Static variables are variables that retain their values throughout the lifetime of a program. They are declared using the keyword ‘static’ and can be used to store data that is not specific to any particular instance of a class or object. Static variables are shared amongst all instances of an object, making them useful for storing data that is common to all instances.
Unlike regular variables, static variables can be changed at any time during the execution of the program. This makes them ideal for storing data that needs to be accessible throughout the entire lifespan of a program, such as configuration settings or program state information. In addition, they can be used by other classes and objects in order to share data with each other.
One important note about static variables is that they must be initialized before they can be used in a program. If a static variable is not initialized it will have an undefined value and may cause unexpected behavior in your code. For example, if you declare a static integer but do not initialize it, it will default to 0 when accessed from anywhere in your code.
In summary, static variables are powerful tools for storing data that needs to persist throughout the lifetime of a program. They are also great for sharing data betwen objects and classes without having to use global scope variables or pass information directly between objects. Just be sure to always initialize your static variables before using them in order to avoid unexpected behavior!
Changing the Value of a Static Variable
Yes, the value of a static variable can be changed. A static variable is defined at the class level and is shared by all instances of that class. It can be accessed using the class name itself, without creating any instance of the class. Its value can be changed within its scope, or from outside its scope using the class name. However, it is important to note that changing the value of a static variable affects all other instances of that class, so care must be taken when doing so.
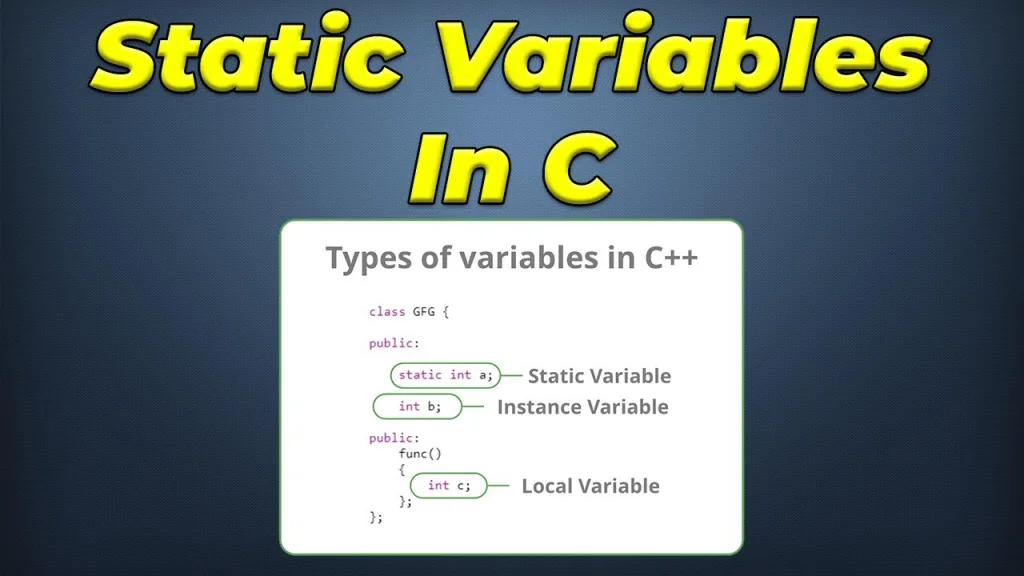
Can Static Variables be Changed in C?
No, a static variable in C cannot be changed. A static variable is allocated for the lifetime of the program, and its value remains the same throughout that program’s execution. Once it has been declared as static, it cannot be modified again, which means that any changes to its value will not be reflected in subsequent code.
Can Static Variables be Overridden?
No, static variables cannot be overridden. Static variables are associated with a class and not with an object, so they are shared by all the objects of a class. They are initialized only once when the class is loaded into memory. When a static variable is modified, the changes are reflected in all instances of the class. Therefore, it is not possible to override static variables as they cannot be changed or overridden on a per-instance basis.
The Permanence of Static Variables
Yes, static variables are permanent. They are allocated in memory when the program first starts and remain there until the program exits. Unlike other variables, which may be created and destroyed as needed during program execution, static variables exist for the entire life of the program. This makes them a useful tool for storing long-term information or data that needs to persist throughout the lifecycle of the application.
Do Static Variables Remain Constant?
Yes, static variables stay the same throughout the life cycle of the program. Static variables are allocated in a fixed block of memory and will persist until the program terminates. They are used to retain their value even after function calls, loop iterations or any other kind of code execution. This makes them extremely useful for keeping track of information that needs to be maintained across different parts of a program. In addition, since static variables stay the same throughout the life cycle of a program, they are easy to deal with for the memory system.
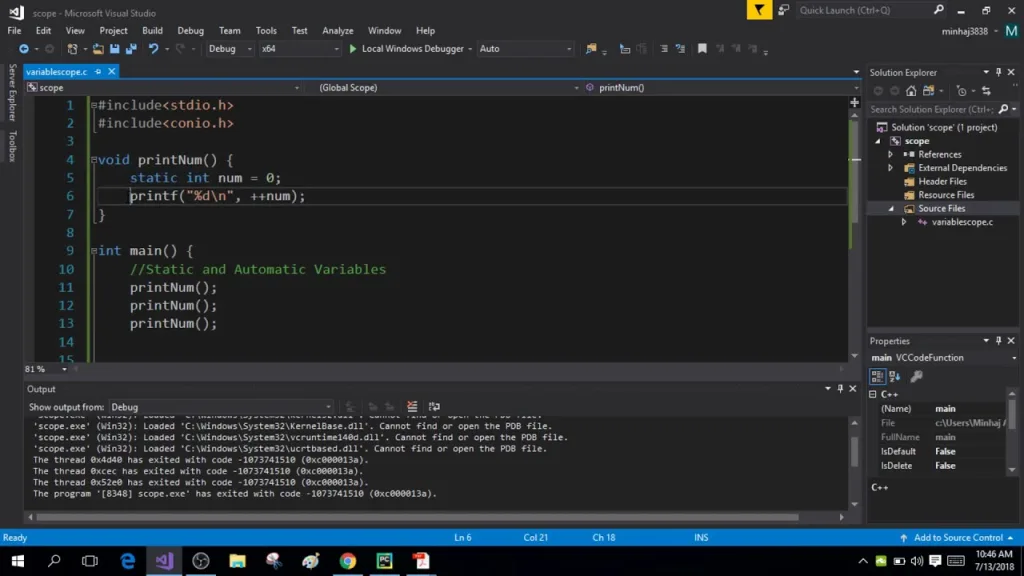
Do Static Variables Require Memory Deallocation?
No, static variables do not need to be freed. They exist throughout the program’s lifetime and do not require any manual memory management like dynamic variables. Static variables have local scope, meaning they are only accessible within the function in which they are declared.
Initializing Static Variables
Yes, you can initialize static variables. When a static variable is declared, it is initialized with a default value, depending on its data type. For example, an integer type static variable will be set to 0; a pointer type static variable will be set to NULL; and a character type static variable will be set to the null character ‘\0’. You can also explicitly initialize static variables by providing an initializer value in the declaration. This initializer value will be assigned to the static variable whenever the program is loaded into memory. The assignment of this initializer value happens only once during program execution and remains unchanged throuhout its lifetime.
Clearing Static Variables in C
In order to clear a static variable in C, you need to reset its value. This can be done by assigning it to some other value or by calling the memset() function. If you want the variable to remain static, then you must remember to reset it each time your program is closed and re-opened. Alternatively, you could pass the address of your static variable into a structure that is passed by reference or pointer and then reset it when necessary.
Incrementing Static Variables
Yes, we can increment static variables. A static variable is a variable that is declared using the static keyword and is shared acrss all instances of a class. It can be incremented within any instance of the class or in a static method of the class. For example, if we have a Counter class with a static variable named ‘count’ that holds an integer value, we can increment this value by calling ‘count++’ from within any instance of the class or from within a static method. This will increase the value of count by one, and all instances of the Counter class will share this same incremented value.
Can Static Variables Be Overridden in Java?
No, you cannot override a static variable in Java. A static variable is associated with the class in which it is declared and not with any particular instance of that class. Consequently, all instances of the class share the same static variable and have the same value for that variable. This means that only one copy of a static variable exists at any given time, so it cannot be overridden. Additionally, like all other variables in Java, a static variable must be assigned a value when it is declared; this value cannot be changed afterward.
Clearing Static Variables in Java
Static variables are class-level variables, meaning they are assocated with the entire class and not any single instance of the class. As a result, static variables can only be cleared or reset by restarting the program. It is not possible to clear or reset the value of a static variable within the program itself.
In some cases, it might be beneficial to set a static variable back to its initial state within the program. This can be accomplished by defining a static method that resets the value of all static variables back to their default values. After calling this method, all static variables will have their original values restored.
Changing Static Values in Java
Changing a static value in Java is reltively straightforward. To do so, you need to create a static method. A static method is one that can be accessed without the need for an instance of the class. This makes it easy to modify a static value quickly and uniformly across all instances of the class.
To create a static method, you need to declare it using the keyword “static” before the return type. Inside this method, you can then change the value of any static field of that particular class.
For example, let’s say we have a Student class with fields such as rollno, name, and college (which is marked as “static”). To change the value of college, we can create a new static method called “change()”:
static void change(){
college = “”BBDIT””;
}
Now whenever this method is called from anywhere within our program, it will update the value of college to “BBDIT”.
It’s important to note that when creating a static method and changing values within it, you must use proper synchronization techniques or else multiple threads could access and update those values simultaneously which might lead to unexpected results.
Lifespan of Static Variables
Static variables last for the entire duration of the program. They are allocated memory only once, when the program is first loaded, and they exist until the program terminates. Unlike other variables, static variables do not get destroyed when they go out of scope or when a function returns; instead, they remain in memory and can be accessed by any other functions or objects within the program. The lifetime of a static variable thus corresponds to that of the program itself.
Storage of Static Variables
Yes, static variables are stored in memory. They are stored in the data segment of the virtual address space of a program. Static variables that do not have an explicit initialization or are initialized to zero are stored in the uninitialized data segment (also known as the BSS segment). The data segment is used to store all global and static variables which can be accessed by any function in the program. In addition, each variable is stored separately, so they can be changed independently from each other.
Conclusion
In conclusion, static variables are variables that remain constant for the lifetime of the program and canot be changed or modified. Static functions are invoked directly by using a class name and can be called from anywhere in the program. Additionally, static variables are initialized only once and cannot be overridden as method overriding depends on dynamic binding at runtime whereas static methods are bonded using static binding at compile-time. Generally, static variables exist for the entire life of the program, beginning when the program starts and ending when the program exits. As such, they are beneficial in embedded systems where they always exist unless the power is removed.