Today, we are going to be discussing the concept of epoch time in Python. Epoch time is a way of measuring time and is used by computer systems to keep track of time.
Epoch time is measured from the Unix epoch, which is January 1, 1970 at 00:00:00 UTC (Coordinated Universal Time). In Python, you can get the current epoch time using the gmtime(0) function, which returns the current epoch time as a tuple of nine integers with each element representing a different part of the date/time. For example, the first element of this tuple is the year, while the second and third elements are month and day respectively.
You can also use the %%time or %time magic commands in IPython to get more detailed information on how much CPU resources were used when running a particlar command. It prints out two values: CPU Times, which gives you information on how much user and system CPU resource was used to run your command.
Finally, you can use datetime.now() to get an accurate timestamp for any given moment in time. The timetuple() function in the datetime class returns a named tuple with all its properties such as year, month, day etc., which makes it easy for you to manipulate certain aspects if needed.
In conclusion, understanding how epoch time works and what functions are available for getting current timestamps in Python will be useful when dealing with tasks related to managing data over long periods of time or when searching data from specific periods in history.
Understanding Epoch Time in Python
Epoch time, also known as Unix time or POSIX time, is a system for tracking time in computers that measures the number of seconds since January 1, 1970 at 00:00:00 UTC. In Python, this is represented by the gmtime() function which takes a single argument timestamp and returns an object representing the current date and time in UTC. The gmtime() function can be used to convert any given timestamp into a readable date and time format. Furthermore, the time() function can be used to convert any given date and time into its coresponding timestamp. This makes epoch time an incredibly useful tool when dealing with dates and times in Python programs.
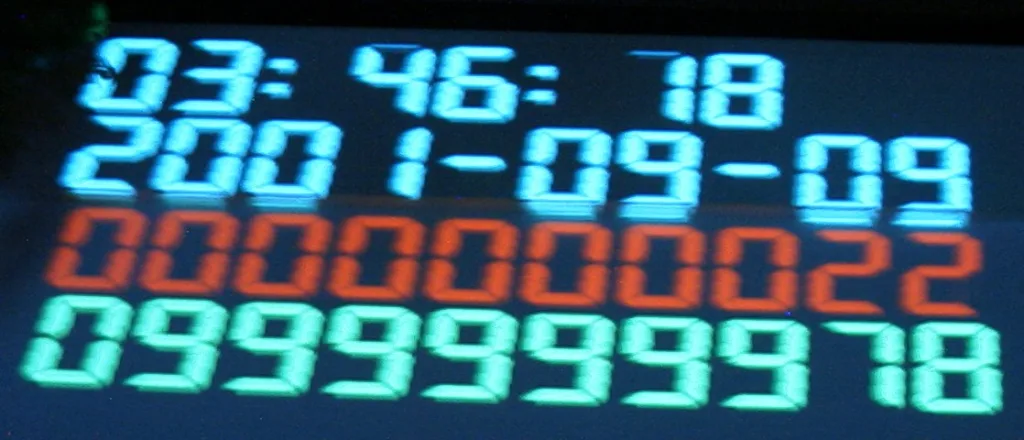
Understanding the Functionality of ‘%% Time’ in Python
%%time is a magic command in Python that allows you to measure the amount of time it takes for a certain code block or statement to execute. It prints out two values: the total CPU times spent on executing the code and the system time used by other processes running concurrently. This makes it an invaluable tool for optimizing your code and finding bottlenecks in long-running scripts. It can also be used to compare different approaches to solving a problem, helping you identify which one is more efficient.
Retrieving the Current Unix Timestamp in Python
In Python, you can get the current Unix timestamp by using the time module. The time module provdes functions for manipulating timestamps, including a function to get the current Unix timestamp. To get the current Unix timestamp, you can use the time() function from the time module. This will return a float representing the number of seconds since 1 January 1970 (the Unix epoch). For example:
import time
now = time.time()
print(now)
This will print out a float representing the current Unix timestamp in Python. You can also use datetime objects to get the current Unix timestamp. If you have a datetime object, you can call its timetuple() method to retrieve a tuple of values representing its properties in Unix time format. For example:
from datetime import datetime
now = datetime.now()
unix_timestamp = now.timetuple()
print(unix_timestamp)
This will print out a tuple of values representing the current date and time in Unix format.
Getting the Current UTC Time in Python
To get the current UTC time in Python, you can use the datetime module’s datetime.utcnow() function. This will return a datetime object representing the current UTC time. To get the timestamp of the current UTC time, you can use the datetime.timestamp() method, which will return a float value representing the number of seconds since the Unix epoch (January 1st, 1970).
Calculating Epoch Time
Epoch time is the number of seconds that have elapsed since January 1, 1970 (midnight UTC/GMT). It can be calculated by subtracting the date/time of interest from the epoch date/time and then converting the difference to seconds. For example, if you wanted to calculate the epoch time for April 1, 2021 at 12:00 PM UTC, you would first subtract January 1, 1970 from April 1, 2021 to get 17,945 days. Then you would multiply this by 86400 (the number of seconds in a day) to get 1,553,168,000 (17,945 x 86400). This is the epoch time for April 1st 2021 at 12:00 PM UTC.
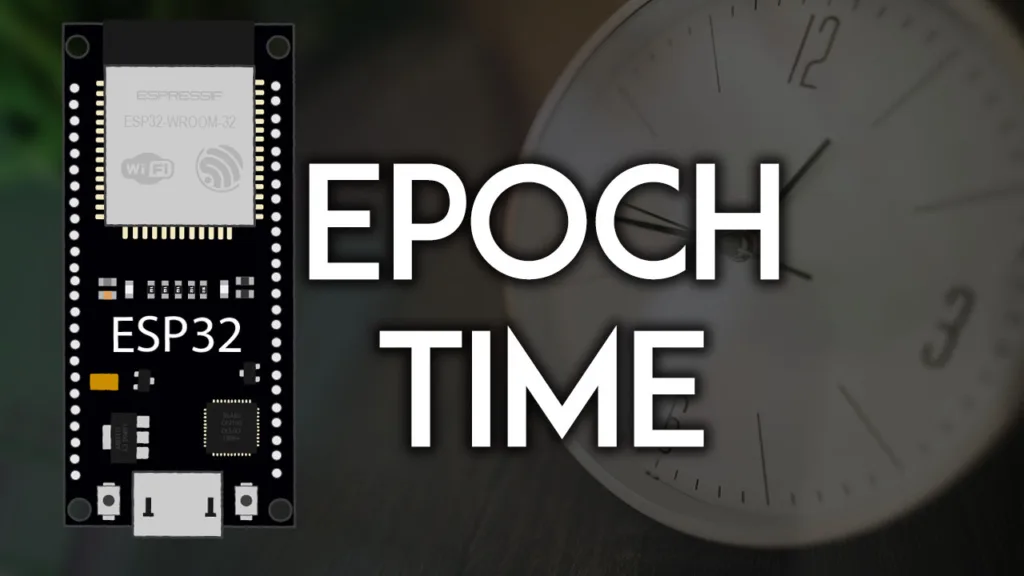
Printing the Current Time in Python
Printing the current time in Python can be done using the datetime module. The datetime.now() function returns the current local date and time as a datetime object, which can then be printed using the strftime() function. The syntax for printing the current time is as follows:
import datetime
# Get the current date and time
current_time = datetime.datetime.now()
# Print today’s date and time
print(current_time.strftime(“%d/%m/%Y %H:%M:%S”))
Checking Time Format in Python
In Python, you can check the time format using the strftime() function. This function takes a format string as an argument, whre each character in the string is a directive for the outputted time string. For example, if you wanted to output the current date and time in a specific format, such as “DD-MM-YYYY HH:MM:SS” you could use the following code:
import time
time_str = time.strftime(“%d-%m-%Y %H:%M:%S”)
print(time_str)
This will output something similar to “28-03-2020 21:32:18”. This is just one example of how you can use strftime() to customize your date/time strings. You can find more information about this function and all its directives in Python’s official documentation.
What Data Type Does the Time() Function Return?
The time() function returns an integer representing the number of seconds since the epoch, which is defined as the start of January 1st, 1970 in UTC. The return value is a long integer that is equivalent to the number of seconds since the epoch. As such, it represents a global moment in time and can be used to calculate elapsed time or compare different timestamps.
Is Python’s Time Module Based on UTC?
Yes, Python’s time method time() returns the time in UTC (Coordinated Universal Time). This means that the returned value is the number of seconds since 00:00:00 UTC on January 1, 1970. It is important to remember that UTC is different from GMT, and does not have daylight savings time adjustments.
Converting Datetime to Epoch Time in Python
To get epoch time from datetime in Python, you can use the strftime() method. This method takes an argument of the format string representing the desired output and returns a string representing the epoch time. You can also pass a datetime object as an argument, wich will return its equivalent epoch time. Here is an example:
import datetime
dt = datetime.datetime(2020, 1, 1)
epoch_time = dt.strftime(‘%s’) # 1577836800
The %s format specifier is used to convert a valid date and time representation into an epoch value (the number of seconds since January 1st 1970). The result will be an integer representing the specified point in time.
You can also specify other format specifiers such as %Y for year (e.g., 2020), %m for month (e.g., 01 for January), %d for day (e.g., 01 for first day of the month), etc., depending on your requirements.
Getting Unix Epoch in Python
Getting the Unix epoch in Python is quite simple. First, you’ll need to import the time module. This can be done with the following line of code:
import time
Once imported, you can access a function called time.time(). This function returns the current Unix epoch, expressed as the number of seconds since January 1st 1970 (the beginning of the Unix epoch). Therefore, if you simply call this function with no arguments like so:
unix_epoch = time.time()
you will have the current Unix epoch stored in a variable called unix_epoch.
Reading Timestamps in Python
Reading a timestamp in Python is relatively easy. The first step is to import the datetime module, which cntains functions for manipulating dates and times. Once imported, you can use the fromtimestamp() function to convert a timestamp into a readable date format. This function takes a single argument, which is an integer representing the timestamp you want to read. It then returns an instance of the datetime class with attributes for the year, month, day, hour, minute, second and microsecond of the specified timestamp. You can then access these values directly or use other methods in the datetime module to manipulate them further.
Fetching UTC Time
Fetching UTC time in Java can be achieved through several different methods. The most straightforward way to do this is by using the SimpleDateFormat class, which provides a wide range of date and time utilities. The format for getting the current UTC time is as follows:
SimpleDateFormat dateFormat = new SimpleDateFormat(“yyyy-MM-dd’T’HH:mm:ss.SSS’Z'”);
dateFormat.setTimeZone(TimeZone.getTimeZone(“UTC”));
String utcTime = dateFormat.format(new Date());
System.out.println(“UTC Time : ” + utcTime);
Another way to fetch UTC time is with Java 8’s Instant class, which provides a very precise representation of an arbitrary timestamp, including nanoseconds if necessary. To get the current UTC time using Instant, the code would look like this:
Instant nowUtc = Instant.now();
System.out.println(“UTC Time : ” + nowUtc);
Finally, you can also use Java 8’s OffsetDateTime class to get the current UTC time, which is especially useul when dealing with Date and Time values that are in different timezones than your own system’s default zone:
OffsetDateTime offsetDt = OffsetDateTime.now( ZoneOffset.UTC ); System.out.println(“UTC Time : ” + offsetDt);
Additionally, you can also use Joda-Time library to fetch UTC times in Java; however, it has been largely superseded by the new Date and Time API in Java 8 and subsequent versions of the language, so it is best used only for legacy code or when interoperability with existing Joda-Time code is required.
Converting Epoch Time to UTC in Python
Converting epoch time to UTC in Python is a simple process that requires the use of the datetime module. First, import the datetime module using ‘import datetime’. Then you can use the fromtimestamp() function to convert an epoch time into a UTC-based timestamp. For example, if you have an epoch time of 1549784800, you can convert it to UTC by using: utc_timestamp = datetime.datetime.fromtimestamp(1549784800). The result will be a timestamp in UTC format, such as 2019-02-08 11:00:00.
Conclusion
In conclusion, the current epoch time in Python is January 1, 1970, 00:00:00 (UTC). This date and time is used as a reference point for all other dates and times in the Python language. The timetuple() function of the datetime class can be used to access the current epoch time in Python, as well as to get the current date and time. Furthermore, IPython’s %%time or %time magic command can be used to get CPU times.