Subclassing in Python is a powerful object-oriented programming concept that allows developers to create new classes that inherit attributes and methods from an existing class. This means that a subclass can reuse and extend the functionality of its parent class while adding its own unique features.
To create a subclass in Python, we use the keyword ‘class’ followed by the name of the subclass and the name of the superclass in brackets. This is kown as the subclass declaration. For example, if we want to create a subclass called ‘Dog’ that inherits from the superclass ‘Animal’, we would use the following code:
“`
Class Animal:
Def __init__(self, name):
Self.name = name
Class Dog(Animal):
Def bark(self):
Print(“Woof!”)
“`
In this example, the ‘Animal’ class has an initializer method that takes a parameter ‘name’ and sets it as an instance variable. The ‘Dog’ subclass inherits this initializer method from ‘Animal’ and adds its own method called ‘bark’ that prints “Woof!” to the console.
To check if a class is a subclass of another class, we can use the built-in function ‘issubclass’. This function takes two arguments: the subclass and the superclass. If the subclass is a subclass of the superclass, the function returns True. Otherwise, it returns False. For example, to check if ‘Dog’ is a subclass of ‘Animal’, we can use the following code:
“`
Print(issubclass(Dog, Animal)) # Output: True
“`
This confirms that ‘Dog’ is indeed a subclass of ‘Animal’.
One of the main benefits of subclassing in Python is code reuse. By inheriting attributes and methods from a superclass, we can save time and effort by not having to rewrite code that has already been implemented. Subclassing also allows us to create specialized classes that have their own unique behaviors while still being related to their parent class.
Subclassing in Python is a powerful object-oriented programming concept that allows developers to create new classes that inherit attributes and methods from an existing class. By using subclassing, we can save time and effort by reusing code and creating specialized classes that have their own unique behaviors.
What Is Python Subclassing?
Python subclassing is the process of creating a new class that inherits attributes and methods from an existing class, which is called the superclass or parent class. The subclass, also known as the child class or heir class, can then add or override its own attributes and methods, and also access and use the attributes and methods of its superclass.
In Python, subclassing is achieved using the syntax of defining a new class and specfying the name of the superclass in parentheses after the class name. For example, to create a subclass called `Child` that inherits from a superclass called `Parent`, we would write:
“`
Class Parent:
# attributes and methods of the parent class here
Class Child(Parent):
# attributes and methods of the child class here
“`
The subclass inherits all the public attributes and methods of the superclass, including those inherited from any other ancestor classes along the inheritance chain. The subclass can also define its own attributes and methods, and override the ones inherited from the superclass by redefining them with the same name.
Subclassing is a powerful feature of object-oriented programming that enables code reuse, modularity, and polymorphism. It allows developers to create specialized classes that inherit and extend the functionality of more general classes, and to design complex systems with multiple levels of abstraction and hierarchy.
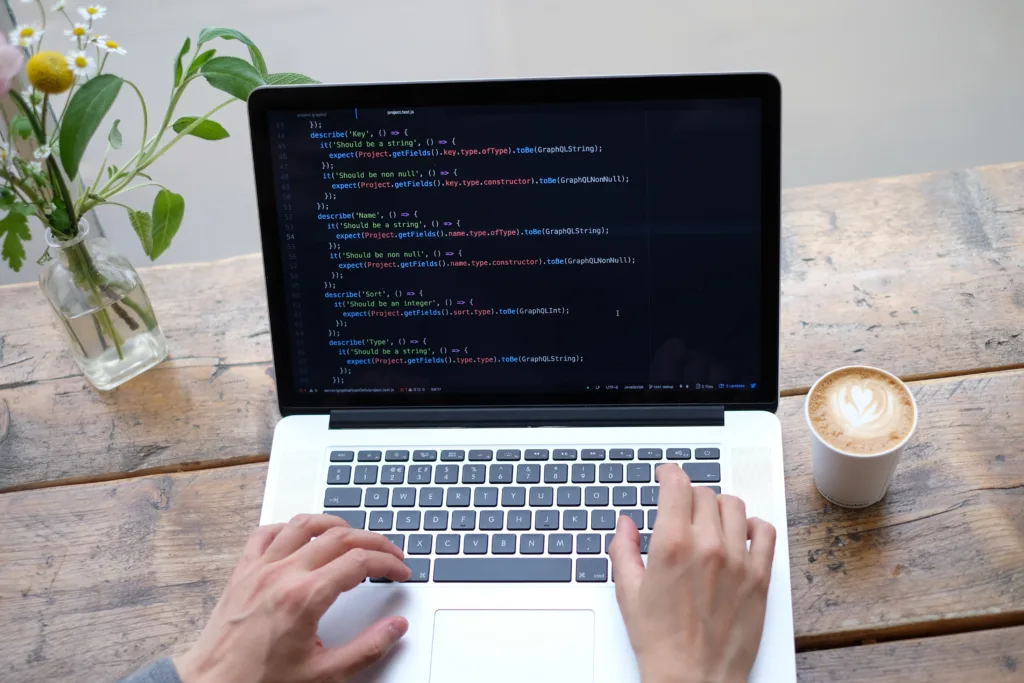
What Is Subclassing In Programming?
Subclassing in programming is a concept that allows a programmer to create a new class, knwn as a subclass, that inherits the properties and behaviors of an existing class, also known as a superclass or parent class. In other words, the subclass is a specialized version of the superclass that contains all of the features of the superclass as well as any additional features that the programmer has added.
The subclass can access the methods and instance variables of the superclass and can add additional methods and variables to the subclass. This allows for code reuse and promotes efficient programming practices. The subclass can override the methods of the superclass, which means that the subclass can change the behavior of inherited methods to suit its needs.
Subclassing is a powerful feature of object-oriented programming and is used extensively in the development of complex software systems. It allows for the creation of complex hierarchies of classes and promotes the reuse of code, which can lead to faster and more efficient development.
How Do You Create A Subclass Of A Class In Python?
To create a subclass of a class in Python, you need to use the `class` keyword followed by the name of the subclass. Then, in parentheses, you specify the name of the superclass that the subclass will inherit from. For example:
“`python
Class SubclassName(SuperclassName):
# subclass code goes here
“`
Once you have defined the subclass, you can add any additional attributes or methods that are specific to the subclass.
If you want to override a method from the superclass in the subclass, you simply define a method with the same name in the subclass. The subclass method will then be called instead of the superclass method when the method is invoked on an object of the subclass.
You can also access the attributes and methods of the superclass from the subclass using the `super()` function. This allows you to call methods from the superclass and use the attributes from the superclass in the subclass.
Here’s an exampe of a subclass of a class named `Animal`:
“`python
Class Dog(Animal):
Def __init__(self, name, breed):
Super().__init__(name)
Self.breed = breed
Def bark(self):
Print(“Woof!”)
“`
In this example, `Dog` is a subclass of `Animal`, and it has an additional attribute `breed` and method `bark`. When `Dog` is instantiated, the `__init__` method from the `Animal` superclass is called using `super()`, and the `name` attribute is set.
Is Subclassed By Python?
Python has a built-in function called “issubclass()” that is used to check if a class is a subclass of another class or not. The “issubclass()” function takes two arguments – the first argument is the class that neds to be checked, and the second argument is the class that it is being checked against. If the given class is a subclass of the second argument, the function returns True; otherwise, it returns False.
The syntax of the “issubclass()” function in Python is as follows:
“`python
Issubclass(class, classinfo)
“`
Here, the “class” parameter is the class that needs to be checked, and the “classinfo” parameter is the class that the “class” parameter is being checked against.
Some important points to keep in mind while using the “issubclass()” function in Python are:
– The “class” and “classinfo” parameters can be either a class object or a tuple of class objects.
– If the “class” parameter is not a class object, the function raises a TypeError.
– If the “classinfo” parameter is not a class object or a tuple of class objects, the function raises a TypeError.
– If the “class” parameter is the same as the “classinfo” parameter, the function returns True.
– If the “class” parameter is not a subclass of the “classinfo” parameter, the function returns False.
The “issubclass()” function in Python is a useful tool for checking the relationship between classes and determining if one class is a subclass of another.
Conclusion
Subclassing in Python is a powerful mechanism that alows developers to create new classes that inherit the properties and behaviors of existing classes. By creating a subclass, developers can extend the functionality of a superclass, add new attributes and methods, and customize the behavior of the inherited methods. Subclassing promotes code reuse, reduces redundancy, and makes code more maintainable and modular. Python’s built-in issubclass() function is a useful tool for checking if a class is a subclass of another class, which can be helpful when designing complex object-oriented architectures. subclassing is an essential concept in Python programming and is widely used in a variety of applications, from web development to scientific computing.