false. As it is 1-bit information, the precise size of it cannot be defined.
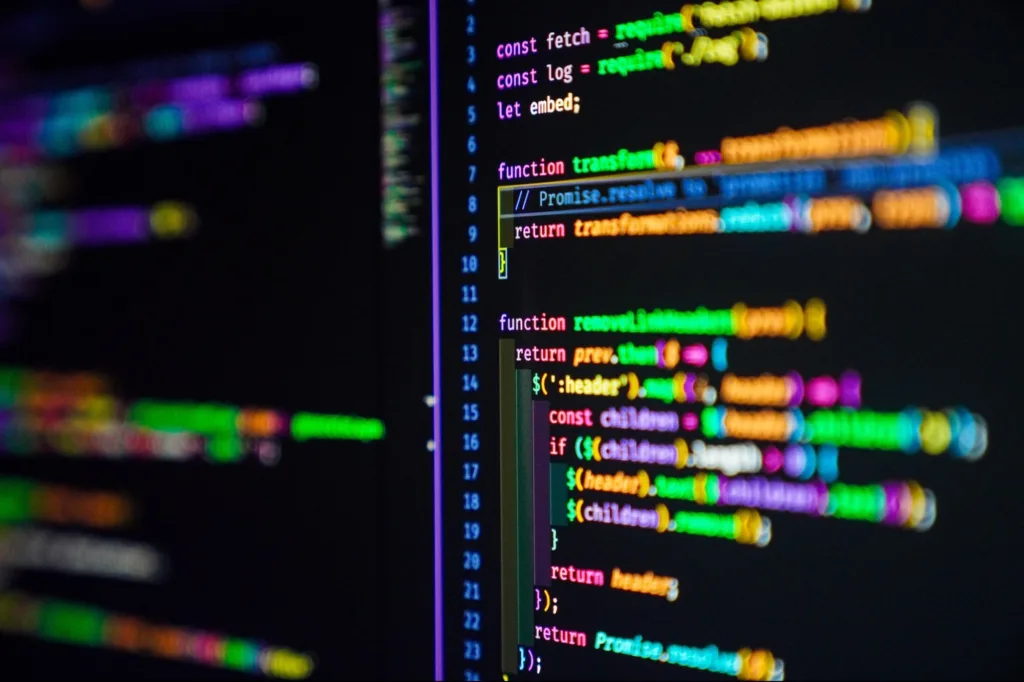
Size of a Java Boolean
A Java Boolean is a primitive data type of size 1 bit. It is used to store the values true and false, which are represented as 1 and 0, respectively. Booleans can be used to represent a binary choice (yes/no) or a logical operation (AND/OR). They are usually used in if-then statements, loops, and other control structures where they can be evaluated to true or false. In Java, booleans are also objects of the class java.lang.Boolean and can be manipulated using methods like booleanValue() and booleanEquals().
Is 0 a Boolean Value?
No, 0 is not considered to be true or false in the traditional sense, but rather a special case. In programming languages and other logical contexts, 0 is usually considered to be false while all other numbers are usually considered to be true. This means that when a comparison between two values is made, if one of the values is 0 then the comparison will evaluate as false. However, this should not be confused with the idea that 0 itself is false; it simply serves as a marker for the evaluation of the comparison being made.
Conclusion
In conclusion, the default value for boolean in Java is false. This is because boolean variables are stored as 16-bit (2-byte) values and can either be True or False. Primitive data types such as Float, Double and Char also have default values assigned to them. It is important to note that if a Boolean object is unintialized, it will point to null instead of a default value.