Are you looking for an easy way to print double values in C? If so, then you’ve come to the right place! In this blog post, we’ll discuss how to use the printf() function to print double values in C.
Start by understanding that printf() is a standard library function that allows us to print data. It is commonly used for printing strings, characters and even integers. For printing double values, we need to use a special formatting specifier called %f. This tells the printf() function that we are going to be printing a double value.
Now let’s look at some examples of using the %f specifier when printing double values. Suppose you want to print out the vaue of Pi (3.1415926535). You can do this with the following code:
printf(“Pi is equal to %f”, 3.1415926535);
In this example, we have used the %f specifier and passed in the value of Pi as an argument. This will print out “Pi is equal to 3.1415926535” on your screen.
We can also specify how many decimal places we want our double value printed with by adding a number before the “f” in our formatting specifier. For example, if we want only two decimal places printed out for Pi, then we can use:
printf(“Pi is equal to %2f”, 3.1415926535);
This will print out “Pi is equal to 3.14” on your screen instead of all 8 digits after the decimal point as before.
So far, we have seen how to use printf() for printing basic double values with different numbers of decimal places specified in our format string (the text inside quotation marks). But what if you wanted to also specify other formatting options such as left/right justification or field width? You can do this by adding additional characters before or after your formatting specifier:
• To justify left/right add a minus (-) sign before your format specifier;
• To specify field width add a number after your format specifier;
For example, suppose you wanted your output string for Pi above justified right and occupying 5 characters total:
printf(“Pi =%5f”, 3.1415926535); // “Pi = 3.14”
Here, we have added a 5 after our format specifier which tells printf() that it must occupy 5 characters in its output string (including any spaces required). Also note that since no minus (-) sign was added before our format specifier, it defaults to left justification which means it will fill up any extra space with spaces on its left side (i.e., making our output string “Pi = 3.14”).
We hope this blog post has been helpful in showing you how easy it is to print double values using printf() in C!
Printf %2d in C
Printf %2d in C is a format specifier for an integer data type. It tells the printf function to print an integer in the output console with two characters of space, no matter how many digits the variable holds. If the number of digits of the variable is larger than two, then it will print without any spaces. For example if you have a variable n= 18999 and use printf(“%2d”,n) it will reserve 2 characters and print 18999 without any spaces.
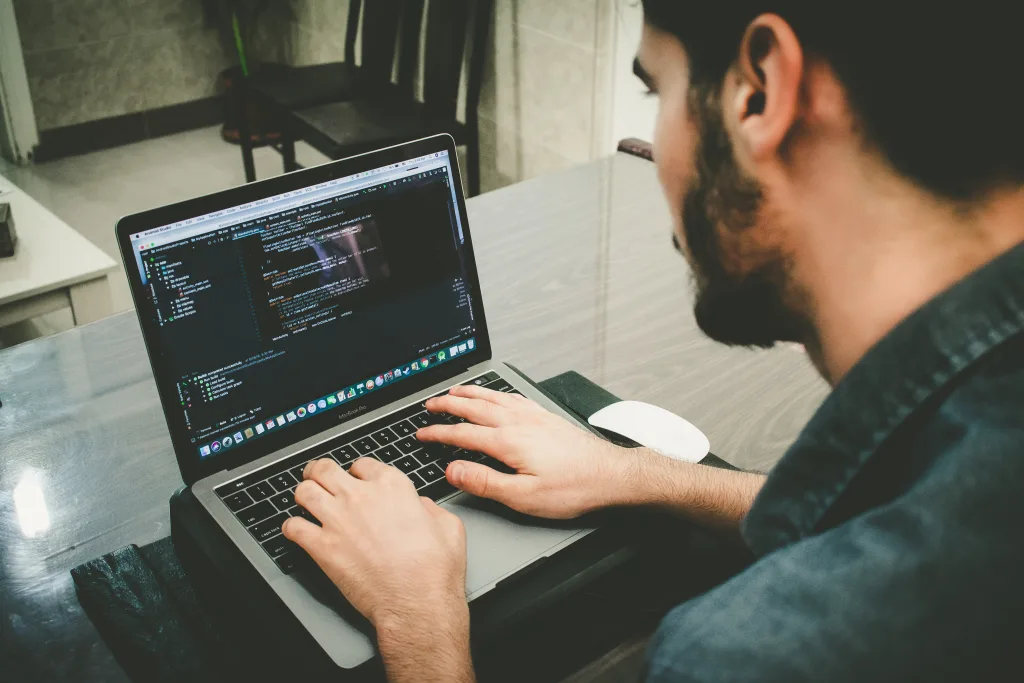
Is Double a Data Type in C?
No, %d is not used for double in C. %d is used for decimal values of type int or some other smaller signed integer type that gets promoted to int. For floating-point types, such as double, you should use %f.
Printing Double Values with Two Decimal Places in C
In order to print a double with 2 decimal places in C, you can use the printf() method and pass in the formatting specifier “%.2f”. This will tell printf to output a floating point vaue (the double) rounded to 2 decimal places. For example, if you have a double x that you wish to print with two decimal places, you can use the following code:
printf(“%.2f”, x);
This will output your double x with two decimal places. If you want to specify more than two decimal places, simply change the format specifier accordingly – for three decimal places use “%.3f”, for four use “%.4f” and so on.
Printing Double Values
In order to print double-sided, you’ll need to use the manual duplex option on your printer. To do this, begin by opening the document you’d like to print in Microsoft Word. Then, click the File tab and select Print. Under Settings, you’ll then see an option to Print One Sided – click this and select Manually Print on Both Sides. When you click print, Word will prompt you to turn over the stack of paper so that it can feed it into your printer again. After this is done, your document will be printed double-sided!
Using %5d in C Programming
%5d in C is used for formatting the output of an integer. It tells the compiler to add left padding with spaces until the total number of characters (including digits and spaces) is five. This is useful for creating uniform tables in the console, so that each row has the same width.
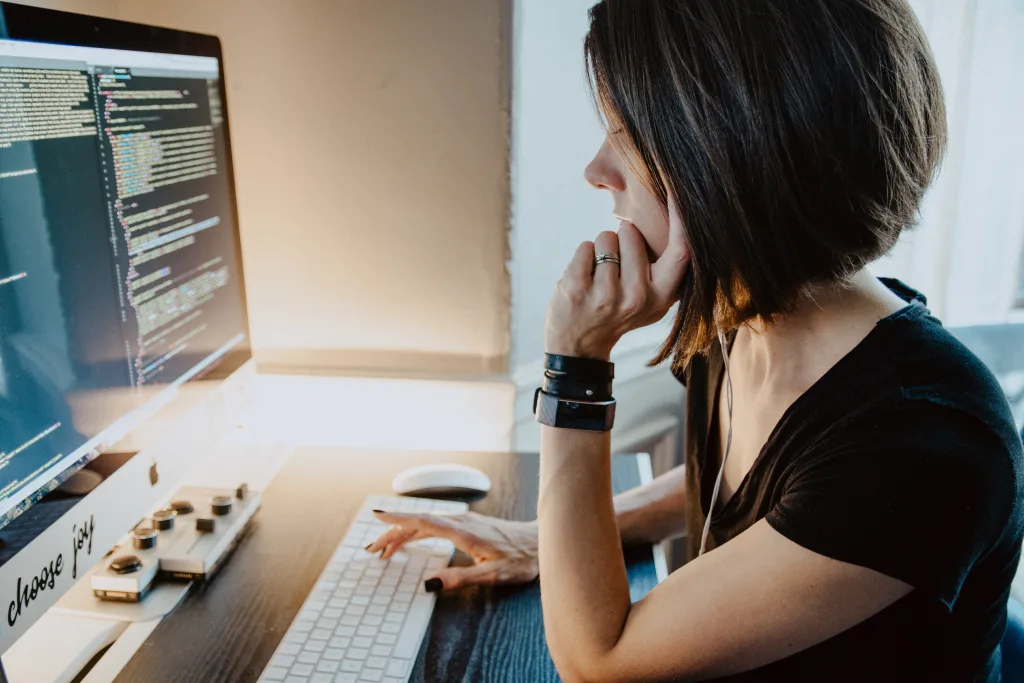
Understanding the Meaning of %d in C Programming
%d is a format specifier used in C programming language. It is used as a placeholder in functions such as printf() and scanf() to indicate that the corresponding argument should be treated as an integer data type. When used with printf(), it displays the corresponding argument as a signed decimal number. When used with scanf(), it reads the value of an integer from the input stream.
When to Use %d and %f in C Programming
When working with C, you should use %d when you need to print an integer as a signed decimal number. This is useful for printing out whole numbers, such as the result of an arithmetic operation. You should use %f when you need to print a floating-point number in normal (fixed-point) notation. This is useful for printing out fractions or decimals, such as the result of a mathematical expression or a calculation involving real numbers.
Are %d and %i the Same?
Yes, the %d and %i format specifiers for printf are exactly the same. Both specify signed decimal integers, and they will produce identical output when used in a printf statement. The only difference between the two is that %d is the traditional format specifier for an integer, while %i is a newer addition to the C language that allows for more flexibility when formatting integers.
Reading Double Values in C
To read a double in C, you need to use the scanf function. The format string used in the scanf function should contain the conversion specification %lf. Additionally, you will need to include a double variable preceded by an ampersand as the second parameter in the scanf function. For example:
double number;
scanf(“%lf”, &number);
The above code would read in a double value that is stored in the variable “number”.
Using Double in C Code
In C, the keyword ‘double’ is used to declare a variable of type double. A double is a data type used to store real numbers (numbers with decimal values). The syntax for declaring a double variable is as follows:
double ;
For example, to declare a double variable named ‘price’, we would use the following statement:
double price;
When declaring a double variable, the memory allocated for it will depend on the target platform’s processor architecture. Generally, 64 bits of memory are allocated for a double value.
Rounding to Two Decimal Places
To round a decimal number to two decimal places, start by identifying the hundredths place, which is the second place to the right of the decimal point. Then, if the third digit after the hundredths place is equal to or greater than five (5), add one (1) to the number in the hundredths place. If it is less than five (5), leave that number as is. For example, 2.83620364 rounds to two decimal places as 2.84, and 0.7035 rounds to two decimal places as 0.70.
Printing Long Double C Values
To print a long double c, we need to use the %Lf format specifier in printf. The syntax for this is as follows:
printf(“Value: %Lf\n”, long_double_variable);
The %Lf format specifier tells the compiler to interpret the argument as a long double and display it accordingly. The \n character after the format specifier causes the output to be printed on a new line.
It is important to note that long double typically has more precision than a regular double. This means that if you are printing a large number or trying to store values with greater accuracy, it is best to use the long double type and its assciated format specifier when printing.
Printing Double Quotes in C
In C, you can print double quotes by using the escape sequence \” (backslash followed by double quote). This escape sequence is used to indicate that the character following it should be treated as a literal character instead of having any special meaning. For example, to print out the text “hello world” in C, you would write: printf(“\”hello world\””);
Printing Floats in C
In order to print a float (a number with decimal places) in C, you can use the printf() statement. The syntax for printing a float is: printf(“The rate of interest for the investment is %f \n”, roi); In this statement, the %f denotes that the value stored in the variable ‘roi’ is of type float and should be printed with 6 digits after the decimal places. For example, if you have declared a variable ‘roi’ as a float and given it a value 10.00, then when you execute this statement it will print 10.000000 as output.
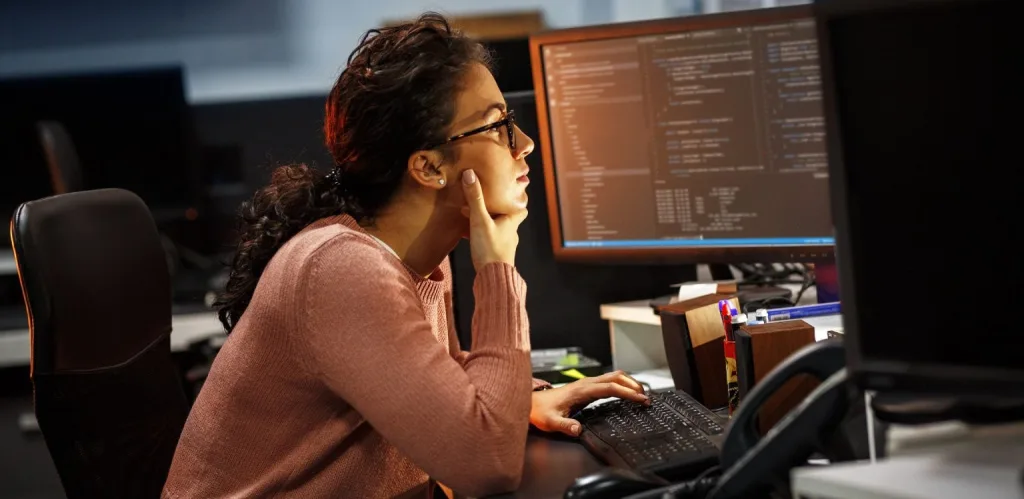
Conclusion
In conclusion, using the c print double function is a great way to accurately and effectively print out double values. It allows you to specify the number of decimal places you want to use, and the output is always consistent. Additionally, it can be used in combination with other formatting specifiers (such as %f) to give you even more control over how your numbers are printed. With its flexibility, accuracy, and ease of use, it is no wonder why the c print double function is such a popular choice for printing out double values.